5 Best Ways to Iterate Through a List of Dictionaries in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: When working with data in Python, it’s common to encounter a list of dictionaries. Each dictionary represents a record with key-value pairs, similar to a row in a database or a spreadsheet. Suppose you have a list of dictionaries, where each dictionary contains information about a student, and you want to iterate over this list to process each student’s details. How do you effectively traverse this collection to access or modify the data contained within? This article presents five methods for iterating through a list of dictionaries in Python, showcasing the versatility and power of Python’s iteration capabilities.
Method 1: Using a Simple For Loop
The simplest and most straightforward method to iterate through a list of dictionaries is by using a for
loop. This approach requires iterating over each dictionary in the list and then accessing the desired keys and their values within the loop’s body.
Here’s an example:
student_records = [ {'name': 'Alice', 'grade': 'A'}, {'name': 'Bob', 'grade': 'B'}, {'name': 'Charlie', 'grade': 'C'} ] for student in student_records: print(f"Name: {student['name']}, Grade: {student['grade']}")
Output:
Name: Alice, Grade: A Name: Bob, Grade: B Name: Charlie, Grade: C
This code snippet demonstrates how to iterate over a list of dictionaries named student_records
, printing out each student’s name and grade. The loop goes through each dictionary (representing a student) and accesses the values associated with the keys ‘name’ and ‘grade’ to generate a formatted output.
Method 2: Using the map() Function
Python’s map()
function applies a given function to each item of an iterable (like our list of dictionaries) and returns a list of the results. This functional programming approach can be concise and expressive, often resulting in cleaner code for simple transformations.
Here’s an example:
student_records = [ {'name': 'Alice', 'grade': 'A'}, {'name': 'Bob', 'grade': 'B'}, {'name': 'Charlie', 'grade': 'C'} ] def print_student(student): print(f"Name: {student['name']}, Grade: {student['grade']}") list(map(print_student, student_records))
Output:
Name: Alice, Grade: A Name: Bob, Grade: B Name: Charlie, Grade: C
In this snippet, the print_student
function is defined to print a single student’s details. The map()
function then applies this function to each element in the student_records
list. Note that list()
is used to trigger the execution of the map()
object in Python 3, as map()
returns an iterator.
Method 3: Using List Comprehensions
List comprehensions provide a concise way to create lists and can also be used to iterate over a list with an implicit loop. In this method, we can perform actions on each dictionary in our list in a single line of code.
Here’s an example:
student_records = [ {'name': 'Alice', 'grade': 'A'}, {'name': 'Bob', 'grade': 'B'}, {'name': 'Charlie', 'grade': 'C'} ] [print(f"Name: {student['name']}, Grade: {student['grade']}") for student in student_records]
Output:
Name: Alice, Grade: A Name: Bob, Grade: B Name: Charlie, Grade: C
This code uses a list comprehension to iterate over student_records
, printing each student’s information. While this is compact and often Pythonic, it’s worth noting that using list comprehensions solely for side effects like printing is discouraged in favor of more explicit loops or other methods.
Method 4: Using the filter() Function
If you need to iterate over a list of dictionaries but only process a subset that satisfies certain conditions, the filter()
function is an excellent choice. Like map()
, filter()
takes a function and an iterable, but it returns only the items for which the function returns True
.
Here’s an example:
student_records = [ {'name': 'Alice', 'grade': 'A'}, {'name': 'Bob', 'grade': 'B'}, {'name': 'Charlie', 'grade': 'C'} ] def is_grade_a(student): return student['grade'] == 'A' grade_a_students = filter(is_grade_a, student_records) for student in grade_a_students: print(f"Name: {student['name']}, Grade: {student['grade']}")
Output:
Name: Alice, Grade: A
This snippet defines the is_grade_a
function, which checks if a student’s grade is ‘A’. The filter()
function uses this to filter student_records
, and then a for
loop prints the details of students with an ‘A’ grade. This method can help in reducing the number of iterations if only a subset of records need to be processed.
Bonus One-Liner Method 5: Using Dictionary Unpacking in a For Loop
dictionary unpacking can make the iteration more readable by directly extracting the values of interest from each dictionary as the loop proceeds.
Here’s an example:
student_records = [ {'name': 'Alice', 'grade': 'A'}, {'name': 'Bob', 'grade': 'B'}, {'name': 'Charlie', 'grade': 'C'} ] for {'name': name, 'grade': grade} in student_records: print(f"Name: {name}, Grade: {grade}")
Output:
Name: Alice, Grade: A Name: Bob, Grade: B Name: Charlie, Grade: C
This snippet uses dictionary unpacking in the for
loop declaration to extract the ‘name’ and ‘grade’ directly from each dictionary, which are then printed. This technique enhances readability by making the intention clear and the code cleaner but is only applicable in Python 3.6 and later due to the introduction of PEP 448, which expanded the unpacking generalizations.
Summary/Discussion
- Method 1: Simple For Loop. Easy to understand and widely applicable. It can be slightly verbose when dealing with complex data structures.
- Method 2: Using map(). Functional-style iteration that promotes code reuse. It may be less readable to those unfamiliar with the functional programming paradigm.
- Method 3: List Comprehensions. Pythonic and succinct for operations that result in a new list. It is considered bad practice when used for side effects like printing instead of generating new lists.
- Method 4: Using filter(). Excellent for conditional iteration. Similar to
map()
, it can be less intuitive for those new to functional programming and requires an additional step of iterating over the resulting filter object. - Bonus Method 5: Dictionary Unpacking. Improves readability by extricating values directly during iteration. It only works in more recent Python versions and could lead to errors if dictionaries have missing or additional keys.
February 22, 2024 at 07:25PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
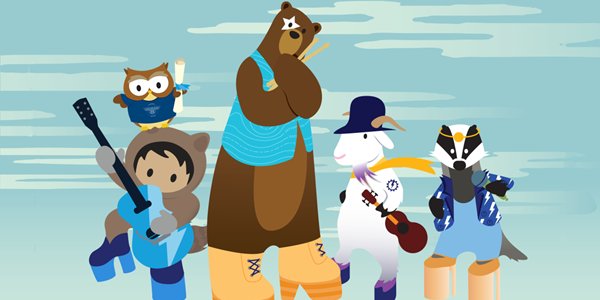
Post a Comment