Python's __all__: Packages, Modules, and Wildcard Imports
by:
blow post content copied from Real Python
click here to view original post
Python has something called wildcard imports, which look like from module import *
. This type of import allows you to quickly get all the objects from a module into your namespace. However, using this import on a package can be confusing because it’s not clear what you want to import: subpackages, modules, objects? Python has the __all__
variable to work around this issue.
The __all__
variable is a list of strings where each string represents the name of a variable, function, class, or module that you want to expose to wildcard imports.
In this tutorial, you’ll:
- Understand wildcard imports in Python
- Use
__all__
to control the modules that you expose to wildcard imports - Control the names that you expose in modules and packages
- Explore other use cases of the
__all__
variable - Learn some benefits and best practices of using
__all__
To get the most out of this tutorial, you should be familiar with a few Python concepts, including modules and packages, and the import system.
Get Your Code: Click here to download the free sample code that shows you how to use Python’s __all__
attribute.
Importing Objects in Python
When creating a Python project or application, you’ll need a way to access code from the standard library or third-party libraries. You’ll also need to access your own code from the multiple files that may make up your project. Python’s import system is the mechanism that allows you to do this.
The import system lets you get objects in different ways. You can use:
- Explicit imports
- Wildcard imports
In the following sections, you’ll learn the basics of both strategies. You’ll learn about the different syntax that you can use in each case and the result of running an import
statement.
Explicit Imports
In Python, when you need to get a specific object from a module or a particular module from a package, you can use an explicit import
statement. This type of statement allows you to bring the target object to your current namespace so that you can use the object in your code.
To import a module by its name, you can use the following syntax:
import module [as name]
This statement allows you to import a module by its name. The module must be listed in Python’s import path, which is a list of locations where the path based finder searches when you run an import.
The part of the syntax that’s enclosed in square brackets is optional and allows you to create an alias of the imported name. This practice can help you avoid name collisions in your code.
As an example, say that you have the following module:
calculations.py
def add(a, b):
return float(a + b)
def subtract(a, b):
return float(a - b)
def multiply(a, b):
return float(a * b)
def divide(a, b):
return float(a / b)
This sample module provides functions that allow you to perform basic calculations. The containing module is called calculations.py
. To import this module and use the functions in your code, go ahead and start a REPL session in the same directory where you saved the file.
Then run the following code:
>>> import calculations
>>> calculations.add(2, 4)
6.0
>>> calculations.subtract(8, 4)
4.0
>>> calculations.multiply(5, 2)
10.0
>>> calculations.divide(12, 2)
6.0
The import
statement at the beginning of this code snippet brings the module name to your current namespace. To use the functions or any other object from calculations
, you need to use fully qualified names with the dot notation.
Note: You can create an alias of calculations
using the following syntax:
import calculations as calc
This practice allows you to avoid name clashes in your code. In some contexts, it’s also common practice to reduce the number of characters to type when using qualified names.
For example, if you’re familiar with libraries like NumPy and pandas, then you’ll know that it’s common to use the following imports:
import numpy as np
import pandas as pd
Using shorter aliases when you import modules facilitates using their content by taking advantage of qualified names.
You can also use a similar syntax to import a Python package:
Read the full article at https://realpython.com/python-all-attribute/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
March 04, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
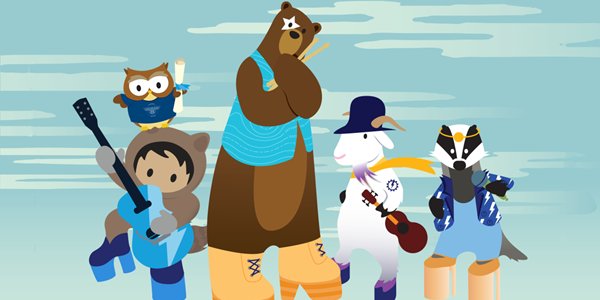
Post a Comment