Python One Line HTTP Server: An Easy Quickstart for Beginners : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
TLDR: To quickly launch an HTTP server using Python, open your terminal, navigate to the directory you want to serve, and execute the command
python3 -m http.server
which will start serving files over HTTP on port 8000 accessible through the default network interface.
If you need to specify a different port or bind to a specific interface, you can augment the command as python3 -m http.server 8080 --bind 127.0.0.1
, which uses port 8080 and restricts access to your local machine only.
This method is ideal for development, testing, or sharing files within a local network but is not secure enough for production environments.
Setting up a web server to test applications or share files can sometimes feel like an uphill climb. Traditional web servers like Apache or NGINX are powerful but not always nimble or necessary for smaller, more immediate tasks. This is where Python’s built-in HTTP server module, http.server
, offers a simple yet effective solution.
How to Launch Python’s HTTP server with One Line of Code
Built into the Python Standard Library is a little gem called http.server
. It doesn’t require installation of third-party packages or extensive configuration, making it incredibly useful for serving files, experimenting with HTTP requests, or teaching networking concepts.
Starting the Server
To run http.server
, you need Python installed on your machine. This is likely already the case, but if not, you can download Python from python.org.

Once Python is installed, launching the server is straightforward. Open your command line tool (Terminal for macOS and Linux, Command Prompt or PowerShell for Windows), navigate to the directory you want to serve files from, and enter:
python3 -m http.server
This command starts a web server on port 8000. By default, the server binds to all available network interfaces with IP address 0.0.0.0
for IPv4 and ::
for IPv6. This setting allows any device on the same network to access the server using your machine’s IP address.
To access the server, type http://0.0.0.0:8000/
in a web browser on your or any connected device. Replace 0.0.0.0
with your actual server IP address if necessary.
As I ran this in my main directory, it now simply starts serving directories on my computer:

Note that as you visit the localhost:8000
in your browser, the command line gives you information about access patterns like so:

Specifying a Port
If port 8000 is in use or you prefer to use another port:
python3 -m http.server 8080
This command launches the server on port 8080 instead.
Binding to a Specific Interface
For added security, especially when working in shared environments, you can bind the server to localhost
, making it accessible only on your local machine:
python3 -m http.server --bind 127.0.0.1 8000
This command restricts access to your personal device, preventing other network users from accessing your server.
Important Considerations
Security: The simplicity of Python’s HTTP server comes with limitations. It is not designed for production usage. It lacks many security features, making it vulnerable to numerous forms of attack if exposed to the public internet.
Performance: The server is not optimized for high traffic or heavy load. It serves content on a single thread, handling one request at a time, which can lead to slow responses under concurrent access.
Practical Uses

Python’s built-in HTTP server, accessed via the http.server
module, serves as an ideal tool for several practical applications suited to non-production environments.
For instance, during educational workshops or classroom settings, instructors can swiftly set up a server to distribute course materials or code examples to students, ensuring everyone works with identical content without the need for USB drives or unreliable email attachments.
Web developers find it particularly useful for quick tests of website prototypes, previewing changes in real-time across different devices on the same network, such as tablets, smartphones, and laptops.
Moreover, it can be used by teams in a corporate setting to temporarily share project files or documentation within an internal network, enhancing collaboration without compromising sensitive data by using external cloud storage services.
Specifically, despite its limitations, http.server
is incredibly useful in specific contexts:
- Development and Testing: Quick test of HTML pages or other web resources during development.
- Education and Workshops: Teaching web technology concepts in environments where installing software is restricted.
- Simple File Sharing: Temporarily sharing files within a local network.
Encrypting Your Connection with HTTPS
While the module does not natively support HTTPS, you can wrap the socket in SSL to secure your transmissions, using a self-signed certificate for testing:
import http.server import ssl server_address = ('0.0.0.0', 4443) httpd = http.server.HTTPServer(server_address, http.server.SimpleHTTPRequestHandler) httpd.socket = ssl.wrap_socket(httpd.socket, keyfile="path/to/key.pem", certfile='path/to/cert.pem', server_side=True) httpd.serve_forever()
This snippet starts a secure server using SSL on port 4443 (ports below 1024 like 443 require root access).
Python One-Liner Webserver HTTP
April 19, 2024 at 08:54PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
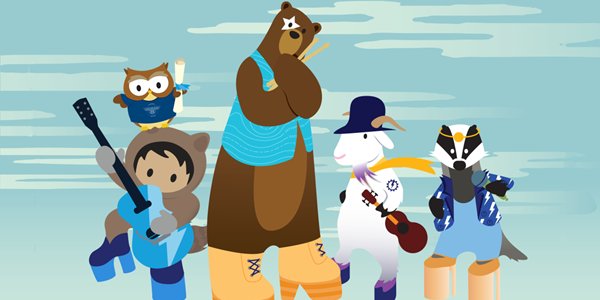
Post a Comment