5 Best Ways to Show a Plotly Animated Slider in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: You want to create interactive visualizations in Python and need to display time series data or sequential information. The goal is to embed an animated slider within a Plotly graph to enable users to dynamically explore data changes over time. For example, your input might be a dataset with sales figures over several years, and your desired output is an animated chart that shows sales trends with a controllable slider.
Method 1: Using Plotly Express
Plotly Express is a high-level interface that simplifies the creation of complex animated plots. To add an animated slider, you can utilize the animation_frame
parameter within Plotly Express functions to define which column in your dataset represents the sequence of frames.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(x=[1, 2, 3], y=[4, 5, 6])], layout=go.Layout( sliders=[{ 'steps': [ {'method': 'animate', 'label': 'Frame 1', 'args': [[0]]}, {'method': 'animate', 'label': 'Frame 2', 'args': [[1]]}, {'method': 'animate', 'label': 'Frame 3', 'args': [[2]]} ] }] ), frames=[go.Frame(data=[go.Scatter(x=[1], y=[4])]), go.Frame(data=[go.Scatter(x=[2], y=[5])]), go.Frame(data=[go.Scatter(x=[3], y=[6])])] ) fig.show()
The output is a simple animated line chart with a custom slider.
This code snippet manually constructs a line chart with frames corresponding to different data points. Sliders are added through the layout object, specifying the method and label for each step of the animation.
Method 3: Adding Play and Pause Buttons
To enhance interactivity, you can include play and pause buttons along with the slider. This provides users with better control over the animation, making it more user-friendly.
Here’s an example:
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.express as px df = px.data.gapminder() fig = px.scatter(df, x="gdpPercap", y="lifeExp", animation_frame="year", size="pop", color="continent", hover_name="country") fig.show()
The output is an interactive scatter plot with a slider representing the years from the dataset.
This code snippet utilizes a built-in dataset from Plotly Express and creates an animated scatter plot. The animation_frame="year"
adds the slider that animates the plot year by year. The resulting graph will update points’ positions, sizes, and colors as the slider moves.
Method 2: Customizing the Slider with Graph Objects
If you require more control over the slider’s appearance and functionality, you can use the lower-level Graph Objects module. You’ll manually create slider objects and define their properties to customize the animation.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(x=[1, 2, 3], y=[4, 5, 6])], layout=go.Layout( sliders=[{ 'steps': [ {'method': 'animate', 'label': 'Frame 1', 'args': [[0]]}, {'method': 'animate', 'label': 'Frame 2', 'args': [[1]]}, {'method': 'animate', 'label': 'Frame 3', 'args': [[2]]} ] }] ), frames=[go.Frame(data=[go.Scatter(x=[1], y=[4])]), go.Frame(data=[go.Scatter(x=[2], y=[5])]), go.Frame(data=[go.Scatter(x=[3], y=[6])])] ) fig.show()
The output is a simple animated line chart with a custom slider.
This code snippet manually constructs a line chart with frames corresponding to different data points. Sliders are added through the layout object, specifying the method and label for each step of the animation.
Method 3: Adding Play and Pause Buttons
To enhance interactivity, you can include play and pause buttons along with the slider. This provides users with better control over the animation, making it more user-friendly.
Here’s an example:
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.express as px df = px.data.gapminder() fig = px.scatter(df, x="gdpPercap", y="lifeExp", animation_frame="year", size="pop", color="continent", hover_name="country") fig.show()
The output is an interactive scatter plot with a slider representing the years from the dataset.
This code snippet utilizes a built-in dataset from Plotly Express and creates an animated scatter plot. The animation_frame="year"
adds the slider that animates the plot year by year. The resulting graph will update points’ positions, sizes, and colors as the slider moves.
Method 2: Customizing the Slider with Graph Objects
If you require more control over the slider’s appearance and functionality, you can use the lower-level Graph Objects module. You’ll manually create slider objects and define their properties to customize the animation.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(x=[1, 2, 3], y=[4, 5, 6])], layout=go.Layout( sliders=[{ 'steps': [ {'method': 'animate', 'label': 'Frame 1', 'args': [[0]]}, {'method': 'animate', 'label': 'Frame 2', 'args': [[1]]}, {'method': 'animate', 'label': 'Frame 3', 'args': [[2]]} ] }] ), frames=[go.Frame(data=[go.Scatter(x=[1], y=[4])]), go.Frame(data=[go.Scatter(x=[2], y=[5])]), go.Frame(data=[go.Scatter(x=[3], y=[6])])] ) fig.show()
The output is a simple animated line chart with a custom slider.
This code snippet manually constructs a line chart with frames corresponding to different data points. Sliders are added through the layout object, specifying the method and label for each step of the animation.
Method 3: Adding Play and Pause Buttons
To enhance interactivity, you can include play and pause buttons along with the slider. This provides users with better control over the animation, making it more user-friendly.
Here’s an example:
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.express as px df = px.data.gapminder() fig = px.scatter(df, x="gdpPercap", y="lifeExp", animation_frame="year", size="pop", color="continent", hover_name="country") fig.show()
The output is an interactive scatter plot with a slider representing the years from the dataset.
This code snippet utilizes a built-in dataset from Plotly Express and creates an animated scatter plot. The animation_frame="year"
adds the slider that animates the plot year by year. The resulting graph will update points’ positions, sizes, and colors as the slider moves.
Method 2: Customizing the Slider with Graph Objects
If you require more control over the slider’s appearance and functionality, you can use the lower-level Graph Objects module. You’ll manually create slider objects and define their properties to customize the animation.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(x=[1, 2, 3], y=[4, 5, 6])], layout=go.Layout( sliders=[{ 'steps': [ {'method': 'animate', 'label': 'Frame 1', 'args': [[0]]}, {'method': 'animate', 'label': 'Frame 2', 'args': [[1]]}, {'method': 'animate', 'label': 'Frame 3', 'args': [[2]]} ] }] ), frames=[go.Frame(data=[go.Scatter(x=[1], y=[4])]), go.Frame(data=[go.Scatter(x=[2], y=[5])]), go.Frame(data=[go.Scatter(x=[3], y=[6])])] ) fig.show()
The output is a simple animated line chart with a custom slider.
This code snippet manually constructs a line chart with frames corresponding to different data points. Sliders are added through the layout object, specifying the method and label for each step of the animation.
Method 3: Adding Play and Pause Buttons
To enhance interactivity, you can include play and pause buttons along with the slider. This provides users with better control over the animation, making it more user-friendly.
Here’s an example:
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(x=[1, 2, 3], y=[4, 5, 6])], layout=go.Layout( sliders=[{ 'steps': [ {'method': 'animate', 'label': 'Frame 1', 'args': [[0]]}, {'method': 'animate', 'label': 'Frame 2', 'args': [[1]]}, {'method': 'animate', 'label': 'Frame 3', 'args': [[2]]} ] }] ), frames=[go.Frame(data=[go.Scatter(x=[1], y=[4])]), go.Frame(data=[go.Scatter(x=[2], y=[5])]), go.Frame(data=[go.Scatter(x=[3], y=[6])])] ) fig.show()
The output is a simple animated line chart with a custom slider.
This code snippet manually constructs a line chart with frames corresponding to different data points. Sliders are added through the layout object, specifying the method and label for each step of the animation.
Method 3: Adding Play and Pause Buttons
To enhance interactivity, you can include play and pause buttons along with the slider. This provides users with better control over the animation, making it more user-friendly.
Here’s an example:
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.express as px df = px.data.gapminder() fig = px.scatter(df, x="gdpPercap", y="lifeExp", animation_frame="year", size="pop", color="continent", hover_name="country") fig.show()
The output is an interactive scatter plot with a slider representing the years from the dataset.
This code snippet utilizes a built-in dataset from Plotly Express and creates an animated scatter plot. The animation_frame="year"
adds the slider that animates the plot year by year. The resulting graph will update points’ positions, sizes, and colors as the slider moves.
Method 2: Customizing the Slider with Graph Objects
If you require more control over the slider’s appearance and functionality, you can use the lower-level Graph Objects module. You’ll manually create slider objects and define their properties to customize the animation.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(x=[1, 2, 3], y=[4, 5, 6])], layout=go.Layout( sliders=[{ 'steps': [ {'method': 'animate', 'label': 'Frame 1', 'args': [[0]]}, {'method': 'animate', 'label': 'Frame 2', 'args': [[1]]}, {'method': 'animate', 'label': 'Frame 3', 'args': [[2]]} ] }] ), frames=[go.Frame(data=[go.Scatter(x=[1], y=[4])]), go.Frame(data=[go.Scatter(x=[2], y=[5])]), go.Frame(data=[go.Scatter(x=[3], y=[6])])] ) fig.show()
The output is a simple animated line chart with a custom slider.
This code snippet manually constructs a line chart with frames corresponding to different data points. Sliders are added through the layout object, specifying the method and label for each step of the animation.
Method 3: Adding Play and Pause Buttons
To enhance interactivity, you can include play and pause buttons along with the slider. This provides users with better control over the animation, making it more user-friendly.
Here’s an example:
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(x=[1, 2, 3], y=[4, 5, 6])], layout=go.Layout( sliders=[{ 'steps': [ {'method': 'animate', 'label': 'Frame 1', 'args': [[0]]}, {'method': 'animate', 'label': 'Frame 2', 'args': [[1]]}, {'method': 'animate', 'label': 'Frame 3', 'args': [[2]]} ] }] ), frames=[go.Frame(data=[go.Scatter(x=[1], y=[4])]), go.Frame(data=[go.Scatter(x=[2], y=[5])]), go.Frame(data=[go.Scatter(x=[3], y=[6])])] ) fig.show()
The output is a simple animated line chart with a custom slider.
This code snippet manually constructs a line chart with frames corresponding to different data points. Sliders are added through the layout object, specifying the method and label for each step of the animation.
Method 3: Adding Play and Pause Buttons
To enhance interactivity, you can include play and pause buttons along with the slider. This provides users with better control over the animation, making it more user-friendly.
Here’s an example:
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.express as px df = px.data.gapminder() fig = px.scatter(df, x="gdpPercap", y="lifeExp", animation_frame="year", size="pop", color="continent", hover_name="country") fig.show()
The output is an interactive scatter plot with a slider representing the years from the dataset.
This code snippet utilizes a built-in dataset from Plotly Express and creates an animated scatter plot. The animation_frame="year"
adds the slider that animates the plot year by year. The resulting graph will update points’ positions, sizes, and colors as the slider moves.
Method 2: Customizing the Slider with Graph Objects
If you require more control over the slider’s appearance and functionality, you can use the lower-level Graph Objects module. You’ll manually create slider objects and define their properties to customize the animation.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(x=[1, 2, 3], y=[4, 5, 6])], layout=go.Layout( sliders=[{ 'steps': [ {'method': 'animate', 'label': 'Frame 1', 'args': [[0]]}, {'method': 'animate', 'label': 'Frame 2', 'args': [[1]]}, {'method': 'animate', 'label': 'Frame 3', 'args': [[2]]} ] }] ), frames=[go.Frame(data=[go.Scatter(x=[1], y=[4])]), go.Frame(data=[go.Scatter(x=[2], y=[5])]), go.Frame(data=[go.Scatter(x=[3], y=[6])])] ) fig.show()
The output is a simple animated line chart with a custom slider.
This code snippet manually constructs a line chart with frames corresponding to different data points. Sliders are added through the layout object, specifying the method and label for each step of the animation.
Method 3: Adding Play and Pause Buttons
To enhance interactivity, you can include play and pause buttons along with the slider. This provides users with better control over the animation, making it more user-friendly.
Here’s an example:
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(x=[1, 2, 3], y=[4, 5, 6])], layout=go.Layout( sliders=[{ 'steps': [ {'method': 'animate', 'label': 'Frame 1', 'args': [[0]]}, {'method': 'animate', 'label': 'Frame 2', 'args': [[1]]}, {'method': 'animate', 'label': 'Frame 3', 'args': [[2]]} ] }] ), frames=[go.Frame(data=[go.Scatter(x=[1], y=[4])]), go.Frame(data=[go.Scatter(x=[2], y=[5])]), go.Frame(data=[go.Scatter(x=[3], y=[6])])] ) fig.show()
The output is a simple animated line chart with a custom slider.
This code snippet manually constructs a line chart with frames corresponding to different data points. Sliders are added through the layout object, specifying the method and label for each step of the animation.
Method 3: Adding Play and Pause Buttons
To enhance interactivity, you can include play and pause buttons along with the slider. This provides users with better control over the animation, making it more user-friendly.
Here’s an example:
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.express as px df = px.data.gapminder() fig = px.scatter(df, x="gdpPercap", y="lifeExp", animation_frame="year", size="pop", color="continent", hover_name="country") fig.show()
The output is an interactive scatter plot with a slider representing the years from the dataset.
This code snippet utilizes a built-in dataset from Plotly Express and creates an animated scatter plot. The animation_frame="year"
adds the slider that animates the plot year by year. The resulting graph will update points’ positions, sizes, and colors as the slider moves.
Method 2: Customizing the Slider with Graph Objects
If you require more control over the slider’s appearance and functionality, you can use the lower-level Graph Objects module. You’ll manually create slider objects and define their properties to customize the animation.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(x=[1, 2, 3], y=[4, 5, 6])], layout=go.Layout( sliders=[{ 'steps': [ {'method': 'animate', 'label': 'Frame 1', 'args': [[0]]}, {'method': 'animate', 'label': 'Frame 2', 'args': [[1]]}, {'method': 'animate', 'label': 'Frame 3', 'args': [[2]]} ] }] ), frames=[go.Frame(data=[go.Scatter(x=[1], y=[4])]), go.Frame(data=[go.Scatter(x=[2], y=[5])]), go.Frame(data=[go.Scatter(x=[3], y=[6])])] ) fig.show()
The output is a simple animated line chart with a custom slider.
This code snippet manually constructs a line chart with frames corresponding to different data points. Sliders are added through the layout object, specifying the method and label for each step of the animation.
Method 3: Adding Play and Pause Buttons
To enhance interactivity, you can include play and pause buttons along with the slider. This provides users with better control over the animation, making it more user-friendly.
Here’s an example:
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(x=[1, 2, 3], y=[4, 5, 6])], layout=go.Layout( sliders=[{ 'steps': [ {'method': 'animate', 'label': 'Frame 1', 'args': [[0]]}, {'method': 'animate', 'label': 'Frame 2', 'args': [[1]]}, {'method': 'animate', 'label': 'Frame 3', 'args': [[2]]} ] }] ), frames=[go.Frame(data=[go.Scatter(x=[1], y=[4])]), go.Frame(data=[go.Scatter(x=[2], y=[5])]), go.Frame(data=[go.Scatter(x=[3], y=[6])])] ) fig.show()
The output is a simple animated line chart with a custom slider.
This code snippet manually constructs a line chart with frames corresponding to different data points. Sliders are added through the layout object, specifying the method and label for each step of the animation.
Method 3: Adding Play and Pause Buttons
To enhance interactivity, you can include play and pause buttons along with the slider. This provides users with better control over the animation, making it more user-friendly.
Here’s an example:
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
import plotly.express as px df = px.data.gapminder() fig = px.scatter(df, x="gdpPercap", y="lifeExp", animation_frame="year", size="pop", color="continent", hover_name="country") fig.show()
The output is an interactive scatter plot with a slider representing the years from the dataset.
This code snippet utilizes a built-in dataset from Plotly Express and creates an animated scatter plot. The animation_frame="year"
adds the slider that animates the plot year by year. The resulting graph will update points’ positions, sizes, and colors as the slider moves.
Method 2: Customizing the Slider with Graph Objects
If you require more control over the slider’s appearance and functionality, you can use the lower-level Graph Objects module. You’ll manually create slider objects and define their properties to customize the animation.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(x=[1, 2, 3], y=[4, 5, 6])], layout=go.Layout( sliders=[{ 'steps': [ {'method': 'animate', 'label': 'Frame 1', 'args': [[0]]}, {'method': 'animate', 'label': 'Frame 2', 'args': [[1]]}, {'method': 'animate', 'label': 'Frame 3', 'args': [[2]]} ] }] ), frames=[go.Frame(data=[go.Scatter(x=[1], y=[4])]), go.Frame(data=[go.Scatter(x=[2], y=[5])]), go.Frame(data=[go.Scatter(x=[3], y=[6])])] ) fig.show()
The output is a simple animated line chart with a custom slider.
This code snippet manually constructs a line chart with frames corresponding to different data points. Sliders are added through the layout object, specifying the method and label for each step of the animation.
Method 3: Adding Play and Pause Buttons
To enhance interactivity, you can include play and pause buttons along with the slider. This provides users with better control over the animation, making it more user-friendly.
Here’s an example:
import plotly.graph_objs as go from plotly.subplots import make_subplots fig = make_subplots(specs=[[{"secondary_y": True}]]) fig.add_trace(go.Scatter(x=[1, 2, 3], y=[4, 5, 6], name="Data Trace"), secondary_y=False) fig.layout.updatemenus = [ { "type": "buttons", "showactive": False, "buttons": [{"label": "Play", "method": "animate", "args": [None, {"frame": {"duration": 500, "redraw": True}, "fromcurrent": True}]}] } ] fig.show()
The output is a scatter plot with play and pause buttons but without a visible slider.
This code creates a simple Plotly graph and configures the layout’s updatemenus property to include buttons that control the animation play state. Note that this example does not have a slider but demonstrates the addition of control buttons for the animation.
Method 4: Integrating Slider Events
For more advanced interactivity, Plotly’s sliders can respond to various events. You can define event handlers that trigger when the user interacts with the slider to perform additional actions, like updating other parts of your visualization or running custom code.
Here’s an example:
import plotly.graph_objs as go from IPython.display import display, clear_output fig = go.Figure() # ... set up the figure ... # Define the slider change event def slider_change(attr, old, new): clear_output(wait=True) print(f"Slider moved from {old} to {new}") # Add slider and configure event slider = go.layout.Slider(..., steps=[...], active=0) slider.on_change(slider_change) fig.layout.sliders = [slider] fig.show()
The output would be an interactive Plotly visualization with a slider that prints the old and new position whenever it changes.
In this fictional code snippet, an event handler function slider_change
is defined, which prints messages to the console when the slider value changes. This example is abstract as real event binding in Plotly is typically done through JavaScript callbacks within a Dash app context.
Bonus One-Liner Method 5: Using Update Menus for Quick Sliders
Create an animated slider with minimal code by leveraging the update menus feature in Plotly, enabling quick prototyping without extensive slider configurations.
Here’s an example:
import plotly.graph_objs as go fig = go.Figure(data=[...], layout=go.Layout(sliders=[default_slider])) fig.layout.updatemenus = [default_play_button] fig.show()
The exact output depends on the data and slider/button configurations but provides a quick way to add a slider with play/pause functionality.
This one-liner utilizes predefined configurations stored in variables default_slider
and default_play_button
to instantly add an animation slider and control buttons to your Plotly figure with minimal setup.
Summary/Discussion
- Method 1: Using Plotly Express. Straightforward implementation. Limited customization options.
- Method 2: Customizing the Slider with Graph Objects. Offers fine-grained control. Requires more setup and understanding of Plotly’s underlying structure.
- Method 3: Adding Play and Pause Buttons. Enhances user interactivity. May not be intuitive for creating a slider but allows easy animation control.
- Method 4: Integrating Slider Events. Highly interactive and responsive. Advanced; requires additional infrastructure or frameworks like Dash for full functionality.
- Bonus Method 5: Using Update Menus for Quick Sliders. Simple and efficient. Less flexibility and granularity compared to other methods.
February 28, 2024 at 06:28PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
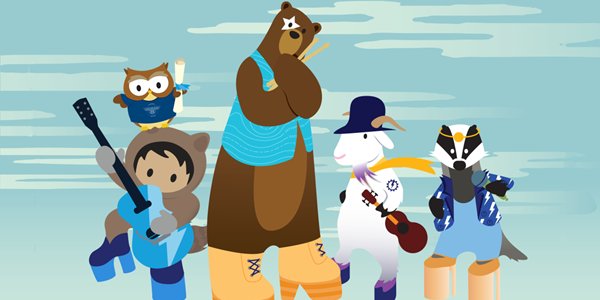
Post a Comment