What Does if __name__ == "__main__" Do in Python?
by:
blow post content copied from Real Python
click here to view original post
You’ve likely encountered Python’s if __name__ == "__main__"
idiom when reading other people’s code. No wonder—it’s widespread! You might have even used if __name__ == "__main__"
in your own scripts. But did you use it correctly?
Maybe you’ve programmed in a C-family language like Java before, and you wonder whether this construct is a clumsy accessory to using a main()
function as an entry point.
Syntactically, Python’s if __name__ == "__main__"
idiom is just a normal conditional block:
1if __name__ == "__main__":
2 ...
The indented block starting in line 2 contains all the code that Python will execute when the conditional statement in line 1 evaluates to True
. In the code example above, the specific code logic that you’d put in the conditional block is represented with a placeholder ellipsis (...
).
So—if there’s nothing special about the if __name__ == "__main__"
idiom, then why does it look confusing, and why does it continue to spark discussion in the Python community?
If the idiom still seems a little cryptic, and you’re not completely sure what it does, why you might want it, and when to use it, then you’ve come to the right place! In this tutorial, you’ll learn all about Python’s if __name__ == "__main__"
idiom—starting with what it really does in Python, and ending with a suggestion for a quicker way to refer to it.
Source Code: Click here to download the free source code that you’ll use to understand the name-main idiom.
In Short: It Allows You to Execute Code When the File Runs as a Script, but Not When It’s Imported as a Module
For most practical purposes, you can think of the conditional block that you open with if __name__ == "__main__"
as a way to store code that should only run when your file is executed as a script.
You’ll see what that means in a moment. For now, say you have the following file:
1# echo.py
2
3def echo(text: str, repetitions: int = 3) -> str:
4 """Imitate a real-world echo."""
5 echoed_text = ""
6 for i in range(repetitions, 0, -1):
7 echoed_text += f"{text[-i:]}\n"
8 return f"{echoed_text.lower()}."
9
10if __name__ == "__main__":
11 text = input("Yell something at a mountain: ")
12 print(echo(text))
In this example, you define a function, echo()
, that mimics a real-world echo by gradually printing fewer and fewer of the final letters of the input text.
Below that, in lines 10 to 12, you use the if __name__ == "__main__"
idiom. This code starts with the conditional statement if __name__ == "__main__"
in line 10. In the indented lines, 11 and 12, you then collect user input and call echo()
with that input. These two lines will execute when you run echo.py
as a script from your command line:
$ python echo.py
Yell something at a mountain: HELLOOOO ECHOOOOOOOOOO
ooo
oo
o
.
When you run the file as a script by passing the file object to your Python interpreter, the expression __name__ == "__main__"
returns True
. The code block under if
then runs, so Python collects user input and calls echo()
.
Try it out yourself! You can download all the code files that you’ll use in this tutorial from the link below:
Source Code: Click here to download the free source code that you’ll use to understand the name-main idiom.
At the same time, if you import echo()
in another module or a console session, then the nested code won’t run:
>>> from echo import echo
>>> print(echo("Please help me I'm stuck on a mountain"))
ain
in
n
.
In this case, you want to use echo()
in the context of another script or interpreter session, so you won’t need to collect user input. Running input()
would mess with your code by producing a side effect when importing echo
.
When you nest the code that’s specific to the script usage of your file under the if __name__ == "__main__"
idiom, then you avoid running code that’s irrelevant for imported modules.
Nesting code under if __name__ == "__main__"
allows you to cater to different use cases:
- Script: When run as a script, your code prompts the user for input, calls
echo()
, and prints the result. - Module: When you import
echo
as a module, thenecho()
gets defined, but no code executes. You provideecho()
to the main code session without any side effects.
By implementing the if __name__ == "__main__"
idiom in your code, you set up an additional entry point that allows you to use echo()
right from the command line.
Read the full article at https://realpython.com/if-name-main-python/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
September 21, 2022 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
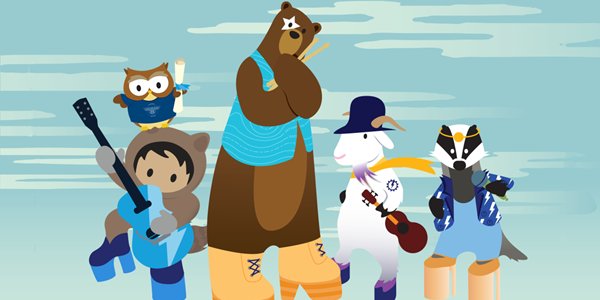
Post a Comment