Python Return Context Manager From Function : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Understanding Context Managers in Python
Context managers in Python are a convenient and efficient way to manage resources such as file handles, sockets, and database connections. They ensure that the resources are acquired and released properly, reducing the chance of resource leaks.
The with
statement is used in conjunction with context managers to ensure that the resource is utilized and released within the indented block of code.
In this section, we will explore class-based and function-based context managers.
Class-Based Context Managers
Class-based context managers are created by defining a class with __enter__
and __exit__
methods.
- The
__enter__
method is called when thewith
statement is executed. It returns the resource object that will be managed. - The
__exit__
method is called when the block of code within thewith
statement is done executing, and it takes care of closing, releasing, or disposing of the resource.
Here’s a simple example of a class-based context manager for file handling:
class FileHandler: def __init__(self, file_name, mode): self.file_name = file_name self.mode = mode def __enter__(self): self.file = open(self.file_name, self.mode) return self.file def __exit__(self, exc_type, exc_val, exc_tb): self.file.close() with FileHandler("test.txt", "r") as file: print(file.readline())
In the example above, the FileHandler
class defines __enter__
and __exit__
methods to manage the opening and closing of a file. The with
statement ensures that the __exit__
method is always called, even if an exception is raised within the block of code.
Function-Based Context Managers
Function-based context managers are created using the contextlib
module’s contextmanager
decorator.
A decorated function should contain a yield
statement, which returns the resource object.
The code before the yield
statement is executed when the with
statement is entered, and the code after the yield
statement is executed when the block of code within the with
statement is done.
Here’s an example of a function-based context manager for file handling:
from contextlib import contextmanager @contextmanager def file_handler(file_name, mode): file = open(file_name, mode) try: yield file finally: file.close() with file_handler("test.txt", "r") as file: print(file.readline())
In this example, the file_handler
function is decorated with the @contextmanager
decorator. The code before the yield
statement opens the file, and the code within the finally
block ensures that the file is closed when the with
statement is exited, regardless of whether an exception occurred or not.
Both class-based and function-based context managers provide a clean and efficient way to manage resources in Python. By using the with
statement, you can ensure that your resources are acquired and released properly, preventing resource leaks and making your code more readable and maintainable.
Implementing Class-Based Context Managers
Class-based context managers make resource and context management in Python easier by implementing two essential methods: __enter__()
and __exit__()
. Through these methods, the class adheres to the context manager protocol and can be used with the with
statement.
Defining the __enter__() Method
The __enter__()
method is called when the with
statement is executed. It performs any required setup or resource allocation. The method can also return an object, which is assigned to a specified variable after the as
keyword in the with
statement.
Here is an example:
class MyContextManager: def __enter__(self): print("Entering the context!") return self with MyContextManager() as cm: print("Inside the context!")
When the code is executed, it outputs:
Entering the context! Inside the context!
The MyContextManager
object is returned by the __enter__()
method and assigned to the cm
variable.
Defining the __exit___() Method
The __exit__()
method is responsible for cleaning up resources or performing teardown actions. It is called when the with
block is exited, whether through an exception or normal execution.
The __exit__()
method receives three arguments: exc_type
, exc_value
, and exc_traceback
. These are used to pass exception information if an exception was raised within the with
block.
Here’s an example of a class-based context manager that utilizes the __exit__()
method:
class MyContextManager: def __enter__(self): print("Entering the context!") return self def __exit__(self, exc_type, exc_value, exc_traceback): print("Exiting the context!") if exc_type: print(f"An {exc_type} exception occurred with value: {exc_value}") with MyContextManager(): print("Inside the context!") raise ValueError("An example exception")
The output will be:
Entering the context! Inside the context! Exiting the context! An <class 'ValueError'> exception occurred with value: An example exception
The __exit__()
method is called when exiting the context, and if an exception occurs, it’s handled and logged, depending on the need.
In summary, by implementing the __enter__()
and __exit__()
methods, a class can adhere to the context manager protocol in Python, making resource management and context handling more efficient and readable.
Function-Based Context Managers with Decorators
Using contextlib.contextmanager
The contextlib
module in Python provides a convenient tool for creating function-based context managers. By using the @contextmanager
decorator, you can create context managers with a more lightweight syntax.
The basic structure of a function-based context manager using the @contextmanager
decorator involves defining a function that yield
s a result:
from contextlib import contextmanager @contextmanager def my_context_manager(): # Setup code here try: yield result finally: # Cleanup code here
In this example, the setup code runs before the yield
statement, and the cleanup code runs after the yield
statement. The yield
statement produces the resource or result that will be managed by the context manager.
Implementing a Function as a Context Manager
To create a function that works both as a decorator and a context manager, you can utilize the functools.wraps
function to preserve metadata and define the necessary methods, such as __enter__()
and __exit__()
.
Here’s an example of a function-based context manager that measures the execution time of a block of code:
import time from contextlib import contextmanager @contextmanager def timing(): start_time = time.time() try: yield finally: end_time = time.time() elapsed_time = end_time - start_time print(f"Elapsed time: {elapsed_time} seconds")
To use this context manager, you can simply use the with
statement:
with timing(): # Your code here
This context manager will measure the execution time of the code within the with
block and print the result at the end.
Creating Custom Context Managers
In Python, managing resources such as files, sockets, and database connections can be efficiently done with context managers.
This section will cover creating custom context managers with class-based and function-based approaches, focusing on resource management, cleanup code, and exception handling.
Resource Management and Cleanup Code
To create a custom context manager, you need to implement the __enter__()
and __exit__()
methods of a class.
- The
__enter__()
method is executed when entering thewith
statement, and it takes care of the resource initialization. - The
__exit__()
method is automatically called when exiting thewith
statement, handling the cleanup process.
Here’s an example of a class-based context manager for a file resource:
class CustomFile: def __init__(self, file_name, mode): self.file_name = file_name self.mode = mode def __enter__(self): self.file = open(self.file_name, self.mode) return self.file def __exit__(self, exc_type, exc_val, exc_tb): self.file.close()
Usage example:
with CustomFile('test.txt', 'r') as file: content = file.read() print(content)
Alternatively, you can use the contextlib
module to create a function-based context manager by decorating the function with the @contextmanager
decorator.
from contextlib import contextmanager @contextmanager def custom_file(file_name, mode): file = open(file_name, mode) try: yield file finally: file.close()
Usage remains the same:
with custom_file('test.txt', 'r') as file: content = file.read() print(content)
Exception Handling in Context Managers
Exception handling within context managers is important to ensure that resources are cleaned up even when errors occur. Caught exceptions can be logged, handled, or propagated based on the desired behavior.
In class-based context managers, the __exit__()
method takes three arguments: exc_type
, exc_val
, and exc_tb
. These represent the exception type, value, and traceback, respectively. To propagate the exception, return False
; to suppress it, return True
.
class CustomFileWithLogging: # ... (same as CustomFile) def __exit__(self, exc_type, exc_val, exc_tb): self.file.close() if exc_type is not None: print(f"An error occurred: {exc_val}") return False # Propagate the exception
In function-based context managers, you can use a try
–except
–finally
block within the with
body.
@contextmanager def custom_file_with_logging(file_name, mode): file = open(file_name, mode) try: yield file except Exception as e: print(f"An error occurred: {e}") raise finally: file.close()
Working with File Management and Context Managers
File Objects and the with Statement
In Python, file objects are a common resource to manage. They represent a file on the system and provide methods to interact with the file (read, write, etc.).
The with
statement is used to create a context manager for resources, like file objects. A context manager is an object that defines methods to set up a context (__enter__()
), and to perform cleanup (__exit__()
), when no longer needed.
Using the with
statement, the file object is automatically closed when the block of code under it is finished executing, even if an exception occurs within the block. Here’s an example of how the with
statement is used with file objects:
with open('example.txt', 'r') as file: content = file.read() print(content)
Open() Function and Context Managers
The built-in open()
function in Python is used to open a file and returns a file object. It takes two arguments — the file name (path) and the file access mode (read, write, append). The open()
function is compatible with the with
statement, providing a context manager for file objects.
Using open()
with a context manager ensures that the file object is closed automatically at the end of the with
block. This is a more elegant and safer way to work with files, as it avoids the need to call the file.close()
method explicitly.
Here’s a code example using the open()
function and a context manager to write data to a file:
with open('output.txt', 'w') as file: file.write('Hello, World!')
In this example, the output.txt
file is opened in write mode ('w'
). When the with
block is done, the file object is closed automatically, ensuring that the resources are properly managed.
Testing and Mocking Context Managers
In this section, we will discuss how to test and mock context managers in Python using pytest
and patch
. Testing context managers helps ensure that your code is robust and reliable, especially when it comes to managing external resources such as files or database connections.
Using pytest
pytest
is a popular testing framework that simplifies writing and running tests in Python. It comes with built-in support for testing context managers using the with
statement.
To demonstrate this, let’s use a simple context manager that prints a message when entering and exiting:
from contextlib import contextmanager @contextmanager def example_manager(): print("Entering the context") yield print("Exiting the context")
Now, let’s write a test for this context manager using pytest
:
import pytest from example_module import example_manager def test_example_manager(capfd): with example_manager(): pass out, err = capfd.readouterr() assert out == "Entering the context\nExiting the context\n"
Here, we’re using the capfd
fixture provided by pytest
to capture standard output and assert that the expected messages are printed.
Patching Context Managers
Sometimes, you may want to test code that uses context managers without actually invoking the original behavior of the context manager. This is where patch
from the unittest.mock
library comes in handy. It allows you to replace the context manager with a mock object during tests.
To demonstrate this, let’s say we have a function that uses the example_manager
context manager as follows:
def function_using_manager(): with example_manager(): print("Inside the context")
We can write a test using patch
to replace the example_manager
context manager with a mock, like so:
from unittest.mock import patch from example_module import function_using_manager def test_function_using_manager(capfd): with patch("example_module.example_manager") as mock_manager: mock_manager.__enter__.return_value = None function_using_manager() mock_manager.__exit__.assert_called_once() out, err = capfd.readouterr() assert out == "Inside the context\n"
In this test, we are patching the example_manager
context manager and configuring its __enter__
method to return None
. We then call the function_using_manager
function and assert that the __exit__
method of the mocked context manager is called once. Additionally, we use capfd
once again to ensure that the “Inside the context” message is printed as expected.
Advanced Context Management
In this section, we will discuss advanced techniques in context management, including Async Context Managers and creating Context Manager Factories, which provide more flexible and powerful ways to manage resources in your Python code.
Async Context Managers
Async context managers, introduced in PEP 343, allow for asynchronous resource management using the async with
statement. This is particularly useful when dealing with I/O operations or tasks that can benefit from non-blocking execution.
Async context managers implement the __aenter__()
and __aexit__()
methods instead of the standard __enter__()
and __exit__()
methods in the context manager protocol.
Here’s an example of using an async context manager with an async function:
import aiofile async def read_file(file_path): async with aiofile.AIOFile(file_path, 'r') as file: contents = await file.read() print(contents) await read_file("file.txt")
In this example, aiofile.AIOFile
is an async context manager, and we use the async with
statement to handle file operations in a non-blocking manner.
Creating Context Manager Factories
A context manager factory is a function that returns a context manager, enabling more dynamic and customizable resource management. The contextlib
module in the Python standard library provides helpful tools for creating context manager factories.
One example is the contextlib.contextmanager
decorator, which allows you to define a single generator function that yields a value, effectively splitting the function into its setup and teardown parts.
Here’s an example of creating a context manager factory using contextlib.contextmanager
:
from contextlib import contextmanager @contextmanager def managed_resource(*args, **kwargs): resource = allocate_resource(*args, **kwargs) try: yield resource finally: release_resource(resource) with managed_resource(arguments) as resource: # Use the resource here pass
In this example, allocate_resource()
and release_resource()
are functions that handle the acquisition and release of a specific resource. The managed_resource()
function is a context manager factory that allows you to use the with
statement to manage resources defined by these functions.
Frequently Asked Questions
How to create a custom context manager using a function?
To create a custom context manager using a function, you can use the contextlib.contextmanager
decorator. The function should be defined as a generator, using the yield
statement to separate the __enter__()
and __exit__()
methods.
Here’s an example:
from contextlib import contextmanager @contextmanager def my_context_manager(): print("Entering the context") yield print("Exiting the context") with my_context_manager(): print("Inside the context")
What is the role of ‘yield’ in a context manager?
In a context manager implemented with a generator function, the yield
statement serves as the separator between the __enter__()
and __exit__()
methods. When the with
statement is encountered, the code before the yield
is executed as part of the __enter__()
method. After the yield
, the code is executed as the __exit__()
method when the context is left.
How can I use ‘contextlib’ to create a context manager?
contextlib
is a Python library that provides utilities for creating and working with context managers. You can use the contextlib.contextmanager
decorator to define a context manager function without needing to create a separate class. Remember, the function must be a generator.
Here’s an example:
from contextlib import contextmanager @contextmanager def my_context_manager(): print("Entering the context") yield print("Exiting the context") with my_context_manager(): print("Inside the context")
What are some use cases of nested context managers?
Nested context managers are useful when you need to manage multiple resources simultaneously, such as opening multiple files or acquiring several locks. They allow you to ensure that each resource is properly acquired and released.
Here’s an example with nested file opening:
with open("file1.txt") as file1: with open("file2.txt") as file2: # Perform operations with both file1 and file2 pass
Can I create a decorator for my context manager?
Yes, you can create a decorator for your context manager. This involves using the contextlib.contextmanager
decorator and defining your context manager function.
Here’s an example of a context manager serving as a decorator:
from contextlib import contextmanager @contextmanager def decorate(): print("Before the function call") yield print("After the function call") @decorate() def my_function(): print("Inside the function") my_function()
How do I implement an async context manager?
To create an async context manager, you can define an asynchronous class with __aenter__()
and __aexit__()
methods or use the asyncio
library and the contextlib.asynccontextmanager
decorator in combination with an asynchronous generator function.
Here’s an example using the decorator:
import asyncio from contextlib import asynccontextmanager @asynccontextmanager async def my_async_context_manager(): print("Entering the async context") yield print("Exiting the async context") async def main(): async with my_async_context_manager(): print("Inside the async context") asyncio.run(main())
Recommended: 6 Easiest Ways to Get Started with Llama2: Meta’s Open AI Model
July 28, 2023 at 05:02PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
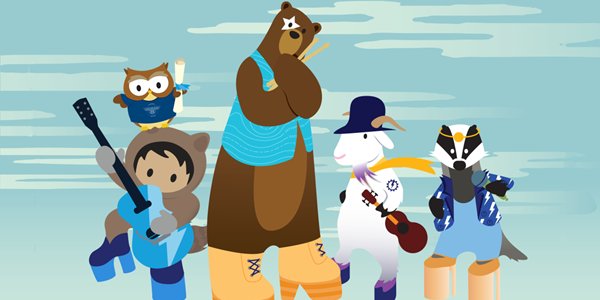
Post a Comment