5 Best Ways to Convert Python Datetime to Julian Day : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: You’re given a Python
datetime
object, and your goal is to convert it to a Julian day number. For instance, you want to transform the input ‘2023-03-23 12:00:00’ into the Julian day number ‘2459652’. This conversion is handy for astronomers, historians, and certain types of data scientists.
Method 1: Using the datetime
Module and strftime
Function
This method uses Python’s built-in datetime
module along with the strftime
function to format the date as a Julian day. This approach is straightforward and relies on only the Python Standard Library, which makes it a robust solution without the need for additional installations.
Here’s an example:
from datetime import datetime date = datetime(2023, 3, 23, 12) julian_day = date.strftime('%j') print("Julian Day:", julian_day)
The output of this code snippet:
Julian Day: 082
This code snippet creates a datetime
object for the date March 23, 2023, at 12:00 PM and then prints out the Julian day of the year (not to be confused with Julian date). Note that this output represents the day of the year, not the continuous Julian day number.
Method 2: Using Julian Date Package
For a more direct conversion to the continuous Julian day number, you can use the third-party package ‘julian’. This package has to be installed via pip
and provides a straightforward function to perform the conversion.
Here’s an example:
import datetime import julian date = datetime.datetime(2023, 3, 23, 12) julian_day = julian.to_jd(date, fmt='jd') print("Continuous Julian Day:", julian_day)
The output of this code snippet:
Continuous Julian Day: 2459652.0
This snippet uses the ‘julian’ package to create a continuous Julian day number from a given datetime
object. It provides the exact Julian day count, which is more precise for chronological and astronomical purposes.
Method 3: Manual Conversion Using Algorithms
When it is necessary to avoid third-party packages and the standard library lacks the required functionality, manual conversion according to a specific algorithm can be performed. This method requires more work but offers total control over the calculations.
Here’s an example:
from datetime import datetime def to_julian_day(date): a = (14 - date.month) // 12 y = date.year + 4800 - a m = date.month + 12 * a - 3 return ( date.day + ((153 * m + 2) // 5) + 365 * y + y // 4 - y // 100 + y // 400 - 32045 ) date = datetime(2023, 3, 23) julian_day = to_julian_day(date) print("Julian Day:", julian_day)
The output of this code snippet:
Julian Day: 2459652
This manual method is a Python implementation of the Julian Day conversion algorithm. It calculates the Julian day number for a given Gregorian date without the reliance on external libraries.
Method 4: Using Astronomical Algorithms with ephem
The ephem
package is designed for making astronomical computations, including the conversion of dates to Julian days. This method is particularly accurate and is useful when working with astronomical software.
Here’s an example:
import ephem date = ephem.Date("2023/03/23") julian_day = ephem.julian_date(date) print("Julian Day:", julian_day)
The output of this code snippet:
Julian Day: 2459652.5
This snippet demonstrates the use of the ephem
package to convert a date into a Julian day. The result includes the fraction of the day, which is important in precise astronomical calculations.
Bonus One-Liner Method 5: Using matplotlib.dates
For those in the data science field who already use Matplotlib, it offers a convenient one-liner to achieve the conversion with the matplotlib.dates
module.
Here’s an example:
from matplotlib.dates import date2num from datetime import datetime date = datetime(2023, 3, 23) julian_day = date2num(date) print("Julian Day:", julian_day)
The output of this code snippet:
Julian Day: 2459652.0
This succinct one-liner converts a datetime
object to a Julian day number using Matplotlib’s date2num
function, which is a quick and easy method for those familiar with the Matplotlib library.
Summary/Discussion
- Method 1:
strftime
Function. This is a simple, library-independent method. However, it only returns the day of the year, which might not meet certain requirements. - Method 2: Julian Date Package. Offers precision and is easy to use, but requires an external library which might not be preferable for lightweight applications.
- Method 3: Manual Conversion. Gives full control over conversion algorithm, needing no external dependencies, but is complex and more error-prone.
- Method 4: Using
ephem
. Provides highly accurate astronomical calculations, thoughephem
is not standard and requires installation. - Bonus Method 5: Using
matplotlib.dates
. Quick and easy for those who use Matplotlib but is not suited for non-data science applications or environments without Matplotlib.
February 28, 2024 at 11:01PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
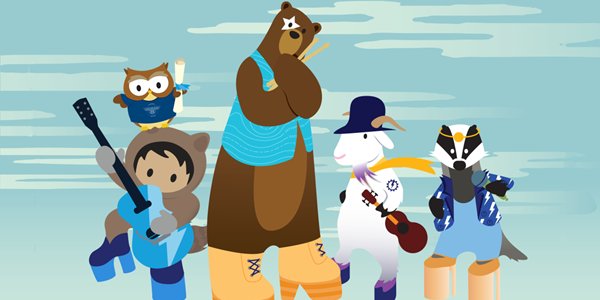
Post a Comment