Pip Install Upgrade: A Concise Guide to Update Your Python Packages : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
To upgrade all packages with pip
, use the command pip list --outdated
to first list all outdated packages, and then pip install --upgrade package-name
for each package to upgrade them individually. There isn’t a built-in pip command to upgrade all packages simultaneously, as this can sometimes lead to dependency conflicts.
Understanding Pip and Upgrade

Pip is a package management system for Python, which allows you to easily install and manage third-party libraries and packages. Upgrade is an option within pip that lets you update an installed package to its latest version.
When you’re working with Python projects, it’s essential to keep your packages up to date to ensure compatibility and take advantage of new features or improvements. To upgrade your packages using pip, you can use the following command:
pip install --upgrade <package>
Replace <package>
with the name of the package you want to upgrade. This command checks the Python Package Index (PyPI) for the latest version of the specified package and updates it accordingly.
Sometimes, it’s necessary to upgrade pip itself. To do this, you can run the following command:
python -m pip install --upgrade pip
This command will check for the latest pip version and update it if a newer version is available. Using python -m
before the pip command ensures that you’re using the correct pip version associated with your Python installation, preventing potential conflicts or issues.
It’s a good idea to periodically check your installed packages for updates and upgrade them as needed. You can list all your installed packages and their versions using this command:
pip list
Installing and Upgrading Pip

To begin with, you should know how to install pip if it’s not already installed in your Python environment. There are two main methods supported by pip’s maintainers: ensurepip
and get-pip.py
. You can use either of these methods to install pip. However, remember that upgrading pip is as important as installing it.
To upgrade pip, you have to use the command line or terminal based on your operating system. Here are the commands you can use in different systems:
- Linux: Open your terminal and type
python -m pip install --upgrade pip
to upgrade pip to its latest version. - MacOS: Similarly, open your terminal and use the command
python -m pip install --upgrade pip
for upgrading pip. - Windows: For Windows, open the command prompt and input
python -m pip install --upgrade pip
.
If you’re using Ubuntu, the usual method to upgrade through the command line won’t work. In this case, you must use the Ubuntu packaging system as follows:
sudo apt update sudo apt install pip
Please note that the Ubuntu package may not always be up-to-date. If you need the latest version, you can download get-pip.py
and run it.
Using the command line or terminal to install and upgrade pip is convenient and ensures that you have the latest version. By following these steps, you can always have an up-to-date pip installation and enjoy the latest features and benefits that come with it.
Pip Operations for Various Platforms
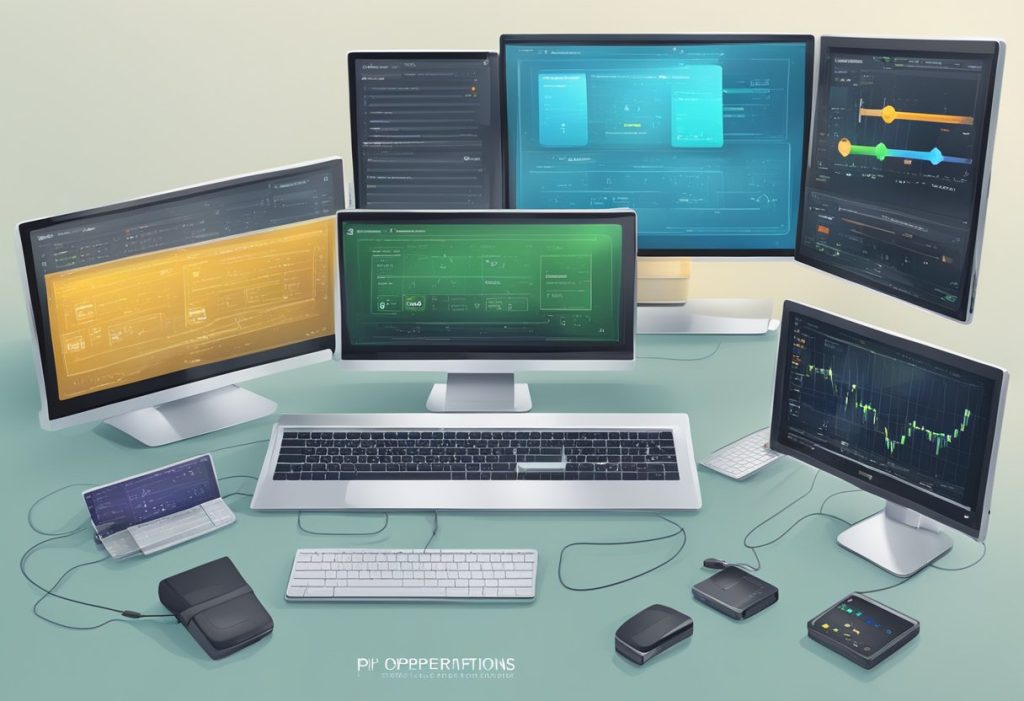
When working with Python packages, Pip is an essential tool that helps you manage their installation and upgrades. Pip operations for various platforms mostly follow the same process, but there are some platform-specific differences that you need to be aware of.
On Windows, MacOS, and Linux, the primary method to upgrade a package is by using the following command:
pip install --upgrade package_name
This command will uninstall the current version of a package and install the latest version available on the Python Package Index (PyPI).
For Ubuntu users, you may occasionally encounter an error during the upgrade process that prevents pip from uninstalling the current version of a package. In such cases, you can use the following command to overcome this issue:
python3 -m pip install --upgrade --user package_name
This command instructs pip to perform the upgrade for the user only, avoiding any global changes to the system Python installation.
When you want to upgrade pip itself, the process is slightly different across platforms:
- On Windows and MacOS, run the following command:
python -m pip install --upgrade pip
- On Linux or Ubuntu, use:
python3 -m pip install --upgrade --user pip
The main difference here is specifying the --user
flag for Linux and Ubuntu to avoid system-level changes.
Handling Python Packages

When it comes to installing packages, you can use the simple command:
pip install <package_name>
This will download the package and its dependencies, and then install them on your system. For instance, if you want to install the popular package numpy
, simply run:
pip install numpy
To list installed packages on your system, use the command:
pip list
This command will display a list of all packages installed on your local Python environment, along with their respective version numbers.
In some cases, you might want to update an existing package to a newer version. To upgrade a package, use the following command:
pip install --upgrade <package_name>
Or use the shorter version:
pip install -U <package_name>
For example, to upgrade numpy
, type:
pip install --upgrade numpy
Exploring User Options
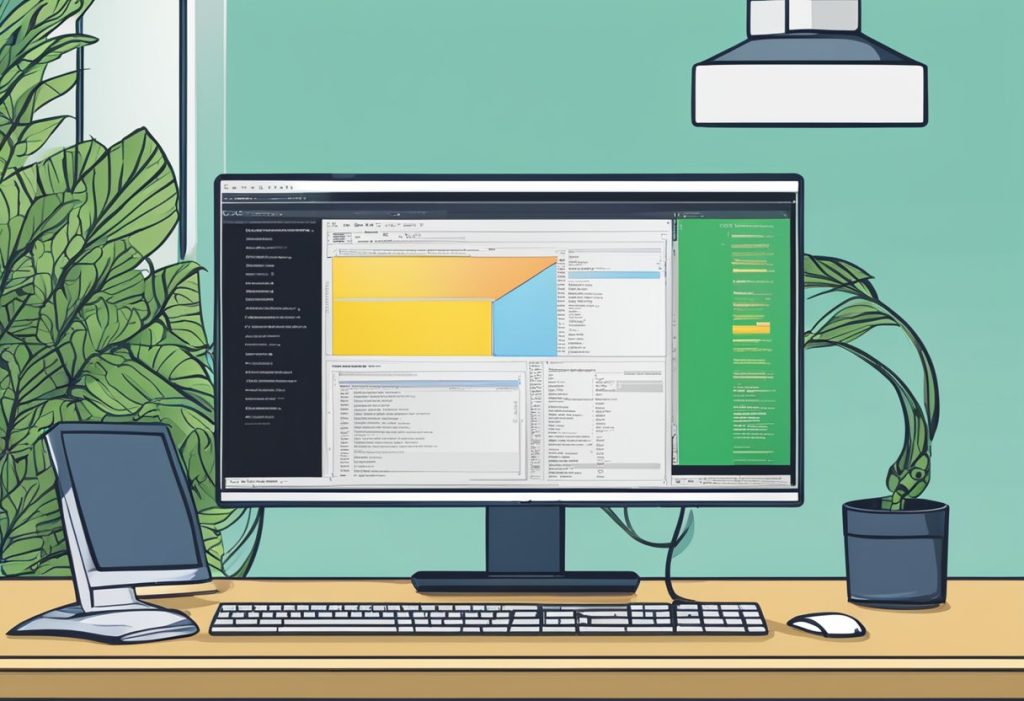
When using pip to manage your Python packages, you have several options for customizing the installation experience. Understanding these user options can help developers like you maintain a clean and organized Python environment.
To upgrade a package using pip, you simply run the command pip install --upgrade <package-name>
. This will look for the latest version of the specified package and update it, ensuring that you have the most up-to-date features and bug fixes. For more information on pip commands, refer to the pip documentation.
If you want to install a package just for the current user, rather than system-wide, you can use the --user
flag. This option is particularly useful on shared machines or servers, where you may not have privileged access. An example of this command would be pip install --user <package-name>
.
When managing Python packages, many developers opt to use virtual environments. These isolated spaces allow you to install and upgrade packages without interfering with the system-wide package installation. To create a virtual environment, you can use either the venv
module for Python 3 or virtualenv
for Python 2. Check out this guide on virtual environments for more details.
Some developers prefer using package managers like Homebrew to keep track of their installed packages. Homebrew is an open-source software package management system, particularly popular among macOS users, that can also handle non-Python packages. You can find further information in the Homebrew documentation.
When using pip, you can interact with subprocesses and execute pip commands programmatically. The subprocess
module in Python allows developers to run, manage, and interact with additional processes. This can be helpful when automating the management and installation of packages. More details about the subprocess
module can be found in the Python documentation.
Lastly, you can customize pip’s behavior by editing its configuration file. The configuration file, typically located in your home directory, allows users to set global options that affect all pip commands. By making changes to the config file, you can tailor your Python package management experience to better suit your needs. More information on pip configuration files can be found in the pip user guide.
Working with Python Versions
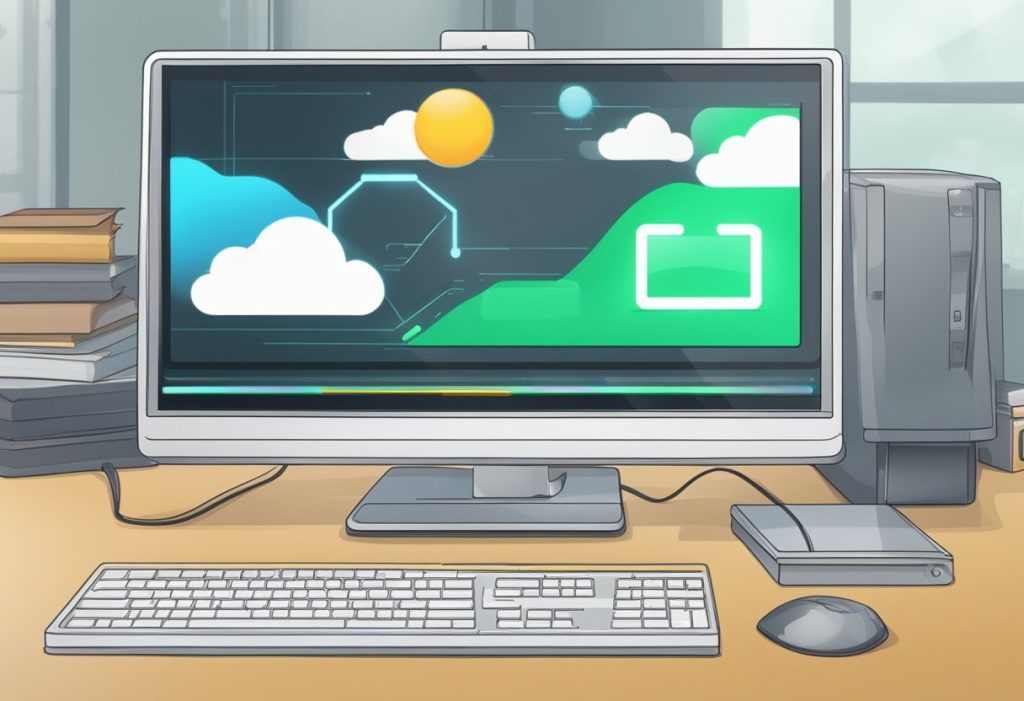
As a Python developer, you might have multiple versions installed, like Python2 and Python3.
First, identify which Python versions are installed in your system by running python --version
and python3 --version
. You’ll receive output that indicates the current version(s) installed. Be aware that python
usually refers to Python2, while python3
refers to Python3.
Now, let’s discuss how to manage packages for these Python versions. You can use pip
for Python2 and pip3
for Python3. Running pip install somepackage
will install the package for the Python version pip is associated with, while pip3 install somepackage
will install it for Python3.
Upgrading your Python packages is essential to keep your projects secure and up to date. Use the command python -m pip install --upgrade somepackage
or python3 -m pip install --upgrade somepackage
to upgrade the desired package for the specific Python version.
Sometimes, you might encounter outdated packages in your installed Python version. To check for outdated packages, use pip list --outdated
(Python2) or pip3 list --outdated
(Python3). Then, you can decide whether to update these packages for better compatibility and stability in your projects.
Don’t forget to use virtual environments when working with multiple Python projects to isolate the dependencies and avoid conflicts.
Understanding Virtual Environments
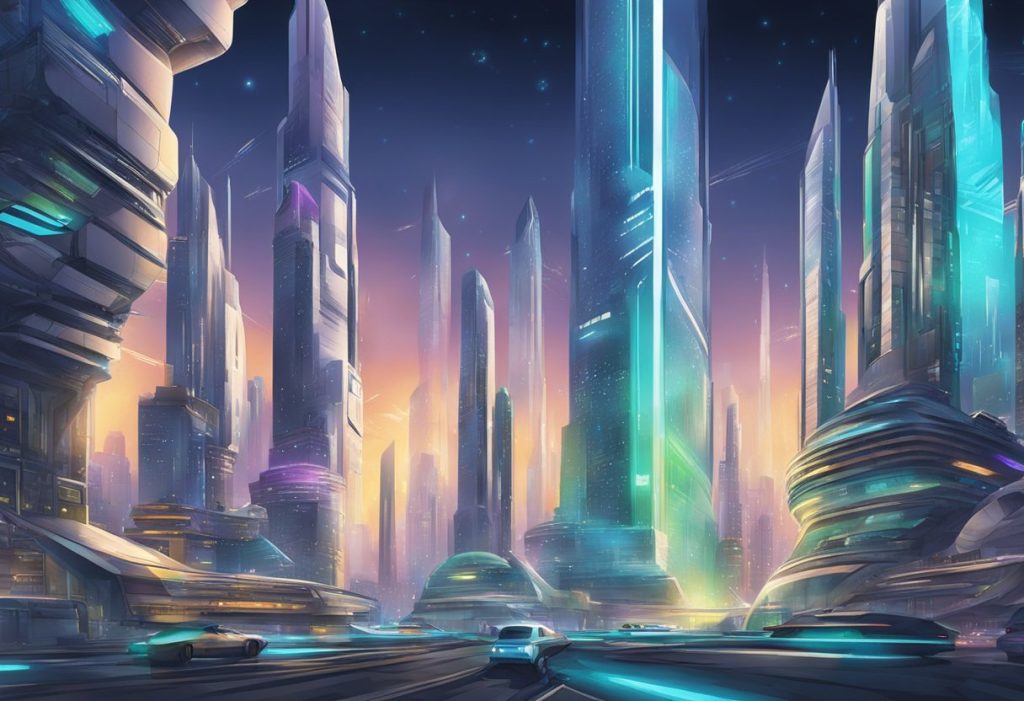
When working with Python, it’s essential to familiarize yourself with virtual environments. A virtual environment is an isolated Python environment where you can install packages and dependencies without affecting your system’s global Python environment. This allows you to maintain separate package installations for different projects, making your work more organized and preventing conflicts between package versions.
There are two main tools for creating and managing virtual environments in Python: venv
and virtualenv
. venv
is a built-in package in Python 3, whereas virtualenv
is a third-party package that supports both Python 2 and 3. To create a virtual environment using venv
, you can use the following command:
python3 -m venv my_project_env
This will create a new folder named my_project_env
that contains the virtual environment. You can think of it as a mini version of your system’s Python environment. To activate the virtual environment, use the appropriate command for your operating system:
- Unix/macOS:
source my_project_env/bin/activate
- Windows:
my_project_env\Scripts\activate.bat
Once activated, you’ll see that your command prompt now has the virtual environment name in it. This indicates that any packages you install using pip
will be installed in the virtual environment and not your system’s global Python environment.
You should always keep your virtual environment’s pip
up to date. Updating pip
ensures your virtual environment is compatible with the latest packages and dependencies. To do this, run the following command:
python -m pip install --upgrade pip
It’s common for developers to work with editable packages, which are Python packages under development that can be installed and updated in a virtual environment without needing to be repackaged and installed each time a change is made. To install an editable package, use the following pip
command:
pip install -e ./path/to/your/package
In summary, virtual environments play a crucial role in Python development. They allow you to manage separate package installations for different projects, keeping your work organized and reducing package conflicts. Making use of tools like venv
or virtualenv
and maintaining an up-to-date pip
installation will help ensure a smooth and efficient development process.
Uninstalling Pip

To uninstall a package in Python using pip, you can use the pip uninstall
command, followed by the package name. This command will remove the specified package from your system. For example, if you want to uninstall a package named “example_package
“, you can run the following command:
pip uninstall example_package
Before actually removing the package, pip will ask for your confirmation. You can also uninstall multiple packages simultaneously by listing their names separated by spaces in the command:
pip uninstall package_name1 package_name2 package_name3
There are some exceptions to packages that pip may not be able to uninstall. Packages installed using python setup.py install
, which do not leave any metadata behind, and script wrappers installed with python setup.py develop
cannot be uninstalled by pip source.
In case you need to uninstall pip itself, follow the specific instructions for your operating system. Generally, removing Python from your system will also remove pip. But if pip was installed separately, refer to the official pip documentation for uninstallation guidelines.
Interacting with PyPI
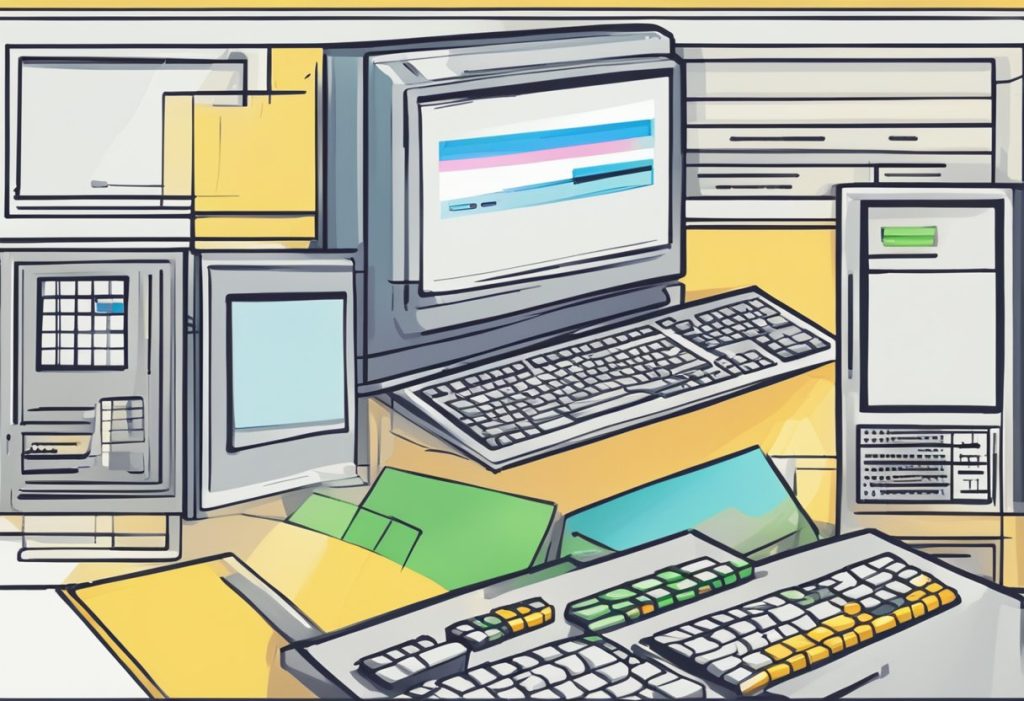
The Python Package Index (PyPI) is a repository of software packages for the Python programming language. PyPI helps you find and install libraries, frameworks, and other modules developed by the Python community. To start interacting with PyPI, you will first need to install pip
.
You can download get-pip.py
from the official Python website and run it to install pip
. Another option is to use the ensurepip
module that comes with Python 3.4 and later versions. Simply run python -m ensurepip --default-pip
to install or upgrade pip
.
Once pip
is installed, you can easily upgrade any specific package with the following command:
python -m pip install --upgrade PACKAGE_NAME
For example, if you want to upgrade the popular data manipulation library pandas
, simply run:
python -m pip install --upgrade pandas
This command will check PyPI for the latest version of the specified package and install or upgrade it accordingly. It’s important to keep your packages up-to-date, as new releases often include bug fixes, security improvements, and new features.
When managing multiple Python projects, using virtual environments is a good idea. This isolates each project’s dependencies, preventing conflicts between different versions of the same package. You can create a new virtual environment by running python -m venv ENV_NAME
and activate it with source ENV_NAME/bin/activate
on Unix/macOS or ENV_NAME\Scripts\activate
on Windows.
Managing Pip with Git and Cloud
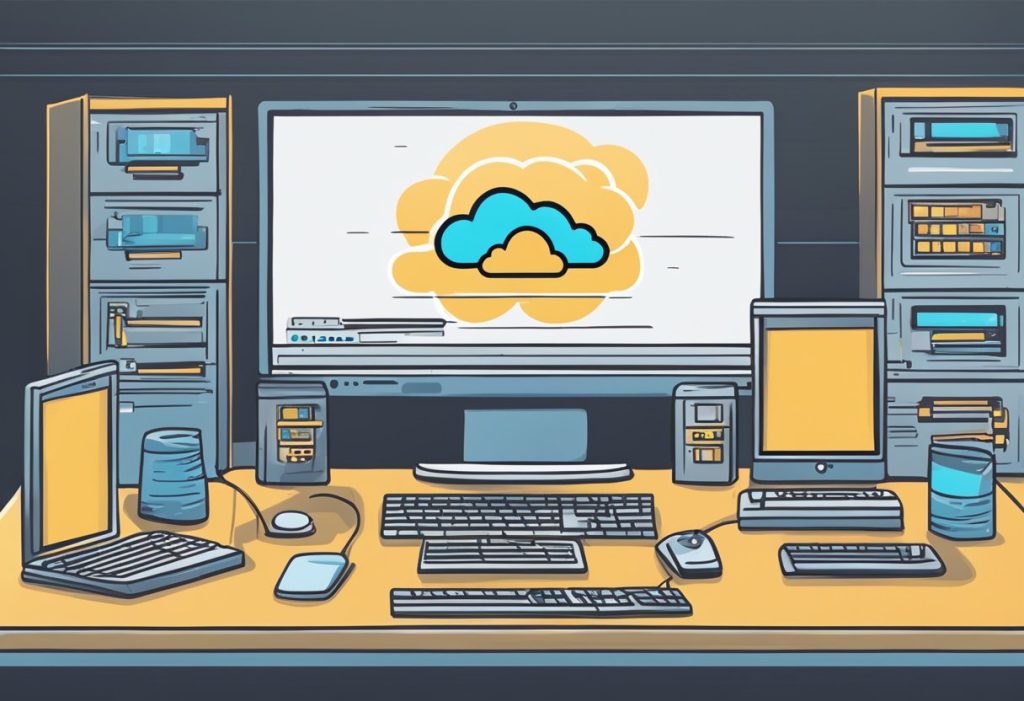
Using pip in combination with Git and cloud-based services can greatly enhance your package management experience. By harnessing the power of Git and the cloud, you can maintain better control over your Python project dependencies.
One way to leverage Git is by installing Python packages directly from a Git repository using pip. This is particularly useful when you need to install a package from a specific branch or commit, which might not be available in the Python Package Index (PyPI).
To install a package from a Git repository, you use the command:
pip install git+https://github.com/username/repo.git@branch_name
Replace username
, repo.git
, and branch_name
with the appropriate values for the package you want to install.
When it comes to cloud-based services, platforms like ActiveState offer streamlined workflows for building and deploying Python projects. By using the ActiveState platform, you can automate the process of creating Python builds, thereby reducing the risk of security vulnerabilities.
Cloud-based platforms can also provide additional benefits such as dependency management, version control, and collaboration tools. This simplifies the process of working with your project dependencies across different environments and team members. It also helps ensure that your project runs consistently across various machines.
Distribution Files and Wheels
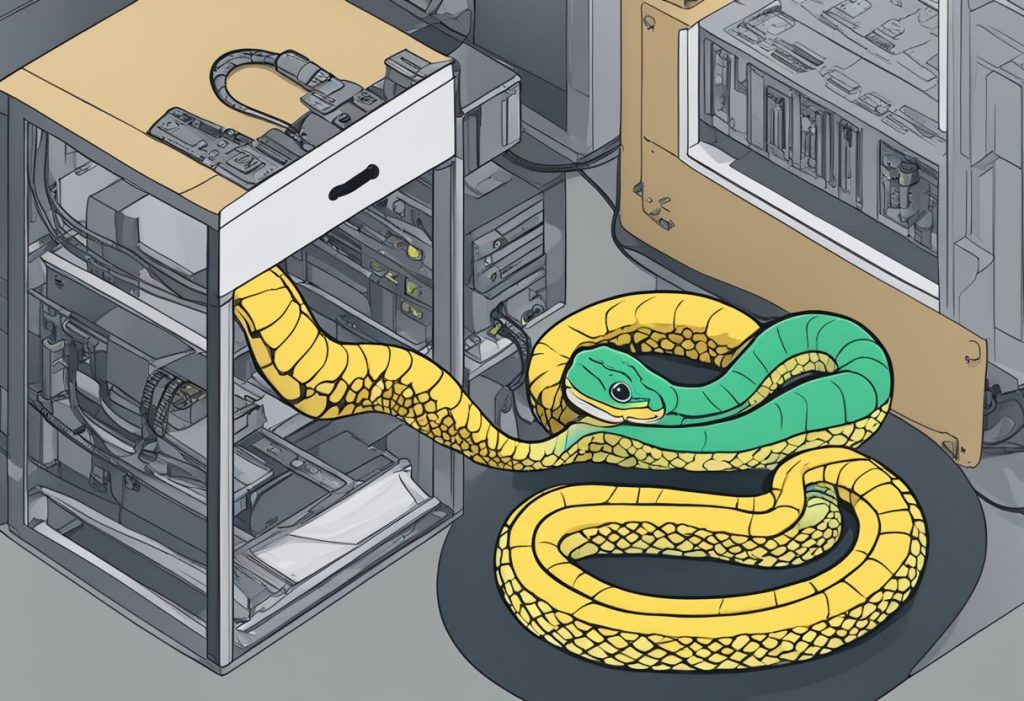
A distribution file is a package that contains all the necessary files and metadata to install a Python library or module. These files can come in various formats like source archives (tar.gz, zip) or binary distributions known as wheels (.whl).
Wheels are a more modern and efficient format for Python packages, designed to make installations faster and easier. They are binary distributions, containing compiled code, which means you don’t need to build anything from source during the installation process. This can save you time, especially when installing packages with complex dependencies or large codebases.
To use wheels, you’ll need pip
, the Python package manager. It automatically handles the installation of both wheels and distribution files. When you run pip install <package_name>
, it will search for a compatible wheel to download and install. If no compatible wheel is found, it will fall back on downloading and compiling the source distribution. This ensures that you always get the best available option for your system.
To upgrade a package using pip
, simply include the --upgrade
flag, like this: pip install --upgrade <package_name>
. This command will check for a newer version of the package, and if available, download and install it. If you want to install a specific version of a package, you can specify it using the ==
operator followed by the version number, like this: pip install <package_name>==<version>
.
Remember that when working with Python packages, it is recommended to use virtual environments, as they help to manage dependencies and create isolated workspaces for your projects. This ensures that your package installations don’t interfere with each other or the system-wide Python environment.
Constraints and Force Reinstalls
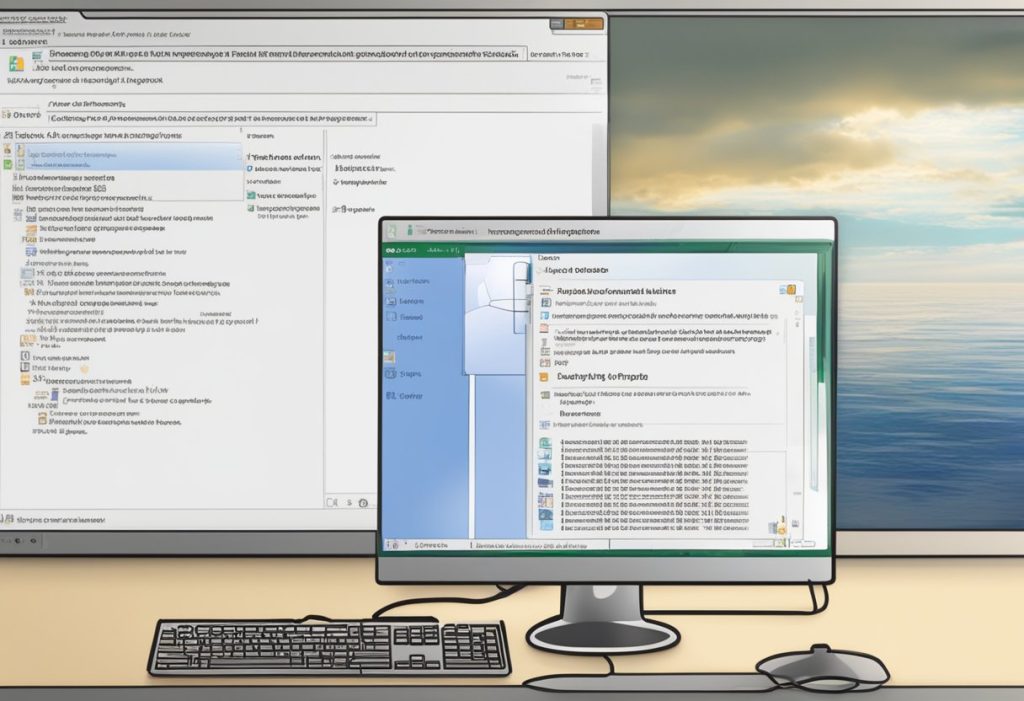
When managing packages in your Python projects, you might sometimes face scenarios where you need to reinstall a package, ignore an installed package, or apply constraints during an upgrade. In such cases, pip provides a few helpful options to cater to your needs.
To force a reinstall of a package, even if it is already installed or up-to-date, you can use the --force-reinstall
option, like so:
pip install --upgrade --force-reinstall <package>
This command ensures that the package gets reinstalled, regardless of its current status.
For cases where you would like to ignore already installed packages and reinstall them as part of the current operation, you can use the --ignore-installed
option:
pip install --ignore-installed <package>
This command helps you ensure that the package is installed anew, without considering existing installations.
Additionally, when upgrading packages, you may want to apply constraints to maintain specific versions or a range of versions to be installed. To achieve this, use the -c
option followed by the path to your constraint file:
pip install --upgrade -c <path/to/constraint/file> <package>
The constraint file is a plain text file containing package names with their desired version specifications. An example constraint file might look like:
some-package==1.2.3 another-package>=2.0.0,<=3.0.0
Frequently Asked Questions

How do I upgrade a specific package using pip?
To upgrade a specific package using pip, you can run the following command in your terminal or command prompt:
pip install --upgrade package-name
Replace package-name
with the name of the package you want to upgrade.
How do I install a specific version of a package with pip?
To install a specific version of a package using pip, use the following command:
pip install package-name==version-number
Replace package-name
with the name of the package, and version-number
with the desired version number of the package.
How can I install pip for Python on Windows?
For installing pip on Windows, first, you need to have Python installed. After that, you can download the get-pip.py script and then run it on the command prompt as follows:
python get-pip.py
This command installs pip for Python on Windows.
What is the command to check the pip version?
To check the pip version installed on your system, open the terminal or command prompt and run the following command:
pip --version
This command will display the currently installed pip version.
How do I upgrade all installed packages using pip?
To upgrade all the installed packages using pip, you can use the following command:
pip list --outdated --format=freeze | grep -v '^\-e' | cut -d = -f 1 | xargs -n1 pip install -U
This command lists all outdated packages, filters them, and then upgrade each package one by one.
Can I upgrade Python itself with pip on Windows?
No, you cannot upgrade Python itself with pip on Windows. To upgrade Python on Windows, visit the Python official website and download the latest version. Install the downloaded package to upgrade your Python version.
November 15, 2023 at 01:09AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
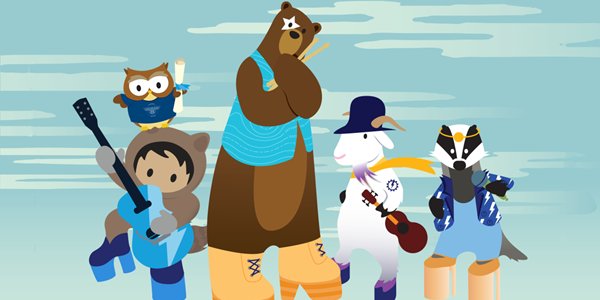
Post a Comment