Python Return Key Value Pair From Function : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Understanding Python Functions and Return Key-Value Pairs
Python functions can return values, which allows you to retrieve information or the result of a computation from the function.
Sometimes, you may want your function to return a key-value pair, a common data structure used in Python dictionaries. Key-value pairs help link pieces of related information, such as item names and their associated values.
To create a function that returns a key-value pair, you can follow this general structure:
def example_function(input_value): # Perform some computation using input_value output_value = input_value * 2 return {input_value: output_value}
In this example, the function takes an input_value
, multiplies it by 2, and returns a dictionary containing a single key-value pair, where the key is the input_value
and the value is the computed output_value
.
Not all functions need to return a value. Some functions, for example, may only perform side effects or write to the console.
To work with key-value pairs effectively in Python, understanding dictionaries and their methods is crucial.
Recommended: Python Dictionary – The Ultimate Guide
Dictionaries have several built-in methods to help you access keys, values, and key-value pairs of the data structure, such as using the items()
method to loop through the items in a dictionary and process each key-value pair.
When you understand how to properly create and use Python functions that return key-value pairs, you can effectively organize and process data, enhancing your code’s versatility and efficiency.
Remember to keep your functions focused on specific tasks, make sure they are “easy to read” and maintain, and always provide informative names for both your functions and variables.
Key-Value Pairs in Dictionary Data Structures
Python dictionaries are versatile data structures that use key-value pairs to store information. Each key maps to a corresponding value, allowing for efficient data retrieval by key search. Keys are unique within the dictionary, while values may not be unique.
Creating a dictionary in Python involves the use of curly braces {}
and separating keys and values with a colon.
For example, initializing a simple dictionary might look like this:
my_dict = { "key1": "value1", "key2": "value2", "key3": "value3" }
To access the value of a specific key, you can use square brackets and the key, like this:
value = my_dict["key1"] # value will be "value1"
Dictionaries also offer several methods to manipulate and access their key-value pairs. The items()
method, for example, returns a view of all the key-value pairs in the dictionary, making it convenient for iterating through them. Here’s an illustration:
for key, value in my_dict.items(): print(key, value)
In some applications, you might need to work with CSV data and convert it to a Python dictionary. This conversion can be helpful in situations requiring more structured data manipulation or easier data access.
Moreover, you may require extracting the first key-value pair of a dictionary or adding multiple values to a single key. Both tasks can be achieved using pre-built Python functions and methods and custom functions depending on the use case.
Dictionaries can also be nested within one another, providing a useful way to represent structured data related to multiple levels of hierarchy. Nested dictionaries can involve more complex operations or traversals, sometimes requiring recursive techniques for dynamic data exploration.
Class Objects and Argument Handling
In Python, functions can accept class objects as arguments and return them as well — instead of key-value pairs. Let’s explore this alternative quickly.
A Python class can be thought of as a blueprint to create objects (instances) with specific characteristics and functionality. Class objects allow the encapsulation of data and methods, providing structure and organization to your code.
To define a class, the class
keyword is used, followed by the class name and a colon to indicate where the class definition begins.
Let’s create a simple Person
class with name
and age
attributes and an introduce
method:
class Person: def __init__(self, name, age): self.name = name self.age = age def introduce(self): print(f"Hi, I'm {self.name} and I'm {self.age} years old.")
The __init__
method, also known as the constructor, is called when an object is created. In this case, it takes two arguments: name
and age
. The keyword self
refers to the current instance of the class and is used to access its attributes and methods.
Now, to create objects using the Person
class, we simply pass the required arguments:
person1 = Person("Alice", 30) person2 = Person("Bob", 25)
Calling the introduce
method on these objects will print a proper introduction:
person1.introduce() person2.introduce()
This would output:
Hi, I'm Alice and I'm 30 years old. Hi, I'm Bob and I'm 25 years old.
In Python, functions can accept class objects as arguments and return them as well. Let’s create a function that takes two Person
objects and returns the one with the greater age:
def older_person(person_a, person_b): if person_a.age > person_b.age: return person_a else: return person_b
We can use this function and display the output like this:
older = older_person(person1, person2) print(f"{older.name} is older.")
This would output:
Alice is older.
Handling class objects and arguments in Python is straightforward and flexible, allowing developers to easily express complex relationships and behaviors in their code.
Working with Tuples in Python
Tuples are an essential data structure in Python, known for their ordered and immutable nature. They can store a sequence of items, which can be of different data types, and are represented by enclosing them within round brackets ( )
, separated by commas.
For example, to create a tuple with integers and strings:
example_tuple = (1, "apple", 3, "banana")
To access individual elements in a tuple, you can use their index. Remember that Python uses zero-based indexing, meaning the first item has an index of 0:
first_item = example_tuple[0] # Output: 1 third_item = example_tuple[2] # Output: 3
Tuples can also have nested tuples, and you can access their elements by using multiple indices:
nested_tuple = (1, (2, 3), (4, (5, 6))) inner_tuple = nested_tuple[1] # Output: (2, 3) nested_element = nested_tuple[2][1][0] # Output: 5
Since tuples are ordered, you can perform sequence operations on them, such as slicing and concatenation:
concatenated_tuple = (1, 2, 3) + (4, 5, 6) # Output: (1, 2, 3, 4, 5, 6) sliced_tuple = concatenated_tuple[1:4] # Output: (2, 3, 4)
Tuples are commonly used to return multiple values from a function. In order to return a key-value pair from a function, you can create a tuple with two elements, where the first element is the key and the second element is the value:
def get_key_value_pair(): return ("key", "value") result = get_key_value_pair() print(result) # Output: ('key', 'value')
To directly unpack the tuple and store the key and value in separate variables, you can use the following syntax:
key, value = get_key_value_pair() print(key) # Output: 'key' print(value) # Output: 'value'
In summary, tuples in Python provide a powerful and flexible way to work with ordered sequences of items. They are particularly useful when returning key-value pairs from functions and can be easily accessed and manipulated using indexing, slicing, and concatenation.
Extracting Items with Items() Method
The items()
method in Python is a valuable tool for working with dictionaries. This method allows you to extract key-value pairs from a Python dictionary, making it easy to access, analyze, and manipulate the data.
The items()
method works on dictionaries and returns a view object consisting of the dictionary’s key-value pairs.
The syntax for using this method is straightforward:
dictionary.items()
Consider the following example of a Python dictionary containing information on various fruits and their associated colors:
fruit_colors = {'apple': 'red', 'banana': 'yellow', 'grape': 'purple'}
To extract the key-value pairs using the items()
method, simply call the method on the dictionary:
fruit_items = fruit_colors.items() print(fruit_items)
The output would be a view object displaying the key-value pairs:
dict_items([('apple', 'red'), ('banana', 'yellow'), ('grape', 'purple')])
Note that the view object received from the items()
method is not a standalone dictionary. Instead, it is a dynamic view that reflects updates to the original dictionary.
To convert the view object back to a dictionary, we can use the dict()
constructor:
fruit_items_dict = dict(fruit_items) print(fruit_items_dict)
This will output the same dictionary we started with:
{'apple': 'red', 'banana': 'yellow', 'grape': 'purple'}
The items()
method is particularly useful when iterating through a dictionary. For example, you can use a for loop to access and display both the keys and values of a dictionary:
for key, value in fruit_colors.items(): print(f"The color of {key} is {value}.")
With this loop, the output would be:
The color of apple is red. The color of banana is yellow. The color of grape is purple.
In summary, the items()
method is a powerful tool in Python for working with dictionaries, providing an efficient way to access and manage key-value pairs.
Error Handling and Exceptions
When working with Python functions that return key-value pairs, you may want to handle errors and exceptions, such as TypeError
and IndexError
.
These may occur when performing operations like setting an array element with a sequence or attempting to access an element using an incorrect index or type. Properly handling these exceptions ensures the robustness of the code.
For instance, consider a function that returns a key-value pair from a dictionary. It is necessary to handle KeyError
, which occurs when a non-existent key is accessed from the dictionary. Additionally, a TypeError
may occur when a key or type is invalid, such as when the 'float' object is not subscriptable
.
Here’s an example of a function that handles these exceptions:
def get_key_value_pair(dictionary, key): try: value = dictionary[key] return key, value except KeyError: print("KeyError: The specified key does not exist in the dictionary.") except TypeError: print("TypeError: The specified key or type is invalid.")
In the above code, we use try
and except
blocks to catch and handle KeyError
and TypeError
. Understanding how to catch and print exception messages in Python will enable you to debug your code efficiently.
Another common error that may occur is the IndexError
, which is raised when attempting to access an array element outside its valid range.
To handle this exception, include an additional except
block, as shown below:
def get_key_value_pair(dictionary, key): try: value = dictionary[key] return key, value except KeyError: print("KeyError: The specified key does not exist in the dictionary.") except TypeError: print("TypeError: The specified key or type is invalid.") except IndexError: print("IndexError: The specified index is out of bounds.")
Frequently Asked Questions
How do you extract a key-value pair in Python?
To extract a key-value pair from a dictionary in Python, you can use a loop or a list comprehension. Here’s an example using a for
loop:
my_dict = {'a': 1, 'b': 2, 'c': 3} for key, value in my_dict.items(): print(f'Key: {key}, Value: {value}')
Which function in Python will return a key-value pair of a dictionary?
The items()
function is commonly used in Python to return key-value pairs of a dictionary. Here’s an example:
my_dict = {'name': 'John', 'age': 30} key_value_pairs = my_dict.items() print(key_value_pairs) # Output: dict_items([('name', 'John'), ('age', 30)])
How to return value if key exists in Python?
You can use the get()
method to return a value if the key exists in a Python dictionary, and optionally provide a default value if the key is not present. Example:
my_dict = {'name': 'John', 'age': 30} value = my_dict.get('name', 'Not found') print(value) # Output: John value = my_dict.get('job', 'Not found') print(value) # Output: Not found
How do I separate key and value in a Python dictionary?
To separate keys and values in a dictionary, you can use the keys()
and values()
methods. Here’s an example:
my_dict = {'a': 1, 'b': 2, 'c': 3} keys = my_dict.keys() values = my_dict.values() print(f'Keys: {list(keys)}') # Output: Keys: ['a', 'b', 'c'] print(f'Values: {list(values)}') # Output: Values: [1, 2, 3]
How to get index of value in dictionary using Python?
Dictionaries do not have a concept of index like lists. However, you can find the key associated with a given value. Here’s an example to find the key for a given value:
my_dict = {'a': 1, 'b': 2, 'c': 3} value_to_find = 2 key = next((k for k, v in my_dict.items() if v == value_to_find), None) print(f'Key for value {value_to_find}: {key}') # Output: Key for value 2: b
Extracting key-value pairs from a string in Python
You can use regular expressions or string parsing techniques to extract key-value pairs from a string. Here’s an example using regular expressions:
import re input_str = "name=John;age=30" pattern = re.compile(r'(\w+)=([\w\d]+)') matches = pattern.findall(input_str) key_value_pairs = dict(matches) print(key_value_pairs) # Output: {'name': 'John', 'age': '30'}
In this example, the re
module’s findall()
function extracts key-value pairs from the given input string.
July 25, 2023 at 06:52PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
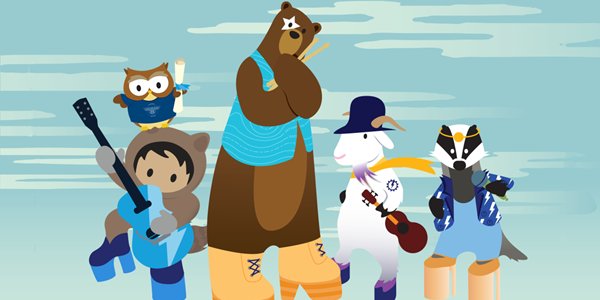
Post a Comment