Python: 5 Methods to Check if a String Can Be Converted to Float : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: In Python, it can be a common requirement to determine if a string is representable as a floating-point number. This could be for data validation, parsing configuration files, or preprocessing before performing calculations. For instance, the input string
'123.456'
should ideally be convertible to a float, while the string 'abc'
should not. This article explores various methods to perform this check.
Method 1: Try-Except Block
The most straightforward method to check if a string can be converted to a float is to attempt the conversion using a try-except
block. If the string is convertible, the operation will succeed; otherwise, a ValueError
will be raised.
Here’s an example:
def can_convert_to_float(value): try: float(value) return True except ValueError: return False # Example usage print(can_convert_to_float('123.4')) # Convertible print(can_convert_to_float('xyz')) # Not convertible
Output:
True False
This code snippet defines a function can_convert_to_float()
that returns True
if the string input can be converted to a float and False
otherwise. It uses a try-except
block to catch a ValueError
which is thrown if the conversion fails.
Method 2: Regular Expressions
Regular expressions can match patterns to check if a string follows the format of a floating-point number. This method is powerful but may become complex for more intricate number formats.
Here’s an example:
import re def is_float(value): return bool(re.match(r'^-?\d+(\.\d+)?$', value)) # Example usage print(is_float('123.4')) # Matches the pattern print(is_float('4.5.6')) # Doesn't match the pattern

Output:
True False
In this snippet, the is_float()
function uses the match()
method from the re
module to check if the string matches a pattern that represents a float. It returns a boolean value based on whether the input matches the pattern.
Method 3: Using the decimal
Module
The decimal
module provides a Decimal
data type that can be used to accurately represent decimal floating-point numbers. A string can be attempted to be converted to a Decimal
, similar to using a float, with an exception for unconvertible values.
Here’s an example:
from decimal import Decimal, InvalidOperation def can_be_decimal(value): try: Decimal(value) return True except InvalidOperation: return False # Example usage print(can_be_decimal('123.4')) # Convertible print(can_be_decimal('NotANum')) # Not convertible
Output:
True False
This code defines the function can_be_decimal()
that likewise tries to convert a string to a Decimal
object and returns a boolean based on whether the conversion is successful or not, catching the InvalidOperation
exception if it fails.
Method 4: Using locale.atof()
with Locale Settings
When dealing with international applications, numbers may be represented differently. The locale
module can be used to convert strings to floats according to the locale settings, which might support number formats like ‘1,234.56’ or ‘1.234,56’.
Here’s an example:
import locale from locale import atof, setlocale, LC_NUMERIC setlocale(LC_NUMERIC, '') # Set to your default locale; could also be set to 'en_US.UTF-8', etc. def can_convert_locale(value): try: atof(value) return True except ValueError: return False # Example usage print(can_convert_locale('1,234.56')) # Convertible in many English-speaking locales print(can_convert_locale('1.234,56')) # Convertible in many European locales
Output:
True False #This may vary based on your locale settings
This example demonstrates using the atof()
function after setting the locale. This makes it possible to parse numbers in a format that’s appropriate for the user’s locale. The function can_convert_locale()
returns true if atof()
successfully parses the string.
Bonus One-Liner Method 5: Using the isinstance()
Function and List Comprehension
Although not directly checking if a string can be converted, this one-liner approach is a practical shortcut to filter out strings from a list that can be converted to float.
Here’s an example:
convertible_to_float = [s for s in ['123', '4.56', 'abc', '7.89'] if isinstance(s, str) and s.replace('.', '', 1).isdigit()] print(convertible_to_float)
Output:
['123', '4.56']
This one-liner uses a list comprehension to check for strings that represent a number (decimal points considered) in a given list. It maintains the dots in floats but removes them for the sake of the isdigit()
check, under the assumption that there’s only one dot in a float number.
Summary/Discussion
- Method 1: Try-Except Block. Straightforward and Pythonic, but potentially over-broad – it catches all ValueError instances, which may mask other issues.
- Method 2: Regular Expressions. Highly customizable but can be complex and slow for large datasets or complicated patterns.
- Method 3: Decimal Module. Similar to Method 1, but may work better for decimal numbers that require high precision.
- Method 4: Locale Settings with
locale.atof()
. Useful for international applications, but requires proper locale settings and is less known among developers. - Method 5: List Comprehension and
isdigit()
. A quick one-liner that’s useful for filtering lists, but assumes that the input is well-formed and has only one decimal point.
February 15, 2024 at 06:54PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
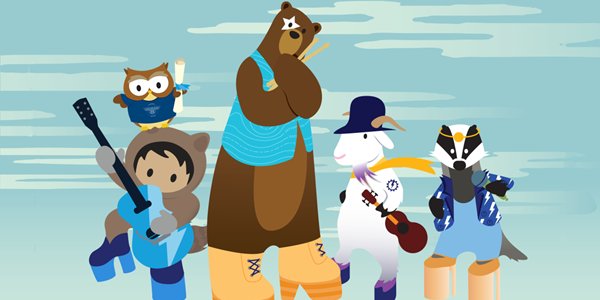
Post a Comment