5 Best Ways to Convert Python CSV Bytes to String : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: When dealing with CSV files in Python, particularly when reading from binary streams such as files opened in binary mode or from network sources, you might receive byte strings. The challenge is converting these CSV byte strings into a standard string format for easier manipulation and readability. Suppose you have a byte string representing CSV data, the objective is to transform it to a string looking like “name,age\nAlice,30\nBob,25”.
Method 1: Using decode()
The decode()
function is the most straightforward method to convert bytes to a string in Python. It takes the encoding format as an argument and returns the string represented by the byte data. This function is especially useful for converting CSV data read from binary files.
Here’s an example:
csv_bytes = b'name,age\\nAlice,30\\nBob,25' string_csv = csv_bytes.decode('utf-8') print(string_csv)
Output:
name,age Alice,30 Bob,25
In this snippet, we have a byte string of CSV data that we want to convert to a regular string. By calling .decode('utf-8')
on our byte string, we convert it to a UTF-8 encoded string, which is the standard text format in Python.
Method 2: Using io.StringIO()
The io.StringIO()
module is a Python in-memory stream for text I/O. By decoding the bytes to a string and passing it to StringIO()
, you can treat it like a file object, which can be particularly useful for reading CSV data using the built-in CSV module.
Here’s an example:
import io csv_bytes = b'name,age\\nAlice,30\\nBob,25' string_io = io.StringIO(csv_bytes.decode('utf-8')) print(string_io.read())
Output:
name,age Alice,30 Bob,25
Here, the byte string is first decoded using .decode('utf-8')
, and then passed to io.StringIO()
. The resulting object behaves like a file, allowing us to call .read()
on it to get the entire string content.
Method 3: Using Pandas
Pandas is a powerful data manipulation library that can read a CSV byte string into a DataFrame, and then convert it to a string with its to_csv()
method. This method is useful when you want to work with CSV data in a tabular format.
Here’s an example:
import pandas as pd from io import BytesIO csv_bytes = b'name,age\\nAlice,30\\nBob,25' df = pd.read_csv(BytesIO(csv_bytes)) print(df.to_csv(index=False))
Output:
name,age Alice,30 Bob,25
In this example, we used the BytesIO()
from the io module to trick Pandas into thinking it’s reading from a file. Then the read_csv()
function is utilized to read the byte string into a DataFrame. Finally, to_csv(index=False)
converts it back to a string, omitting the DataFrame’s index.
Method 4: Using CSV Module Directly
The CSV module provides functions to directly work with CSV files. By combining csv.reader()
with StringIO()
, you can read byte strings as if they were CSV files. This method is useful if you want to use functionalities specific to the CSV module.
Here’s an example:
import csv import io csv_bytes = b'name,age\\nAlice,30\\nBob,25' string_io = io.StringIO(csv_bytes.decode('utf-8')) csv_reader = csv.reader(string_io) for row in csv_reader: print(','.join(row))
Output:
name,age Alice,30 Bob,25
The example decodes the byte string into a string, passes it to StringIO()
, and then to csv.reader()
. We iterate over the CSV reader object and print each row, joining the columns with commas.
Bonus One-Liner Method 5: Chaining Methods
For quick conversions without additional variable assignments, one can chain the above methods into a one-liner. This is useful for limited, on-the-fly conversions.
Here’s an example:
import io import csv csv_bytes = b'name,age\\nAlice,30\\nBob,25' print("".join([','.join(row) for row in csv.reader(io.StringIO(csv_bytes.decode('utf-8')))]))
Output:
name,ageAlice,30Bob,25
This one-liner decodes the bytes, passes them to StringIO()
, and then into csv.reader()
. We use a list comprehension to join each row back into a string and concatenate all rows into one big string.
Summary/Discussion
- Method 1: Using
decode()
: Simple and direct. Strengths: Easy and quick for small data. Weaknesses: Lacks direct CSV parsing features. - Method 2: Using
io.StringIO()
: More flexible, allows for file-like operations. Strengths: Simulates a file object; useful for integrating with other modules. Weaknesses: Extra step of decoding before use. - Method 3: Using Pandas: Great for data analysis tasks. Strengths: Powerful data manipulation, handles complex CSV formats. Weaknesses: Requires installing Pandas, overkill for simple tasks.
- Method 4: Using CSV Module Directly: Native CSV parsing. Strengths: No third-party modules required, specialized for CSV. Weaknesses: Requires multiple steps for reading and writing.
- Method 5: Chaining Methods: Compact and convenient for one-off tasks. Strengths: Quick and elegant one-liner. Weaknesses: Can be harder to read and maintain.
March 02, 2024 at 03:41AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
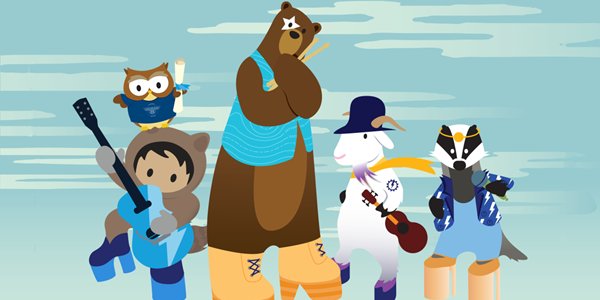
Post a Comment