Python Print String Format: Mastering Output Aesthetics : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
In the dynamic world of Python programming, the ability to produce clear and well-formatted output is essential. Whether you’re generating reports, displaying messages, or logging data, how you present information can significantly enhance readability and understanding. Python offers several string formatting techniques, each with its own advantages, allowing you to tailor your output to your specific needs.
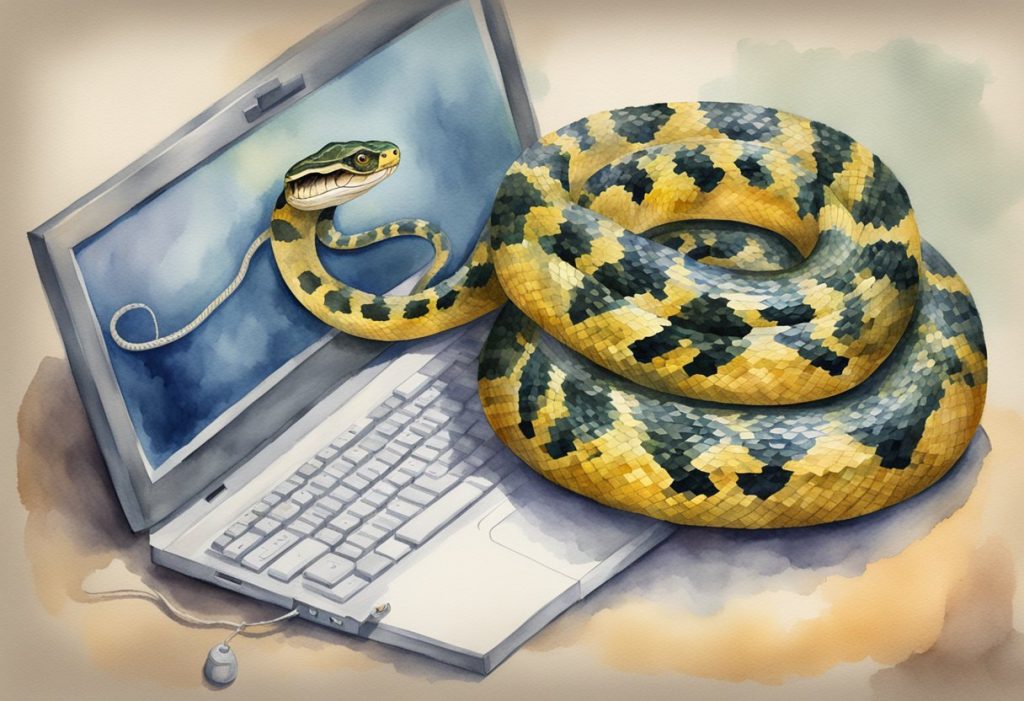
Understanding Python string formatting starts with familiarizing yourself with the older modulus %
operator and progresses to the more versatile .format()
method. The .format()
method provides numerous ways to control the alignment, width, and precision of the formatted string. On the cutting edge, formatted string literals, also known as f-strings, offer a succinct and readable way to embed expressions inside string literals, simply by prefixing the string with f
or F
. Each technique has its place, and mastering them enables you to write more readable and efficient Python code.
As you venture through writing Python code, you’ll encounter scenarios that require different methods of string formatting. Whether you need to interpolate variables seamlessly into strings with f-strings, use the percentage operator for simple placeholders, or leverage the powerful .format()
method for more complex scenarios, learning these approaches will sharpen your coding expertise. This knowledge will ensure that your strings are not just informative but also formatted in the most appropriate style for any given task.
Basics of String Formatting in Python

When you work with strings in Python, formatting is an essential skill that allows you to control the way strings are presented and combined with other data types. Let’s explore the core methods of formatting strings effectively in Python.
Understanding String Literals and Placeholders
String literals are the text you provide in code, demarcated by quotes. Placeholders, represented by brace notation {}
, serve as the targets within string literals where you can insert values. For example, in the string "Hello, {}!"
, the {}
is a placeholder for a name or other piece of information you want to include.
The Format Function and Its Uses
The str.format()
method replaces placeholders in a string with provided values. This method offers flexibility, as you can use positional arguments like "{0} {1}".format("Hello,", "world!")
or keyword arguments like "{greeting} {name}".format(greeting="Hello,", name="world!")
. The placeholders can also include format specifiers that modify the output, such as adjusting number precision or adding padding.
String Formatting with f-strings
Python 3.6 introduced formatted string literals, or f-strings, which allow for inline expressions using {expression}
syntax. Write a variable name or an expression inside the curly braces, and it will be evaluated and converted into a string directly: f"Result is {value * 2}"
. F-strings are a concise and readable way to embed expressions within string literals.
Template Strings and Advanced Formatting
Template strings, part of Python’s string module’s Template
class, offer an alternative way of substituting values into strings using $
syntax, like Template("Hello, $name!").substitute(name="world")
. They are simpler and less powerful compared to str.format()
but are useful for user-provided formatting strings due to their reduced complexity.
Practical Applications and Output Formatting

In this section, you’ll learn how to apply various formatting strategies to enhance the readability and precision of your Python output, enabling you to handle different data types and files, as well as troubleshoot common issues effectively.
Formatting for Readability
When you use the print()
function, readability is key. To make your output human-readable, especially when dealing with lists or large amounts of data, you can include spaces or commas for separation. For example, to print each item in a list on a new line, you could iterate over the list and print each element with print(item)
.
Additionally, you can employ format fields to control the alignment and spacing within your formatted strings. To left align, right align, or center align your text within a certain number of spaces, you would use -
, >
, or ^
respectively. For instance:
names_list = ["Alice", "Bob", "Charlie"] for name in names_list: print(f"{name:<10}", end="") # Left align
Customizing Formats for Various Data Types
Adjusting the format for different data types enhances clarity and precision. You might require different formatting types like:
- Binary format: To display a number in binary format, use
b
. For example:format(5, 'b')
produces'101'
. - Decimal format: For decimal numbers, use
d
. Like:format(123, 'd')
. - General format: This format, represented by
g
, automatically chooses between fixed-point and exponential notation.
Customizing the display of numbers further with signs is also possible: for positive numbers, +
will prepend a plus sign, while -
will only show the minus sign for negative numbers. An underscore _
can be used as a thousands separator as well:
print(f"{123456789:_}")
Handling Files and Streams
Output formatting goes beyond the console; formatting when writing to text files or using streams like sys.stdout
is equally crucial. You must use the .write()
method when outputting to a file. Incorporating newlines (\n
) is essential for formatting when writing to files since write()
does not add them by default:
with open("output.txt", "w") as file: file.write("Hello, world!\n")
Be aware that newlines differ between Windows (\r\n
) and Unix (\n
), as this affects the readability of your written files across different operating systems.
Troubleshooting Common Formatting Issues
Encountering bugs in output formatting is part of the Python journey. Common issues include misplaced format fields or incorrect formatting strings, but these can often be resolved by checking the syntax of your Python expressions. If you receive a Traceback
, review it carefully; it’s the interpreter’s way of guiding you to the issue. When debugging, isolate and test small parts of the formatted string to pinpoint the specific problem.
Frequently Asked Questions
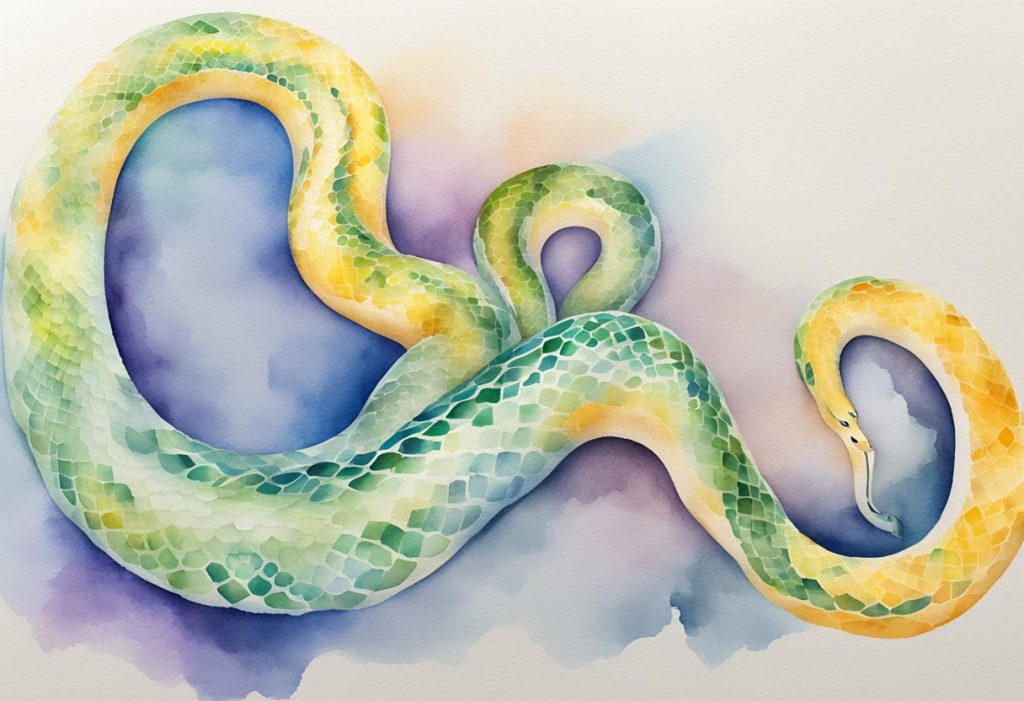
In this section, we’ll explore common inquiries related to Python’s string formatting capabilities, offering clear and precise answers to enhance your understanding and application of these techniques.
How can you use f-strings to interpolate variables into a string in Python?
To interpolate variables into a string, you can use f-strings, which were introduced in Python 3.6. Place an f
before your string and enclose the variables in curly braces within the string. For example: name = "Alice"
; using f"Hello, {name}!"
would output 'Hello, Alice!'
.
What is the syntax to format a floating-point number to two decimal places using Python?
To format a floating-point number to two decimal places, you can use the format specifier :.2f
. For instance, number = 3.14159
; writing "{:.2f}".format(number)
would result in '3.14'
.
In Python, how do string format specifiers like %s and %i work?
String format specifiers like %s
and %i
are placeholders within a string that get replaced by variables. %s
is used for string values, while %i
is designated for integers. For example, "%s is %i years old." % ('Bob', 25)
would produce 'Bob is 25 years old.'
.
How do you include variables in a string with the .format() method in Python?
To include variables in a string with the .format()
method, you place curly braces {}
in the string where you want the variables to be inserted. The .format()
method then accepts the variables as arguments. Example: age = 30
; 'You are {} years old.'.format(age)
would output 'You are 30 years old.'
.
What are the different ways to concatenate or format strings in Python for output?
In Python, you can concatenate strings with the +
operator, format them using the %
operator, the .format()
method, or with f-strings. Each method provides different levels of control and flexibility for constructing your output.
How can you properly align and pad strings when printing in Python?
To align and pad strings when printing in Python, you can use format specifiers within the .format()
method or f-strings. For example, to align text to the right and pad with spaces, you might use '{:>10}'.format('text')
or in an f-string f'{"text":>10}'
.
January 17, 2024 at 01:10AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
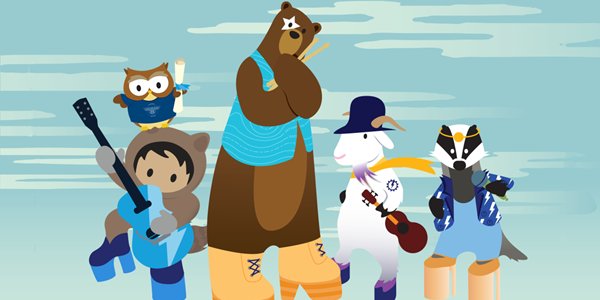
Post a Comment