Python Enum Conversion (Ultimate Guide) : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Enums (short for enumerations) are a powerful feature in Python that allows for organizing a set of symbolic names (members) bound to unique, constant values. Enums are a convenient way to associate names with constants in a clear and explicit way.
Before diving into the conversion methods, let’s quickly recap the Python enum
module. In this example, Color
is an enumeration with three members: RED
, GREEN
, and BLUE
, associated with the values 1, 2, and 3, respectively.
MINIMAL ENUM EXAMPLE:
from enum import Enum # Define an enum named Color with a few members class Color(Enum): RED = 1 GREEN = 2 BLUE = 3 # Accessing members and their values Color.RED # <Color.RED: 1> Color.RED.name # 'RED' Color.RED.value # 1
But how do we convert these enums to more common data types and back? Let’s start with the first:
Python Convert Enum to String – and Back
Converting an Enum to a string can be particularly useful for display purposes or when the enumeration needs to be serialized into a format that requires text (like JSON). The reverse process is handy when parsing strings to obtain Enum values.
from enum import Enum class Color(Enum): RED = 1 GREEN = 2 BLUE = 3 # Convert Enum to String color_string = Color.RED.name # Convert String to Enum color_enum = Color[color_string] print(color_string) # Outputs: RED print(color_enum) # Outputs: Color.RED
Here, Color.RED.name
is used to convert an Enum member to a string. On the flip side, Color[color_string]
converts a string back to an Enum member. This is particularly helpful in configurations or environments where only strings are usable.
Python Convert Enum to Int – and Back
Sometimes, you might need to convert Enum members to integers for indexing, interfacing with C code, or simple arithmetic. Conversely, converting integers back to Enums is vital for maintaining the encapsulation of Enum types.
# Convert Enum to Int color_int = Color.RED.value # Convert Int to Enum color_enum_from_int = Color(color_int) print(color_int) # Outputs: 1 print(color_enum_from_int) # Outputs: Color.RED
In this snippet, Color.RED.value
gives the integer associated with Color.RED
. To convert this integer back to an Enum, we call Color(color_int)
.
Python Convert Enum to List – and Back
Converting an Enum to a list is useful when you need to perform operations that require sequence data types like iterations, mapping, etc. The reversal doesn’t apply typically as you wouldn’t convert a list directly back into an Enum, but you could recreate the Enum if needed.
# Convert Enum to List color_list = [c for c in Color] print(color_list) # Outputs: [<Color.RED: 1>, <Color.GREEN: 2>, <Color.BLUE: 3>]
This list comprehension iterates over the Enum class, converting it into a list of Enum members.
List Comprehension in Python — A Helpful Illustrated Guide
Python Convert Enum to Dict – and Back
When dealing with Enum members in a dynamic manner, converting it to a dictionary can be incredibly useful, especially when the names and values are needed directly.
# Convert Enum to Dict color_dict = {color.name: color.value for color in Color} # Convert Dict to Enum class ColorNew(Enum): pass for name, value in color_dict.items(): setattr(ColorNew, name, value) print(color_dict) # Outputs: {'RED': 1, 'GREEN': 2, 'BLUE': 3} print(ColorNew.RED) # Outputs: ColorNew.RED
Here, we first create a dictionary from the Enum, ensuring each name maps to its respective value. To convert it back, we dynamically generate a new Enum class and assign values.
Python Convert Enum to JSON – and Back
Serialization of Enums into JSON is essential for configurations and network communications where lightweight data interchange formats are used.
import json # Convert Enum to JSON color_json = json.dumps(color_dict) # Convert JSON to Enum color_info = json.loads(color_json) color_enum_from_json = Color[color_info['RED']] print(color_json) # Outputs: '{"RED": 1, "GREEN": 2, "BLUE": 3}' print(color_enum_from_json) # Outputs: Color.RED
This example shows how you can use a dictionary representation to convert Enum members into JSON and then parse it back.
Python Convert Enum to List of Strings – and Back
In some cases, a list of string representations of Enum members may be required, such as for populating dropdowns in GUI frameworks, or for data validation.
# Convert Enum to List of Strings color_str_list = [color.name for color in Color] # Convert List of Strings to Enum color_enum_list = [Color[color] for color in color_str_list] print(color_str_list) # Outputs: ['RED', 'GREEN', 'BLUE'] print(color_enum_list) # Outputs: [<Color.RED: 1>, <Color.GREEN: 2>, <Color.BLUE: 3>]
This straightforward comprehension allows for quick conversions to and from lists of string representations.
Python Convert Enum to Tuple – and Back
Tuples, being immutable, serve as a good data structure to hold Enum members when modifications are not required.
# Convert Enum to Tuple color_tuple = tuple(Color) # This showcases conversion but typically you won't convert back directly # However, Enum members can be extracted back from the tuple if needed print(color_tuple) # Outputs: (Color.RED, Color.GREEN, Color.BLUE)
While the reverse is uncommon, extracting specific Enum members from a tuple is straightforward.
Python Convert Enum to Literal – and Back
Python type hints support literals which can be handy in situations where function parameters are expected to be specific Enum members only.
from typing import Literal ColorLiteral = Literal[Color.RED.name, Color.GREEN.name, Color.BLUE.name] # Example function using this literal def set_background_color(color: ColorLiteral): print(f"Setting background color to {color}") set_background_color('RED') # Correct usage # Conversion back is essentially parsing the literal to the Enum parsed_color = Color['RED'] # Works as expected print(parsed_color) # Outputs: Color.RED
Here, Literal
is a way to ensure type-checking for functions that should only accept specific Enum members in string form.
Python Convert Enum to Map – and Back
The map
function in Python can apply a function over a sequence and is easily demonstrated with Enums.
# Use map to convert Enum to another form mapped_values = list(map(lambda c: (c.name, c.value), Color)) # Normally you won't convert a map result back to Enum directly # But you can reconstruct the Enum if needed print(mapped_values) # Outputs: [('RED', 1), ('GREEN', 2), ('BLUE', 3)]
Mapping Enum values to a structure like tuple pairs can facilitate operations such as sorting by either names or values.
Python Convert Enum to List of Tuples – and Back
This conversion is useful for creating indexed structures from Enums, beneficial in scenarios where a tuple-based lookup is needed.
# Convert Enum to List of Tuples color_tuples_list = [(color.name, color.value) for color in Color] # This can directly be used to reconstruct the Enum if needed class ReconstructedEnum(Enum): RED = 1 GREEN = 2 BLUE = 3 print(color_tuples_list) # Outputs: [('RED', 1), ('GREEN', 2), ('BLUE', 3)] print(ReconstructedEnum.RED) # Outputs: ReconstructedEnum.RED
Though direct conversion back isn’t typical, reconstruction is an option here.
Summary of Methods
- String: Convert with
.name
and restore withEnum[name]
. - Integer: Use
.value
andEnum(value)
. - List: Directly iterate over Enum.
- Dictionary: Comprehensions suit well for conversions; Class redefinition helps in reverse.
- JSON: Utilize dictionaries for intermediate conversions.
- List of Strings: Enum names in a list simplify GUI use.
- Tuple: Direct use for immutable sequence requirements.
- Literal: Enhance type safety.
- Map: Apply transformations with
map
. - List of Tuples: Ideal for tuple-based structure requirements.
Using Python Enums effectively means understanding how to transform them to and from different types, broadening their usability across various aspects of Python programming.
Python Dictionary Comprehension: A Powerful One-Liner Tutorial
April 17, 2024 at 12:05AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
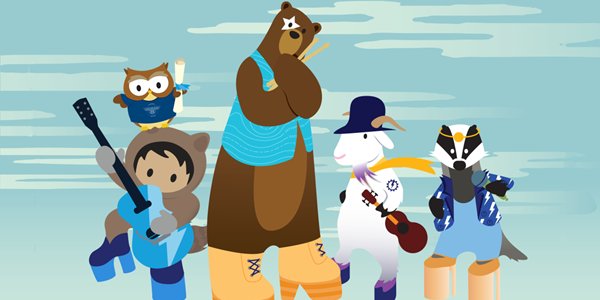
Post a Comment