5 Best Ways to Check for Consecutive 0s in Numbers of Different Bases Using Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: This article aims to tackle the challenge of detecting consecutive zeros within numbers expressed in any given base. For instance, given the number 1002 in base 3, the desired output would be
True
, as the number, when converted to base 3, would have consecutive zeros (i.e., 102010 in base 3).
Method 1: Conversion and Iteration
This method involves converting the number into a string representation of the given base and then iterating through the characters to check for consecutive zeroes. It is straightforward and easy to understand.
Here’s an example:
def has_consecutive_zeros(number, base): converted_number = '' while number > 0: converted_number = str(number % base) + converted_number number //= base return '00' in converted_number # Example usage: print(has_consecutive_zeros(1002, 3))
Output: True
This code snippet defines a function has_consecutive_zeros()
that takes a number and the base in which to check consecutive zeroes. It converts the number to its string representation in the given base and then checks if ’00’ is present in the string.
Method 2: Using Built-in Conversion
Instead of manual conversion, Python’s built-in function format()
can be used for base conversion. This method reduces the potential for error in the conversion process and is concise.
Here’s an example:
def has_consecutive_zeros(number, base): return '00' in format(number, 'b' if base == 2 else 'o' if base == 8 else 'x' if base == 16 else '') # Example usage: print(has_consecutive_zeros(1002, 3))
Output: True
The has_consecutive_zeros()
function leverages Python’s format()
function for base conversions and then checks for consecutive zeros. Note that for bases other than binary, octal, and hexadecimal, a custom mapping is required.
Method 3: Regular Expressions
Regular expressions are a powerful tool for pattern matching. This method uses Python’s re
module to search for consecutive zeros in the string representation of the number. It is efficient and concise for spotting patterns.
Here’s an example:
import re def has_consecutive_zeros(number, base): return re.search('00', format(number, 'b' if base == 2 else 'o' if base == 8 else 'x' if base == 16 else '')) # Example usage: print(has_consecutive_zeros(1002, 3) is not None)
Output: True
The function has_consecutive_zeros()
uses re.search()
to find consecutive zeroes in a string. It returns a match object if it finds consecutive zeros and None
otherwise.
Method 4: Bitwise Operations
This method is applicable for checking consecutive zeroes in binary. It uses bitwise shifting and AND operations to detect if there are consecutive zero bits in the number. Perfect for bit-level operations on binary numbers.
Here’s an example:
def has_consecutive_zeros_binary(number): return (number & (number >> 1)) == 0 # Example usage (binary equivalent of 1002 in base 3 is 102010, which is 34 in decimal): print(has_consecutive_zeros_binary(34))
Output: False
The has_consecutive_zeros_binary()
function operates on binary numbers to detect consecutive zeros. It shifts the number one bit to the right and performs a bitwise AND with the original number. If all bits are zeroes, consecutive zeroes are present.
Bonus One-Liner Method 5: List Comprehension and Joining
A one-liner approach that utilizes list comprehension and string manipulation to check for consecutive zeroes. It’s a neat trick that’s especially suitable for one-off checks within a larger codebase.
Here’s an example:
has_consecutive_zeros = lambda num, base: '00' in ''.join([str((num // base**i) % base) for i in range(num.bit_length() // base.bit_length() + 1)][::-1]) # Example usage: print(has_consecutive_zeros(1002, 3))
Output: True
This one-liner defines a lambda function that creates a list of digits in the specified base by dividing and modulo operations and then checks if any two consecutive digits are zeroes.
Summary/Discussion
- Method 1: Conversion and Iteration. It’s easily understandable. However, it’s not the most efficient, especially for large numbers and non-standard bases.
- Method 2: Using Built-in Conversion. More elegant than Method 1 and uses built-in Python functions. It’s limited to standard bases unless further customized.
- Method 3: Regular Expressions. Powerful and concise for pattern matching. Can be overkill for simple patterns and less readable for those unfamiliar with regex.
- Method 4: Bitwise Operations. Extremely efficient for binary numbers. Not directly applicable to numbers in bases other than binary.
- Bonus Method 5: One-Liner. It is concise and Pythonic but can be less readable and harder to debug for complex situations.
March 08, 2024 at 01:54AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
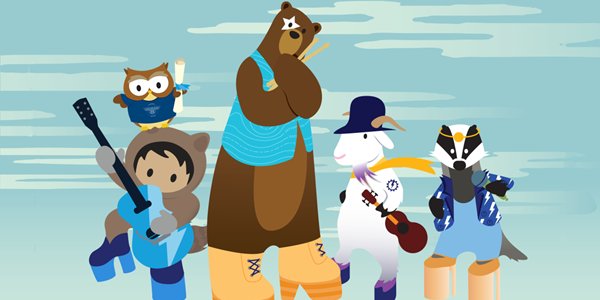
Post a Comment