SyntaxError: (Unicode Error) unicodeescape Codec Issue Fixing Truncated Position 2-3 Escape : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
When working with Python, you might encounter the “SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
” error.
This error occurs when Python attempts to interpret a file path that contains incorrect formatting. Specifically, Python identifies single backslashes as escape characters, rather than path separators, which is a common mistake for developers working on Windows systems.

Recommended:
(unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
In this article, we will explore solutions to fix this syntax error and prevent it from occurring in the future.
Root cause of this problem
Inaccurate path formatting typically results from the use of single backslashes in file paths, especially when dealing with Windows paths. Since single backslashes are escape characters in Python, they can lead to confusion when interpreting the file paths.
Let’s dive deeper into the error next:
Understanding The Error
SyntaxError and Unicode Error
The SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
is a common error that occurs when working with strings containing backslashes in Python. This error is caused by a combination of two issues: a SyntaxError
, which occurs when your code has incorrect syntax; and a UnicodeError
, an encoding/decoding error that arises when dealing with Unicode characters.
Unicode Escape Sequence
In Python, the backslash \
is used as an escape character to represent various special characters. When dealing with Unicode characters, you can use the escape sequence \u
or \U
followed by a specific set of hexadecimal digits to represent a Unicode character.
For example, \u00A9
represents the copyright symbol ©.
However, when a backslash is placed before regular characters (like in file paths), it can lead to an erroneous interpretation of those characters as Unicode escape sequences, causing the unicodeescape
error.
Truncated Escape
The “truncated escape” portion of the error refers to an incomplete or incorrect Unicode escape sequence.
In this case, Python tries to interpret the character(s) following the backslash as a Unicode escape sequence, but fails to do so because the sequence is either too short or contains invalid characters. This triggers the SyntaxError
and UnicodeError
, indicating that there’s an issue with your code when dealing with Unicode escape sequences.
To avoid this error, you can use one of the following solutions:
- Replace backslashes with forward slashes in your file paths (e.g.,
'C:/Users/username/Desktop/example.csv'
). - Use double backslashes (e.g.,
'C:\Users\username\Desktop\example.csv'
). - Use raw strings by adding an
'r'
before the string (e.g.,r'C:\Users\username\Desktop\example.csv'
).
Recommended: Python Raw Strings: A Helpful Easy Guide
Python and Strings
In Python, strings are an essential data type often used for representing texts and storing information. This section will discuss escape characters, sequences, and how to use raw strings and string literals.
Escape Characters and Sequences
An escape character in Python is a backslash \
followed by a special character, known as an escape sequence. Escape sequences represent specific control characters, such as newline '\n'
or tab '\t'
. They are used to embed special characters into a string without causing syntax errors or misinterpretation.
For example:
example_string = "This is a string with a newline\ncode." print(example_string)
Output:
This is a string with a newline code.
When using single quotes '
or double quotes "
within a string, escape characters can be helpful to avoid syntax errors. To include single quotes within a single-quoted string or double quotes within a double-quoted string, use the escape character:
single_quote_example = 'It\'s a beautiful day!' double_quote_example = "The teacher said, \"Study hard!\""
Raw Strings and String Literals
In some cases, you may need to use a string with multiple backslashes or escape sequences, such as representing file paths in Windows. For this, you can use raw string literals in Python. To define a raw string, you can prefix it with the r
character. A raw string treats backslashes as regular characters and does not interpret escape sequences:
raw_string = r"This is a raw string with\no newline." print(raw_string)
Output:
This is a raw string with\no newline.
Raw string literals are especially useful when working with unicode code points in strings, since Python may interpret the characters after the backslash as escape sequences and raise a SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \UXXXXXXXX escape
. For example, you can use raw string literals for Windows file paths:
file_path = r"C:\Users\username\Documents\example.txt"
By using escape characters, sequences, and raw string literals, you can confidently work with strings in Python and avoid common errors associated with special characters.
Handling File Paths
When working with file paths in Python, it’s important to consider the differences in how paths are formatted on various operating systems. This can sometimes lead to the SyntaxError: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \uxxxxxxxx escape
. To handle file paths correctly, let’s explore several approaches for different systems.
Windows and Backslashes
In Windows systems, the backslash (\
) is used as a path separator. However, the backslash character in Python can also be a special character that creates escape sequences. This can cause issues when dealing with file paths.
For example, consider this path: C:\Users\yourname\Desktop\example.txt
. To avoid issues with the backslash character, there are a few methods to handle file paths in Windows.
Forward Slashes
One easy way to prevent issues with backslash escape sequences is to use forward slashes instead (“/
“). Python can interpret forward slashes correctly on both Windows and other systems.
Here’s how the previous path would look using forward slashes:
file_path = 'C:/Users/yourname/Desktop/example.txt'
This approach can help you avoid the (unicode error) 'unicodeescape'
issue .
Double Backslashes
Another way to handle file paths in Windows is by using double backslashes (“\
“). This effectively “escapes” the special character and allows Python to interpret the path correctly.
Here’s the example path using double backslashes:
file_path = 'C:\\Users\\yourname\\Desktop\\example.txt'
Using either forward slashes or double backslashes can help you prevent issues with file paths in Python, ensuring a more seamless experience across different systems. Remember to consider the specific needs of your project and choose the method that works best for your situation.
Dealing with CSV Files
Importing CSV Files
When working with CSV files in Python, importing the csv
module is essential. This module provides two important methods: csv.reader
and csv.writer
. They simplify reading and writing CSV files, offering ways to handle newline characters and delimiter options.
import csv with open('example.csv', 'r') as file: csv_reader = csv.reader(file) for row in csv_reader: print(row)
Pandas and CSV Files
Pandas, a fantastic library for data manipulation, also provides a useful method for reading and writing CSV files: pd.read_csv()
. This method can handle complex cases such as different delimiters, escape sequences, and even take care of memory management while loading large CSV files.
import pandas as pd df = pd.read_csv('example.csv')
Fixing Common Errors
When working with CSV files, some common errors may arise, such as syntaxerror: (unicode error) 'unicodeescape' codec can't decode bytes in position 2-3: truncated \uxxxxxxxx escape
. This error is often due to an incorrect use of backslashes in file paths, as they may be interpreted as escape sequences rather than simple path separators.
To fix this error, you can:
- Replace backslashes with forward slashes in file paths:
df = pd.read_csv('C:/Users/example/Desktop/example.csv')
- Use a raw string in the file path, by adding an
r
prefix:
with open(r'C:\Users\example\Desktop\example.csv', 'r') as file:
- Make sure to use the correct encoding for reading the file, such as UTF-8:
df = pd.read_csv('example.csv', encoding='utf-8')
Solutions and Workarounds
Raw String Prefix
Using a raw string prefix can help solve this error involving the file path and Unicode escape characters . By simply adding an
r
before the file path string, Python will treat the string as a raw string and ignore any escape characters present, like backslashes.
For example:
file_path = r"C:\Users\your_username\Desktop\data.csv"
By using this method, you can avoid issues with the decode bytes
in the file path that may otherwise throw a SyntaxError
.
Triple-Quoted Strings
Another solution is using triple-quoted strings to avoid unicodeescape
errors. By using triple quotes, you can create multi-line strings and include escape characters without any issues. This can be particularly helpful when importing pandas data from a file path.
Here’s an example:
file_path = """C:\\Users\\your_username\\Desktop\\data.csv"""
This approach allows you to use escape characters without causing an error message
. Triple-quoted strings can work with single or double quotes, and they will make the bot’s task of processing the data much more manageable.
Using Both Single and Double Quotes
A third workaround consists of using a combination of single and double quotes in your file path strings. This method can help you to deal with escape characters without the need for a raw string or triple-quoted strings.
For example:
file_path = "C:\\Users\\your_username\\Desktop\\'data.csv'"
When processing text, some authors prefer this approach due to its simplicity. Although it might not be as popular as using the raw character r
or creating triple-quoted strings, this method is an effective solution to handle escape characters and import pandas data.
Frequently Asked Questions
How to fix UnicodeEscape error in Python?
The UnicodeEscape error often occurs when Python interprets backslashes as escape characters in file paths. To fix the issue, consider using double backslashes \\
or raw strings by prefixing your string with r
. For example, change 'C:\Users\example.txt'
to 'C:\\Users\\example.txt'
or r'C:\Users\example.txt'
. Alternatively, you may use forward slashes /
instead of backslashes \
in your file path.
What causes FileNotFoundError: [Errno 2] No such file or directory?
This error occurs when Python cannot find the specified file or directory in the given path. Make sure that the file path is correct and the file exists. Check for typos and ensure the correct case is used, particularly on case-sensitive file systems. If your file is located in another directory, adjust the path accordingly, or use absolute paths instead of relative paths.
How to resolve UnicodeDecodeError: ‘utf-8’ codec can’t decode byte?
This error signifies that Python encountered non-UTF-8 encoded data when trying to decode a file or a text stream. To resolve the issue, determine the correct encoding of the data and specify it as a parameter when opening the file. For instance, if your file is encoded in ISO-8859-1, use open('file.txt', 'r', encoding='ISO-8859-1')
to read the file correctly.
Why am I encountering Unicode error in Python?
Unicode errors in Python are typically due to incorrect handling of text data. Common causes include mismatched encodings, incorrect file paths with special characters, or operations between Unicode strings and byte sequences. To prevent these errors, pay close attention to encodings and use escape sequences or raw strings for file paths with special characters.
How to handle PermissionError: [Errno 13] Permission denied?
This error occurs when you attempt to read, write, or execute a file without the necessary permissions. To fix this, ensure that your Python script has the required permissions to access the file. You can modify permissions using the os.chmod()
function, or by manually adjusting the file’s properties on your operating system. Additionally, check if your script is running with the correct user privileges, as elevated permissions might be needed in certain scenarios.
How to fix UnicodeDecodeError: ‘utf-8’ codec can’t decode bytes in position 15-16: invalid continuation byte?
This error occurs when Python encounters an improperly formatted UTF-8 byte sequence. To fix this, try specifying the correct encoding using the encoding
parameter when opening the file or decoding the text. If you’re unsure of the correct encoding, you may use the chardet
library to automatically detect the encoding before decoding. Keep in mind that not all byte sequences are valid text, so make sure the data you’re dealing with is indeed text data.
Recommended: 26 Freelance Developer Tips to Double, Triple, Even Quadruple Your Income
June 25, 2023 at 05:04PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
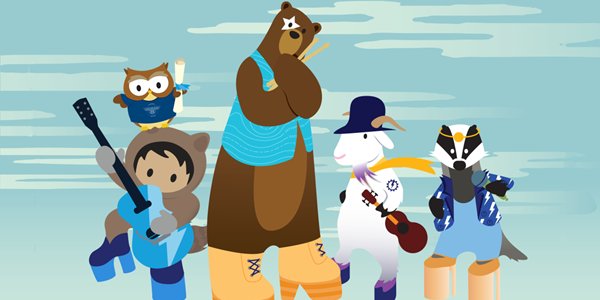
Post a Comment