5 Best Ways to Sort Tuples by Total Digits in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Imagine you have a list of tuples, each containing numeric data. You want to sort these tuples based on the cumulative count of digits across all numbers within each tuple. For instance, if you have
[ (123, 4), (12, 34), (1, 234) ]
, after sorting by total digits, the expected output would be [ (1, 234), (12, 34), (123, 4) ]
.
Method 1: Using a Custom Key Function
This method involves defining a custom key function that calculates the total number of digits for each tuple. The sorted()
function in Python takes this custom function as the key argument to sort the list of tuples.
Here’s an example:
def count_digits(tup): return sum(len(str(num)) for num in tup) tuples = [(123, 4), (12, 34), (1, 234)] sorted_tuples = sorted(tuples, key=count_digits) print(sorted_tuples)
The output will be:
[(1, 234), (12, 34), (123, 4)]
This code defines a function count_digits()
that computes the total digit count of a tuple by iterating over each number, converting it to a string, and summing up their lengths. The sorted()
function then uses this key function to order the list tuples
.
Method 2: Using Lambda within the Sorted Function
A lambda function is an anonymous, inline function in Python that can be used directly within the sorted function’s key argument. It is a succinct alternative to defining a separate function.
Here’s an example:
tuples = [(123, 4), (12, 34), (1, 234)] sorted_tuples = sorted(tuples, key=lambda tup: sum(len(str(num)) for num in tup)) print(sorted_tuples)
The output will be:
[(1, 234), (12, 34), (123, 4)]
The code snippet uses a lambda function to calculate the total number of digits directly in the sorted()
call, making it a more concise solution.
Method 3: Using List Comprehension and Zip
This method involves creating a list of digit counts using comprehension and then sorting the tuples based on this auxiliary list using the zip()
function.
Here’s an example:
tuples = [(123, 4), (12, 34), (1, 234)] digit_counts = [sum(len(str(num)) for num in tup) for tup in tuples] sorted_tuples = [tup for digit_count, tup in sorted(zip(digit_counts, tuples))] print(sorted_tuples)
The output will be:
[(1, 234), (12, 34), (123, 4)]
The code creates digit_counts
which maps each tuple to its total digit count. zip()
pairs these counts with their corresponding tuples. After sorting this zipped list, it extracts the sorted tuples.
Method 4: Sorting In-Place with Tuples’ sort() Method
Using the list.sort()
method, we can sort the tuples in place. Similar to using sorted()
, we can define a key function to achieve the intended sort order.
Here’s an example:
tuples = [(123, 4), (12, 34), (1, 234)] tuples.sort(key=lambda tup: sum(len(str(num)) for num in tup)) print(tuples)
The output will be:
[(1, 234), (12, 34), (123, 4)]
This example modifies the original list of tuples in place instead of creating a sorted copy, which can be more efficient in terms of memory usage.
Bonus One-Liner Method 5: Using a Generator Expression
By using a generator expression, we can perform the digit counting and sorting in a single, concise line of Python code.
Here’s an example:
tuples = [(123, 4), (12, 34), (1, 234)] sorted_tuples = sorted(tuples, key=lambda tup: sum(len(str(num)) for num in tup)) print(sorted_tuples)
The output will be:
[(1, 234), (12, 34), (123, 4)]
This method uses a generator expression to create an iterator for counting digits, which is memory efficient since it doesn’t create intermediate lists.
Summary/Discussion
- Method 1: Custom Key Function. Clear and modular. Requires defining an extra function.
- Method 2: Lambda in Sorted. Concise and quick. Can be less readable for complex calculations.
- Method 3: List Comprehension and Zip. Separates counting and sorting stages. More verbose and potentially less efficient due to extra list creation.
- Method 4: In-Place sort() Method. Efficient in memory. Alter the original list, which may not be desirable.
- Bonus Method 5: Generator Expression. Memory efficient and clean. May not offer any significant advantage over lambda in simple cases.
March 07, 2024 at 02:10AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
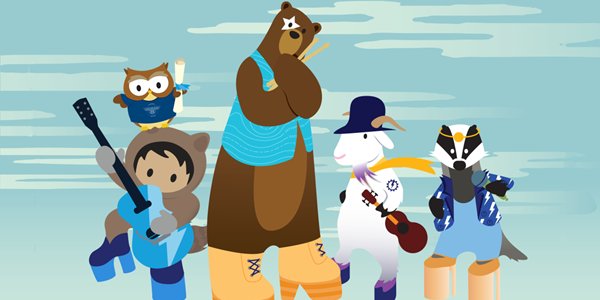
Post a Comment