6 Best Ways to Check the Package Version in Python : Chris
by: Chris
blow post content copied from Finxter
click here to view original post
In this article, I’ll show you how to check the version of a Python module (package, library).
These are the six best ways to check the version of a Python module:
- Method 1:
pip show your_package
- Method 2:
pip list
- Method 3:
your_package.__version__
- Method 4:
importlib.metadata.version
- Method 5:
conda list
- Method 6:
pip freeze
Let’s dive into some examples for each of those next!
Method 1: pip show
To check which version of a given Python library, say xyz
, is installed, use pip show xyz
or pip3 show xyz
. For example, to check the version of your NumPy installation, run pip show numpy
in your CMD/Powershell (Windows), or terminal (macOS/Linux/Ubuntu).
This will work if your pip installation is version 1.3 or higher—which is likely to hold in your case because pip 1.3 was released a decade ago in 2013!!
Here’s an example in my Windows Powershell for NumPy: I’ve highlighted the line that shows that my package version is 1.21.0
:
PS C:\Users\xcent> pip show numpy Name: numpy Version: 1.21.0 Summary: NumPy is the fundamental package for array computing with Python. Home-page: https://www.numpy.org Author: Travis E. Oliphant et al. Author-email: None License: BSD Location: c:\users\xcent\appdata\local\programs\python\python39\lib\site-packages Requires: Required-by: pandas, matplotlib
In some instances, this will not work—depending on your environment. In this case, try those commands before giving up:
python -m pip show numpy python3 -m pip show numpy py -m pip show numpy pip3 show numpy
Of course, replace “numpy
” with your particular package name.
Method 2: pip list
To check the versions of all installed packages, use pip list
and locate the version of your particular package in the output list of package versions sorted alphabetically.
This will work if your pip installation is version 1.3 or higher.
Here’s an example in my Windows Powershell, I’ve highlighted the line that shows that my package version is 1.21.0:
PS C:\Users\xcent> pip list Package Version --------------- --------- beautifulsoup4 4.9.3 bs4 0.0.1 certifi 2021.5.30 chardet 4.0.0 cycler 0.10.0 idna 2.10 kiwisolver 1.3.1 matplotlib 3.4.2 mss 6.1.0 numpy 1.21.0 pandas 1.3.1 Pillow 8.3.0 pip 21.1.1 pyparsing 2.4.7 python-dateutil 2.8.1 pytz 2021.1 requests 2.25.1 setuptools 56.0.0 six 1.16.0 soupsieve 2.2.1 urllib3 1.26.6
In some instances, this will not work—depending on your environment. Then try those commands before giving up:
python -m pip list python3 -m pip list py -m pip list pip3 list
Method 3: Library.__version__ Attribute
To check your package installation in your Python script, you can also use the xyz.__version__
attribute of the particular library xyz
. Not all packages provide this attribute but as it is recommended by PEP, it’ll work for most libraries.
Here’s the code:
import numpy print(numpy.__version__) # 1.21.0
Here’s an excerpt from the PEP 8 docs mentioning the __version__
attribute.
“PEP 8 describes the use of a module attribute called __version__
for recording “Subversion, CVS, or RCS” version strings using keyword expansion. In the PEP author’s own email archives, the earliest example of the use of an __version__
module attribute by independent module developers dates back to 1995.”
Method 4: importlib.metadata.version
The importlib.metadata
library provides a general way to check the package version in your Python script via importlib.metadata.version('xyz')
for library xyz
. This returns a string representation of the specific version. For example, importlib.metadata.version('numpy')
returns 1.21.0
in my current environment.
Here’s the code:
import importlib.metadata print(importlib.metadata.version('numpy')) # 1.21.0
Method 5: conda list
If you have created your Python environment with Anaconda, you can use conda list
to list all packages installed in your (virtual) environment. Optionally, you can add a regular expression using the syntax conda list regex to list only packages matching a certain pattern.
How to list all packages in the current environment?
conda list
How to list all packages installed into the environment 'xyz'
?
conda list -n xyz
Regex: How to list all packages starting with 'py'
?
conda list '^py'
Regex: How to list all packages starting with 'py'
or 'code'
?
conda list '^(py|code)'
Method 6: pip freeze
The pip freeze
command without any option lists all installed Python packages in your environment in alphabetically order (ignoring UPPERCASE or lowercase). You can spot your specific package if it is installed in the environment.
pip freeze
Output from my local Windows environment with PowerShell (strange packages I know) ;):
PS C:\Users\xcent> pip freeze asn1crypto==1.5.1 et-xmlfile==1.1.0 openpyxl==3.0.10
For example, I have the Python package openpyxl
installed with version 3.0.10
.
You can modify or exclude specific packages using the options provided in this screenshot:
Summary
In this article, you’ve learned six ways to check a Python package version:
- Method 1:
pip show your_package
- Method 2:
pip list
- Method 3:
your_package.__version__
- Method 4:
importlib.metadata.version
- Method 5:
conda list
- Method 6:
pip freeze
Thanks for giving us your valued attention — we’re grateful to have you here!
Programmer Humor
There are only 10 kinds of people in this world: those who know binary and those who don’t.
~~~
There are 10 types of people in the world. Those who understand trinary, those who don’t, and those who mistake it for binary.
July 13, 2022 at 02:53PM
Click here for more details...
=============================
The original post is available in Finxter by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
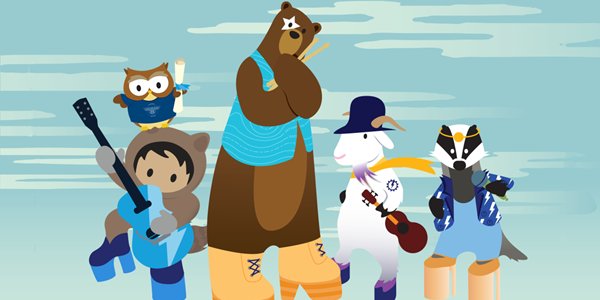
Post a Comment