Python Tuple Concatenation: A Simple Illustrated Guide : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Python tuples are similar to lists, but with a key difference: they are immutable, meaning their elements cannot be changed after creation.
Tuple concatenation means joining multiple tuples into a single tuple. This process maintains the immutability of the tuples, providing a secure and efficient way to combine data. There are several methods for concatenating tuples in Python, such as using the +
operator, the *
operator, or built-in functions like itertools.chain()
.
# Using the + operator to concatenate two tuples tuple1 = (1, 2, 3) tuple2 = (4, 5, 6) concatenated_tuple = tuple1 + tuple2 print("Using +:", concatenated_tuple) # Output: (1, 2, 3, 4, 5, 6) # Using the * operator to repeat a tuple repeated_tuple = tuple1 * 3 print("Using *:", repeated_tuple) # Output: (1, 2, 3, 1, 2, 3, 1, 2, 3) # Using itertools.chain() to concatenate multiple tuples import itertools tuple3 = (7, 8, 9) chained_tuple = tuple(itertools.chain(tuple1, tuple2, tuple3)) print("Using itertools.chain():", chained_tuple) # Output: (1, 2, 3, 4, 5, 6, 7, 8, 9)
The +
operator is used to join two tuples, the *
operator is used to repeat a tuple, and the itertools.chain()
function is used to concatenate multiple tuples. All these methods maintain the immutability of the tuples
Understanding Tuples
Python tuple is a fundamental data type, serving as a collection of ordered, immutable elements. Tuples are used to group multiple data items together. Tuples are created using parentheses
()
and elements within the tuple are separated by commas.
For example, you can create a tuple as follows:
my_tuple = (1, 2, 3, 4, 'example')
In this case, the tuple my_tuple
has five elements, including integers and a string. Python allows you to store values of different data types within a tuple.
Immutable means that tuples cannot be changed once defined, unlike lists. This immutability makes tuples faster and more memory-efficient compared to lists, as they require less overhead to store and maintain element values.
Being an ordered data type means that the elements within a tuple have a definite position or order in which they appear, and this order is preserved throughout the tuple’s lifetime.
Recommended: Python Tuple Data Type
Tuple Concatenation Basics

One common operation performed on tuples is tuple concatenation, which involves combining two or more tuples into a single tuple. This section will discuss the basics of tuple concatenation using the +
operator and provide examples to demonstrate the concept.
Using the + Operator
The +
operator is a simple and straightforward way to concatenate two tuples. When using the +
operator, the two tuples are combined into a single tuple without modifying the original tuples. This is particularly useful when you need to merge values from different sources or create a larger tuple from smaller ones.
Here’s the basic syntax for using the +
operator:
new_tuple = tuple1 + tuple2
new_tuple
will be a tuple containing all elements of tuple1
followed by elements of tuple2
. It’s essential to note that since tuples are immutable, the original tuple1
and tuple2
remain unchanged after the concatenation.
Examples of Tuple Concatenation
Let’s take a look at a few examples to better understand tuple concatenation using the +
operator:
tuple1 = (1, 2, 3) tuple2 = (4, 5, 6) # Concatenate the tuples tuple3 = tuple1 + tuple2 print(tuple3) # Output: (1, 2, 3, 4, 5, 6)
In this example, we concatenated tuple1
and tuple2
to create a new tuple called tuple3
. Notice that the elements are ordered, and tuple3
contains all the elements from tuple1
followed by the elements of tuple2
.
Here’s another example with tuples containing different data types:
tuple1 = ("John", "Doe") tuple2 = (25, "New York") # Concatenate the tuples combined_tuple = tuple1 + tuple2 print(combined_tuple) # Output: ('John', 'Doe', 25, 'New York')
In this case, we combined a tuple containing strings with a tuple containing an integer and a string, resulting in a new tuple containing all elements in the correct order.
Using the * Operator
The *
operator can be used for replicating a tuple a specified number of times and then concatenating the results. This method can be particularly useful when you need to create a new tuple by repeating an existing one.
Here’s an example:
original_tuple = (1, 2, 3) replicated_tuple = original_tuple * 3 print(replicated_tuple) # Output: (1, 2, 3, 1, 2, 3, 1, 2, 3)
In the example above, the original tuple is repeated three times and then concatenated to create the replicated_tuple
. Note that using the *
operator with non-integer values will result in a TypeError
.
Using itertools.chain()
The itertools.chain()
function from the itertools
module provides another way to concatenate tuples. This function takes multiple tuples as input and returns an iterator that sequentially combines the elements of the input tuples.
Here’s an illustration of using itertools.chain()
:
import itertools tuple1 = (1, 2, 3) tuple2 = (4, 5, 6) concatenated_tuple = tuple(itertools.chain(tuple1, tuple2)) print(concatenated_tuple) # Output: (1, 2, 3, 4, 5, 6)
In this example, the itertools.chain()
function is used to combine tuple1
and tuple2
. The resulting iterator is then explicitly converted back to a tuple using the tuple()
constructor.
It’s important to note that itertools.chain()
can handle an arbitrary number of input tuples, making it a flexible option for concatenating multiple tuples:
tuple3 = (7, 8, 9) result = tuple(itertools.chain(tuple1, tuple2, tuple3)) print(result) # Output: (1, 2, 3, 4, 5, 6, 7, 8, 9)
Both the *
operator and itertools.chain()
offer efficient ways to concatenate tuples in Python.
Manipulating Tuples

Tuples are immutable data structures in Python, which means their content cannot be changed once created. However, there are still ways to manipulate and extract information from them.
Slicing Tuples
Slicing is a technique for extracting a range of elements from a tuple. It uses brackets and colons to specify the start, end, and step if needed. The start index is inclusive, while the end index is exclusive.
my_tuple = (0, 1, 2, 3, 4) sliced_tuple = my_tuple[1:4] # This will return (1, 2, 3)
You can also use negative indexes, which count backward from the end of the tuple:
sliced_tuple = my_tuple[-3:-1] # This will return (2, 3)
Tuple Indexing
Tuple indexing allows you to access a specific element in the tuple using its position (index).
my_tuple = ('apple', 'banana', 'cherry') item = my_tuple[1] # This will return 'banana'
An IndexError
will be raised if you attempt to access an index that does not exist within the tuple.
Adding and Deleting Elements
Since tuples are immutable, you cannot directly add or delete elements. However, you can work around this limitation by:
- Concatenating tuples: You can merge two tuples by using the
+
operator.
tuple1 = (1, 2, 3) tuple2 = (4, 5, 6) combined_tuple = tuple1 + tuple2 # This will return (1, 2, 3, 4, 5, 6)
- Converting to a list: If you need to perform several operations that involve adding or removing elements, you can convert the tuple to a list. Once the operations are completed, you can convert the list back to a tuple.
my_tuple = (1, 2, 3) my_list = list(my_tuple) my_list.append(4) # Adding an element my_list.remove(2) # Removing an element new_tuple = tuple(my_list) # This will return (1, 3, 4)
Remember that manipulating tuples in these ways creates new tuples and does not change the original ones.
Common Errors and Solutions

One common error that users might encounter while working with tuple concatenation in Python is the TypeError
. This error can occur when attempting to concatenate a tuple with a different data type, such as an integer or a list.
>>> (1, 2, 3) + 1 Traceback (most recent call last): File "<pyshell#2>", line 1, in <module> (1, 2, 3) + 1 TypeError: can only concatenate tuple (not "int") to tuple
To overcome this issue, make sure to convert the non-tuple object into a tuple before performing the concatenation.
For example, if you’re trying to concatenate a tuple with a list, you can use the tuple()
function to convert the list into a tuple:
tuple1 = (1, 2, 3) list1 = [4, 5, 6] concatenated_tuple = tuple1 + tuple(list1)
Another common error related to tuple concatenation is the AttributeError
. This error might arise when attempting to call a non-existent method or attribute on a tuple. Since tuples are immutable, they don’t have methods like append()
or extend()
that allow addition of elements.
Instead, you can concatenate two tuples directly using the +
operator:
tuple1 = (1, 2, 3) tuple2 = (4, 5, 6) concatenated_tuple = tuple1 + tuple2
When working with nested tuples, ensure proper syntax and data structure handling to avoid errors like ValueError
and TypeError
. To efficiently concatenate nested tuples, consider using the itertools.chain()
function provided by the itertools
module.
This function helps to flatten the nested tuples before concatenation:
import itertools nested_tuple1 = ((1, 2), (3, 4)) nested_tuple2 = ((5, 6), (7, 8)) flattened_tuple1 = tuple(itertools.chain(*nested_tuple1)) flattened_tuple2 = tuple(itertools.chain(*nested_tuple2)) concatenated_tuple = flattened_tuple1 + flattened_tuple2
Frequently Asked Questions
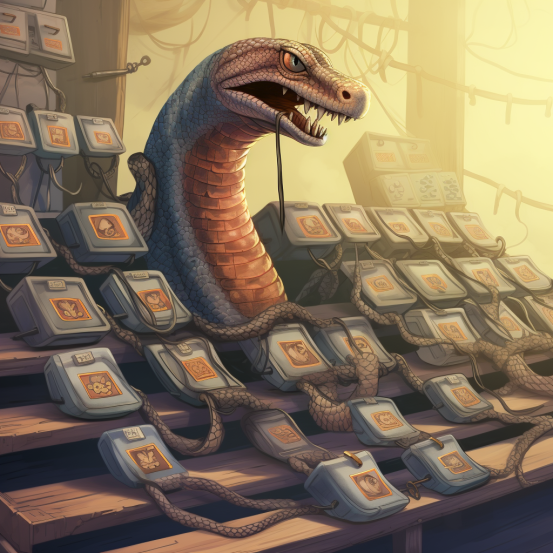
How can I join two tuples?
To join two tuples, simply use the addition +
operator. For example:
tuple_a = (1, 2, 3) tuple_b = (4, 5, 6) result = tuple_a + tuple_b
The result
variable now contains the concatenated tuple (1, 2, 3, 4, 5, 6)
.
What is the syntax for tuple concatenation?
The syntax for concatenating tuples is straightforward. Just use the +
operator between the two tuples you want to concatenate.
concatenated_tuples = first_tuple + second_tuple
How to concatenate a tuple and a string?
To concatenate a tuple and a string, first convert the string into a tuple containing a single element, and then concatenate the tuples. Here’s an example:
my_tuple = (1, 2, 3) my_string = "hello" concatenated_result = my_tuple + (my_string,)
The concatenated_result
will be (1, 2, 3, 'hello')
.
Is it possible to modify a tuple after creation?
Tuples are immutable, which means they cannot be modified after creation (source). If you need to modify the contents of a collection, consider using a list instead.
How can I combine multiple lists of tuples?
To combine multiple lists of tuples, use a combination of list comprehensions and tuple concatenation. Here is an example:
lists_of_tuples = [ [(1, 2), (3, 4)], [(5, 6), (7, 8)] ] combined_list = [t1 + t2 for lst in lists_of_tuples for t1, t2 in lst]
The combined_list
variable will contain [(1, 2, 3, 4), (5, 6, 7, 8)]
.
Can tuple concatenation be extended to more than two tuples?
Yes, tuple concatenation can be extended to more than two tuples by using the +
operator multiple times. For example:
tuple_a = (1, 2, 3) tuple_b = (4, 5, 6) tuple_c = (7, 8, 9) concatenated_result = tuple_a + tuple_b + tuple_c
This will result in (1, 2, 3, 4, 5, 6, 7, 8, 9)
.
Recommended: Python Programming Tutorial [+Cheat Sheets]
The post Python Tuple Concatenation: A Simple Illustrated Guide appeared first on Be on the Right Side of Change.
August 14, 2023 at 01:09AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
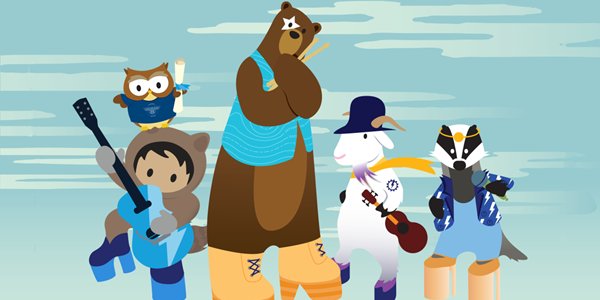
Post a Comment