5 Best Ways to Perform Incremental Slice Concatenation in Python String Lists : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: In Python, sometimes we need to concatenate slices from a list of strings in an incremental manner. For instance, given a list such as
['a', 'b', 'c', 'd']
, one may want to concatenate slices in a pattern that generates an output like ['ab', 'abc', 'abcd']
. This article covers five distinct methods to achieve this task, ensuring efficiency and readability.
Method 1: Using List Comprehension
This method utilizes list comprehension to create a new list by incrementally concatenating slices of the original list. The code loops over the range of indices and on each iteration, concatenates the elements from the beginning of the list up to the current index.
Here’s an example:
strings = ['a', 'b', 'c', 'd'] result = [''.join(strings[:i]) for i in range(2, len(strings) + 1)] print(result)
Output:
['ab', 'abc', 'abcd']
This snippet starts concatenation from the second element (index 1) until the final element, creating a gradually increasing sequence of concatenated strings. List comprehension offers a compact and readable solution for this problem.
Method 2: Iterative Concatenation with a Loop
An iterative approach involves initializing an empty result list and then appending the cumulative concatenation of each element from the original list within a loop. This method easily illustrates the concept of incremental addition.
Here’s an example:
strings = ['a', 'b', 'c', 'd'] result = [] for i in range(len(strings)): if i: result.append(result[-1] + strings[i]) print(result)
Output:
['ab', 'abc', 'abcd']
In this method, the loop skips the first item and starts appending from the second item, concatenating it with the previously appended string, thus building the output incrementally. It’s simple to understand but less concise than list comprehension.
Method 3: Using functools.reduce
The functools.reduce
function can be leveraged to apply a function of two arguments cumulatively to the items of a sequence. This method succinctly expresses the incremental concatenation process.
Here’s an example:
import functools strings = ['a', 'b', 'c', 'd'] result = [functools.reduce(lambda acc, x: acc + [acc[-1] + x], strings[1:], [strings[0]])] print(result)
Output:
['ab', 'abc', 'abcd']
Here, reduce
is accumulating a list with a lambda function that adds on to the previous value, demonstrating incremental concatenation using Python’s functional programming capabilities. It is elegant but can be less intuitive for those not familiar with functional programming constructs.
Method 4: Using itertools.accumulate
The itertools.accumulate
function generates a new iterator by returning accumulated sums or the accumulated results of a two-argument function. It offers a clear and efficient solution for string concatenation tasks.
Here’s an example:
import itertools strings = ['a', 'b', 'c', 'd'] result = list(itertools.accumulate(strings, lambda x, y: x + y))[1:] print(result)
Output:
['ab', 'abc', 'abcd']
The code above uses accumulate
to concatenate elements cumulatively. The lambda function specifies that the operation is concatenation. The slicing at the end excludes the first element, aligning the result with the problem’s requirements. It’s both concise and clear.
Bonus One-Liner Method 5: Using a Generator Expression
A generator expression is another compact method that resembles list comprehension but generates items on the fly rather than storing them in a list. It’s particularly memory-efficient for large datasets.
Here’s an example:
strings = ['a', 'b', 'c', 'd'] result = list(''.join(strings[:i]) for i in range(2, len(strings)+1)) print(result)
Output:
['ab', 'abc', 'abcd']
This code uses a generator expression within a list constructor for the same effect as Method 1, but it is suited for situations where memory usage is a concern. The expression is intuitive and elegant, making it an excellent one-liner solution.
Summary/Discussion
- Method 1: List Comprehension. Quick and easy to write. May consume more memory for very large lists.
- Method 2: Iterative Concatenation with a Loop. Straightforward and explicit. Slightly more verbose and possibly less pythonic.
- Method 3: Using
functools.reduce
. Functional programming approach. Less readable for those unfamiliar with the reduce function. - Method 4: Using
itertools.accumulate
. Pythonic and efficient. May require familiarity with the itertools module. - Method 5: Generator Expression. Memory-efficient. Generates items on-the-fly, which can be slower for immediate full list generation.
March 07, 2024 at 02:10AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
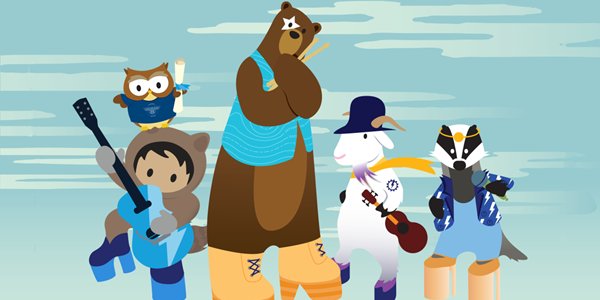
Post a Comment