String Interpolation in Python: Exploring Available Tools :
by:
blow post content copied from Real Python
click here to view original post
String interpolation allows you to create strings by inserting objects into specific places in a target string template. Python has several tools for string interpolation, including f-strings, the str.format()
method, and the modulo operator (%
). Python’s string
module also provides the Template
class, which you can use for string interpolation.
In this tutorial, you’ll:
- Learn how to use f-strings for eager string interpolation
- Perform lazy string interpolation using the
str.format()
method - Learn the basics of using the modulo operator (
%
) for string interpolation - Decide whether to use f-strings or the
str.format()
method for interpolation - Create templates for string interpolation with
string.Template
To get the most out of this tutorial, you should be familiar with Python strings, which are represented by the str
class.
Get Your Code: Click here to download the free sample code you’ll use to explore string interpolation tools in Python.
Take the Quiz: Test your knowledge with our interactive “String Interpolation in Python: Exploring Available Tools” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
String Interpolation in Python: Exploring Available ToolsTake this quiz to test your understanding of the available tools for string interpolation in Python, as well as their strengths and weaknesses. These tools include f-strings, the .format() method, and the modulo operator.
String Interpolation in Python
Sometimes, when working with strings, you’d make up strings by using multiple different string values. Initially, you could use the plus operator (+
) to concatenate strings in Python. However, this approach results in code with many quotes and pluses:
>>> name = "Pythonista"
>>> day = "Friday" # Of course 😃
>>> "Hello, " + name + "! Today is " + day + "."
'Hello, Pythonista! Today is Friday.'
In this example, you build a string using some text and a couple of variables that hold string values. The many plus signs make the code hard to read and write. Python must have a better and cleaner way.
Note: To learn more about string concatenation in Python, check out the Efficient String Concatenation in Python tutorial.
The modulo operator (%
) came to make the syntax a bit better:
>>> "Hello, %s! Today is %s." % (name, day)
'Hello, Pythonista! Today is Friday.'
In this example, you use the modulo operator to insert the name
and day
variables into the string literals. The process of creating strings by inserting other strings into them, as you did here, is known as string interpolation.
Note: Formatting with the modulo operator is inspired by printf()
formatting used in C and many other programming languages.
The %s
combination of characters is known as a conversion specifier. They work as replacement fields. The %
operator marks the start of the specifier, while the s
letter is the conversion type and tells the operator that you want to convert the input object into a string. You’ll learn more about conversion specifiers in the section about the modulo operator.
Note: In this tutorial, you’ll learn about two different types of string interpolation:
- Eager interpolation
- Lazy interpolation
In eager interpolation, Python inserts the values into the string at execution time in the same place where you define the string. In lazy interpolation, Python delays the insertion until the string is actually needed. In this latter case, you create string templates at one point in your code and fill the template with values at another point.
But the story doesn’t end with the modulo operator. Later, Python introduced the str.format()
method:
>>> "Hello, {}! Today is {}.".format(name, day)
'Hello, Pythonista! Today is Friday.'
The method interpolates its arguments into the target string using replacement fields limited by curly brackets. Even though this method can produce hard-to-read code, it represents a significant advance over the modulo operator: it supports the string formatting mini-language.
Note: String formatting is a fundamental topic in Python, and sometimes, people think that formatting and interpolation are the same. However, they’re not. In this tutorial, you’ll only learn about interpolation. To learn about string formatting and the formatting mini-language, check out the Python’s Format Mini-Language for Tidy Strings tutorial.
Python continues to evolve, and every new version brings new, exciting features. Python 3.6 introduced formatted string literals, or f-strings for short:
>>> f"Hello, {name}! Today is {day}."
'Hello, Pythonista! Today is Friday.'
F-strings offer a more readable and clean way to create strings that include other strings. To make an f-string, you must prefix it with an f
or F
. Again, curly brackets delimit the replacement fields.
Note: To learn more about f-strings, check out the Python’s F-String for String Interpolation and Formatting tutorial.
Read the full article at https://realpython.com/python-string-interpolation/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
June 03, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
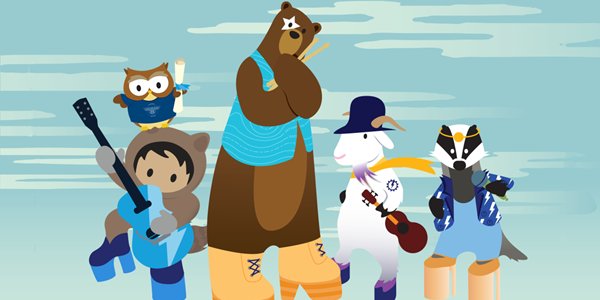
Post a Comment