Using the len() Function in Python
by:
blow post content copied from Real Python
click here to view original post
The len()
function in Python is a powerful and efficient tool used to determine the number of items in objects, such as sequences or collections. You can use len()
with various data types, including strings, lists, dictionaries, and third-party types like NumPy arrays and pandas DataFrames. Understanding how len()
works with different data types helps you write more efficient and concise Python code.
Using len()
in Python is straightforward for built-in types, but you can extend it to your custom classes by implementing the .__len__()
method. This allows you to customize what length means for your objects. For example, with pandas DataFrames, len()
returns the number of rows. Mastering len()
not only enhances your grasp of Python’s data structures but also empowers you to craft more robust and adaptable programs.
By the end of this tutorial, you’ll understand that:
- The
len()
function in Python returns the number of items in an object, such as strings, lists, or dictionaries. - To get the length of a string in Python, you use
len()
with the string as an argument, likelen("example")
. - To find the length of a list in Python, you pass the list to
len()
, likelen([1, 2, 3])
. - The
len()
function operates in constant time, O(1), as it accesses a length attribute in most cases.
In this tutorial, you’ll learn when to use the len()
Python function and how to use it effectively. You’ll discover which built-in data types are valid arguments for len()
and which ones you can’t use. You’ll also learn how to use len()
with third-party types like ndarray
in NumPy and DataFrame
in pandas, and with your own classes.
Free Bonus: Click here to get a Python Cheat Sheet and learn the basics of Python 3, like working with data types, dictionaries, lists, and Python functions.
Getting Started With Python’s len()
The function len()
is one of Python’s built-in functions. It returns the length of an object. For example, it can return the number of items in a list. You can use the function with many different data types. However, not all data types are valid arguments for len()
.
You can start by looking at the help for this function:
>>> help(len)
Help on built-in function len in module builtins:
len(obj, /)
Return the number of items in a container.
The function takes an object as an argument and returns the length of that object. The documentation for len()
goes a bit further:
Return the length (the number of items) of an object. The argument may be a sequence (such as a string, bytes, tuple, list, or range) or a collection (such as a dictionary, set, or frozen set). (Source)
When you use built-in data types and many third-party types with len()
, the function doesn’t need to iterate through the data structure. The length of a container object is stored as an attribute of the object. The value of this attribute is modified each time items are added to or removed from the data structure, and len()
returns the value of the length attribute. This ensures that len()
works efficiently.
In the following sections, you’ll learn about how to use len()
with sequences and collections. You’ll also learn about some data types that you cannot use as arguments for the len()
Python function.
Using len()
With Built-in Sequences
A sequence is a container with ordered items. Lists, tuples, and strings are three of the basic built-in sequences in Python. You can find the length of a sequence by calling len()
:
>>> greeting = "Good Day!"
>>> len(greeting)
9
>>> office_days = ["Tuesday", "Thursday", "Friday"]
>>> len(office_days)
3
>>> london_coordinates = (51.50722, -0.1275)
>>> len(london_coordinates)
2
When finding the length of the string greeting
, the list office_days
, and the tuple london_coordinates
, you use len()
in the same manner. All three data types are valid arguments for len()
.
The function len()
always returns an integer as it’s counting the number of items in the object that you pass to it. The function returns 0
if the argument is an empty sequence:
>>> len("")
0
>>> len([])
0
>>> len(())
0
In the examples above, you find the length of an empty string, an empty list, and an empty tuple. The function returns 0
in each case.
A range
object is also a sequence that you can create using range()
. A range
object doesn’t store all the values but generates them when they’re needed. However, you can still find the length of a range
object using len()
:
>>> len(range(1, 20, 2))
10
This range of numbers includes the integers from 1
to 19
with increments of 2
. The length of a range
object can be determined from the start, stop, and step values.
In this section, you’ve used the len()
Python function with strings, lists, tuples, and range
objects. However, you can also use the function with any other built-in sequence.
Read the full article at https://realpython.com/len-python-function/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
November 16, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
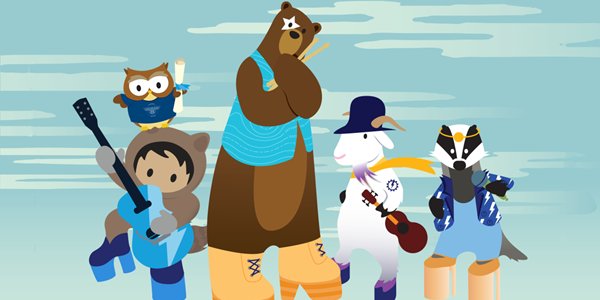
Post a Comment