How to Check Package Version in JavaScript? : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
You can access the version number from the package.json
file in your Node.js application by using the require()
function. This is a common method for accessing metadata like version numbers from the package definition.
Here’s how you can do it:
- Ensure that the
package.json
file is in the root directory of your Node.js project (or adjust the path accordingly in the code). - Use the following code snippet to load the
package.json
file and access theversion
field:
// Load the package.json file const packageJson = require('./package.json'); // Adjust the path if your package.json is not in the root directory // Access the version property const version = packageJson.version; console.log('The version of the package is:', version);
This code snippet will read the package.json
file relative to the location of the script that is being executed. If the script is in a different directory from package.json
, you’ll need to adjust the path to point to the correct location.
The require()
function here is used to import the JSON file as a JavaScript object, making it very simple to access any property defined within package.json
, such as version
.
But there are some alternatives:
Some Alternatives That May Work on Your Machine
Here are alternative methods to retrieve the version from package.json
in a Node.js application:
Using fs
Module to Read File Directly:
- Read
package.json
usingfs.readFileSync
. - Parse the JSON string with
JSON.parse
. - Access the
version
field.
const fs = require('fs'); const packageJson = JSON.parse(fs.readFileSync('./package.json', 'utf8')); const version = packageJson.version;
Using Environment Variables during Build Time:
- Set an environment variable based on
package.json
during the build/deployment process. - Access the version via
process.env.VERSION
.
# In your build script or deployment configuration VERSION=$(node -p "require('./package.json').version") && export VERSION
// In your Node.js code const version = process.env.VERSION;
Using Child Process to Execute Node Command:
Execute a Node.js command that reads package.json
using child_process.execSync
.
const { execSync } = require('child_process'); const version = execSync('node -p "require(\'./package.json\').version"').toString().trim();
April 21, 2024 at 04:11PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
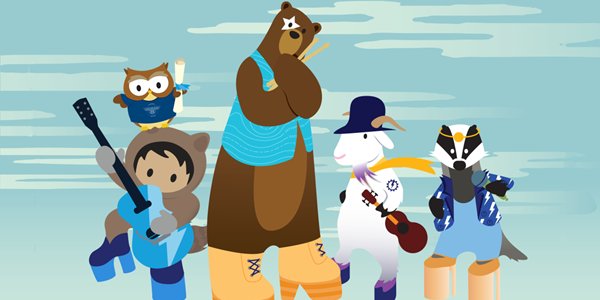
Post a Comment