5 Best Ways to Create Subplots with Plotly in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Visualizing different datasets or aspects of data side by side can be very insightful. However, creating subplots in visualization can often be intricate. This article aims to describe different methods to create subplots in Python using the Plotly library, allowing you to visualize multiple plots in a single view. Imagine wanting to compare different stock’s performance within the same timeframe. The input would be several time series data, and the desired output is a grid-like visualization where each stock’s graph is a subplot.
Method 1: Using make_subplots and add_trace
This method involves creating a subplot layout using Plotly’s make_subplots
function, then populating it with plots using the add_trace
function. This method is flexible as you can define the number of rows and columns for the subplots and can specify the type of plots in each subplot.
Here’s an example:
from plotly.subplots import make_subplots import plotly.graph_objs as go fig = make_subplots(rows=2, cols=2) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6]), row=1, col=1) fig.add_trace(go.Bar(x=[1, 2, 3], y=[6, 5, 4]), row=1, col=2) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6]), row=2, col=1) fig.add_trace(go.Bar(x=[1, 2, 3], y=[6, 5, 4]), row=2, col=2) fig.show()
Output: A 2×2 subplot grid with top left and bottom left as line plots and top right and bottom right as bar plots.
The above code creates a subplot grid of 2 rows and 2 columns. It then adds a line plot to the first cell and a bar plot to the second cell using the add_trace
method, specifying the appropriate row and column for each trace. By calling fig.show()
, it displays the resulting subplots.
Method 2: Plotly Express with Facet Row and Facet Column
Plotly Express functions allow the creation of faceted plots using the facet_row
or facet_column
arguments. This is a concise method when dealing with categorical data or if you want to create subplots based on a specific data dimension.
Here’s an example:
import plotly.express as px df = px.data.gapminder() fig = px.scatter(df, x="gdpPercap", y="lifeExp", color="continent", facet_col="continent") fig.show()
Output: Subplots separated into columns based on the unique values of the ‘continent’ field, comparing GDP per capita and life expectancy for each continent.
In this example, the px.scatter
function is used to create a scatter plot with GDP per capita on the x-axis and life expectancy on the y-axis. The facet_col
argument creates a subplot for each continent. The result is a clear and comparative visualization of data segments.
Method 3: Using Subplot Titles and Annotations
This method extends the use of the make_subplots
function by customizing subplot titles and adding annotations for better readability. This improves the subplot presentation by providing context to each subplot.
Here’s an example:
fig = make_subplots(rows=2, cols=1, subplot_titles=("Plot 1", "Plot 2")) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6]), row=1, col=1) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[6, 7, 8]), row=2, col=1) fig.update_annotations(dict(font_size=12, showarrow=False)) fig.show()
Output: Two vertically stacked subplots with custom titles “Plot 1” and “Plot 2”.
The code illustrates how to add titles to each subplot in a vertical stack using subplot_titles
. It adds two scatter plots, one in each subplot. The update_annotations
method is used to style the annotations.
Method 4: Mixed-Type Subplots
Plotly allows the creation of subplots with different types of plots in each subplot, such as scatter plots, bar charts, and more, within the same figure. This is particularly useful for comparing different styles of data visualization.
Here’s an example:
fig = make_subplots(rows=1, cols=2, specs=[[{"type": "scatter"}, {"type": "bar"}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6]), row=1, col=1) fig.add_trace(go.Bar(x=[1, 2, 3], y=[6, 5, 4]), row=1, col=2) fig.show()
Output: One row with two subplots, where the first is a scatter plot and the second is a bar chart.
This code block demonstrates how to create a row of subplots where each subplot can be a different type, specified in the specs
argument of the make_subplots
function. A scatter plot is placed in the first subplot and a bar chart in the second subplot.
Bonus One-Liner Method 5: Using make_subplots with rows and shared_xaxes
For a quick and straightforward subplot creation, one can use a single line of code to create subplots that share the same x-axis using make_subplots
with the shared_xaxes
attribute.
Here’s an example:
fig = make_subplots(rows=2, shared_xaxes=True) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6]), row=1, col=1) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[6, 7, 8]), row=2, col=1) fig.show()
Output: Two vertically stacked subplots that share the same x-axis.
Using the shared_xaxes
argument creates subplots with a common x-axis, making it easier to compare trends over the same range or time period. This one-liner is powerful for time-series comparisons.
Summary/Discussion
Method 1: Using make_subplots and add_trace. Offers high flexibility and control over subplot layout and content. Can combine different plot types but requires more verbose code.
Method 2: Plotly Express with Facet Row and Facet Column. Best for quickly creating faceted subplots based on data dimensions. Limited to using Plotly Express compatible plots.
Method 3: Using Subplot Titles and Annotations. Enhances subplots with descriptive titles and customizable annotations. Adds clarity, but involves additional setup.
Method 4: Mixed-Type Subplots. Allows for diverse visual comparisons within the same figure. Requires careful arrangement of subplot types but is very informative.
Method 5: Bonus One-Liner with shared_xaxes. The simplest method for creating aligned subplots quickly. Best for time series but limited to shared axis scenarios.
February 28, 2024 at 06:28PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
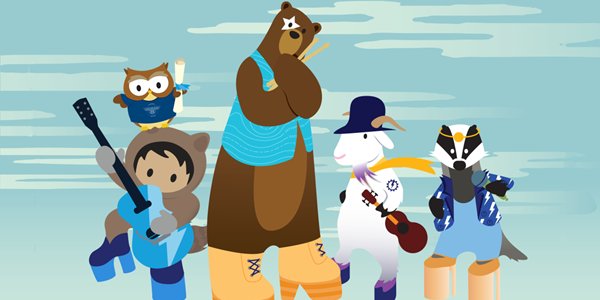
Post a Comment