Asynchronous Iterators and Iterables in Python :
by:
blow post content copied from Real Python
click here to view original post
When you write asynchronous code in Python, you’ll likely need to create asynchronous iterators and iterables at some point. Asynchronous iterators are what Python uses to control async for
loops, while asynchronous iterables are objects that you can iterate over using async for
loops.
Both tools allow you to iterate over awaitable objects without blocking your code. This way, you can perform different tasks asynchronously.
In this tutorial, you’ll:
- Learn what async iterators and iterables are in Python
- Create async generator expressions and generator iterators
- Code async iterators and iterables with the
.__aiter__()
and.__anext__()
methods - Use async iterators in async loops and comprehensions
To get the most out of this tutorial, you should know the basics of Python’s iterators and iterables. You should also know about Python’s asynchronous features and tools.
Get Your Code: Click here to download the free sample code that you’ll use to learn about asynchronous iterators and iterables in Python.
Take the Quiz: Test your knowledge with our interactive “Asynchronous Iterators and Iterables in Python” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Asynchronous Iterators and Iterables in PythonTake this quiz to test your understanding of how to create and use Python async iterators and iterables in the context of asynchronous code.
Getting to Know Async Iterators and Iterables in Python
Iterators and iterables are fundamental components in Python. You’ll use them in almost all your programs where you iterate over data streams using a for
loop. Iterators power and control the iteration process, while iterables typically hold data that you want to iterate over.
Python iterators implement the iterator design pattern, which allows you to traverse a container and access its elements. To implement this pattern, iterators need the .__iter__()
and .__next__()
special methods. Similarly, iterables are typically data containers that implement the .__iter__()
method.
Note: To dive deeper into iterators and iterables, check out the Iterators and Iterables in Python: Run Efficient Iterations tutorial.
Python has extended the concept of iterators and iterables to asynchronous programming with the asyncio
module and the async
and await
keywords. In this scenario, asynchronous iterators drive the asynchronous iteration process, mainly powered by async for
loops and comprehensions.
Note: In this tutorial, you won’t dive into the intricacies of Python’s asynchronous programming. So, you should be familiar with the related concepts. If you’re not, then you can check out the following tutorials:
In these tutorials, you’ll gain the required background to prepare for exploring asynchronous iterators and iterables in more depth.
In the following sections, you’ll briefly examine the concepts of asynchronous iterators and iterables in Python.
Async Iterators
Python’s documentation defines asynchronous iterators, or async iterators for short, as the following:
An object that implements the
.__aiter__()
and.__anext__()
[special] methods..__anext__()
must return an awaitable object. [An]async for
[loop] resolves the awaitables returned by an asynchronous iterator’s.__anext__()
method until it raises aStopAsyncIteration
exception. (Source)
Similar to regular iterators that must implement .__iter__()
and .__next__()
, async iterators must implement .__aiter__()
and .__anext__()
. In regular iterators, the .__iter__()
method usually returns the iterator itself. This is also true for async iterators.
To continue with this parallelism, in regular iterators, the .__next__()
method must return the next object for the iteration. In async iterators, the .__anext__()
method must return the next object, which must be awaitable.
Python defines awaitable objects as described in the quote below:
An object that can be used in an
await
expression. [It] can be a coroutine or an object with an.__await__()
method. (Source)
In practice, a quick way to make an awaitable object in Python is to call an asynchronous function. You define this type of function with the async def
keyword construct. This call creates a coroutine object.
Note: You can also create awaitable objects by implementing the .__await__()
special method in a custom class. This method must return an iterator that yields control back to the event loop until the awaited result is ready. This topic is beyond the scope of this tutorial.
When the data stream runs out of data, the method must raise a StopAsyncIteration
exception to end the asynchronous iteration process.
Here’s an example of an async iterator that allows iterating over a range of numbers asynchronously:
Read the full article at https://realpython.com/python-async-iterators/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
August 07, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
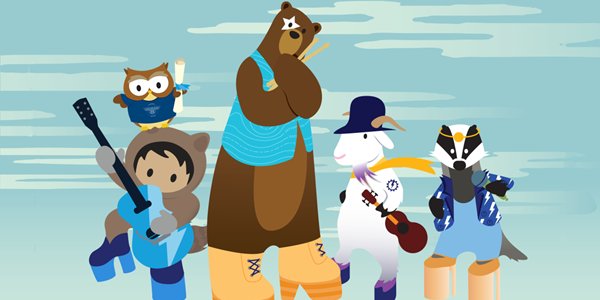
Post a Comment