Python Check If an Integer is in Range : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation
You’re given a number. How do you check if the number lies between two numbers, i.e., a numeric range?
When you check if a number lies between two other numbers it returns a boolean that determines if the number is greater than or equal to the minimum number and also less than or equal to the maximum number.
Here’s an example that demonstrates the problem:
Example 1: Is 25 between 15 and 35?Output:
True
Example 2: Is 0.5 between 1 and 5?Output:
False
Example 3: Is 10 between 10 and 20?Output:
True
Let’s dive into the trivial solution next — can you figure out why this is not optimal?
Method 1: Using Python’s in
Keyword with the range()
Function
You can use the range()
function to determine the upper and lower limits/numbers by feeding in the start and stop values within it. Now, to check if the number lies between the two numbers you can simply use the in
keyword to check it. So, if the number lies in the specified range then the output will be “True
” else “False
“.
Here’s a simple example:
print(25 in range(15, 36)) # True print(0.5 in range(0, 2)) # False print(10 in range(10, 20)) # True
Note that the stop value within the range()
function should always be one greater than the given maximum number/upper limit since the range of values taken into account by the range
function always lies between start to stop-1. Read more about the range()
function here:
Python
range()
Function — A Helpful Illustrated Guide
Info: Python’s “
in
” operator is a reserved keyword to test membership of the left operand in the right operand. The right operand, therefore, needs to be a collection type.
For example, the expression x in my_list checks
if object x
exists in the my_list
collection, so that at least one element y
exists in my_list
for that x == y
holds.
You can check membership using the “in
” operator in collections such as lists, sets, strings, and tuples.

This is not the optimal method because you need to create a whole range of values. Its runtime complexity depends on the size of the range which is really bad when compared to the better alternative:
Method 2: Use Comparison Operators
Python comparison operators can compare numerical values such as integers and floats in Python. The operators are:
- equal to ( == ),
- not equal to ( != ),
- greater than ( > ),
- less than ( < ),
- less than or equal to ( <= ), and
- greater than or equal to ( >= ).
You can use the <= and the >= operators to check if a number lies between the upper limit (maximum number) and lower limit(minimum number).
print(15 <= 25 < 35) # True print(1 <= 0.5 < 5) # False print(10 <= 10 < 20) # True
Check If a Number is Between Two Numbers in Python
Method 3: Using “and” Keyword with Comparison Operators
An alternative way of checking whether a value lies in a range of values is quite similar to the one used above. The only difference, in this case, is to use the and
keyword in between the two comparison operators as shown in the solution below.
print(15 <= 25 and 25 <= 35) # True print(1 <= 0.5 and 0.5 <= 5) # False print(10 <= 10 and 10 <= 20) # True
Some of you might be thinking why use an extra keyword when the solution given before is more readable than this one! Well! That’s true. The first syntax is more readable but this solution runs faster. Let’s compare the two solutions using timeit.
~$ python3 -m timeit "10 <= 20 and 20 <= 30" 10000000 loops, best of 3: 0.0366 usec per loop ~$ python3 -m timeit "10 <= 20 <= 30" 10000000 loops, best of 3: 0.0396 usec per loop
Quick Overview
Here are various ways to check if a Python integer, x
, falls within a specific range:
- Direct Comparison:
if low <= x <= high
for inclusive orif low < x < high
for exclusive. - Using
range()
:if x in range(low, high+1)
for inclusive orif x in range(low, high)
for exclusive. - Boolean Operators:
if x >= low and x <= high
for inclusive orif x > low and x < high
for exclusive. - Custom Function: Define a function like
def in_range(x, low, high): return low <= x <= high
for easy reuse. - Using
math
module:import math; if math.isclose(x, low, abs_tol=1e-9) or low < x < high
for near lower bound inclusivity. - List Comprehension:
if x in [i for i in range(low, high+1)]
offers explicit control over range elements.
List Comprehension in Python — A Helpful Illustrated Guide
April 24, 2024 at 05:00PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
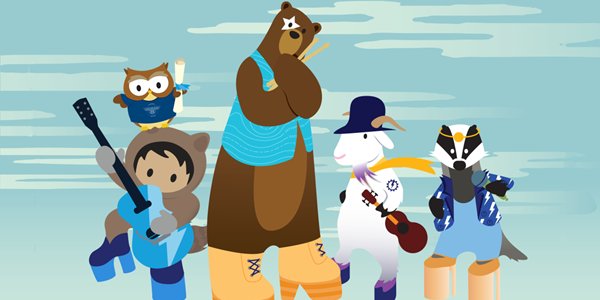
Post a Comment