5 Best Ways to Program to Find Out the Number of Squares in a Grid in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Given a two-dimensional grid, our goal is to compute the total number of squares present. For instance, a 3×3 grid encompasses 14 squares: 9 1×1 squares, 4 2×2 squares, and 1 3×3 square. The desired output would be a numerical result representing this total square count.
Method 1: Iterative Counting
This method uses a simple nested loop structure iterating through possible square sizes. Given a grid of size N x N
, we run through all conceivable top-left corners of a square and all potential square lengths. It provides a clear and robust means of enumerating each square explicitly.
Here’s an example:
def count_squares(n): total_squares = 0 for size in range(1, n+1): for i in range(n-size+1): for j in range(n-size+1): total_squares += 1 return total_squares print(count_squares(3))
Output: 14
This Python function count_squares
takes an integer n
representing the grid size and iterates over all possible squares. It systematically considers each starting position and size, incrementing the count for each square found. This method is very intuitive but may not be the most efficient for large grids due to its cubic time complexity.
Method 2: Mathematical Formula
Understanding the pattern in the square counts leads to a formula: the sum of squares of integers from 1 to N. This method harnesses a more mathematical approach to compute the number of squares, which is efficient and has a time complexity of O(1).
Here’s an example:
def count_squares(n): return (n * (n + 1) * (2 * n + 1)) // 6 print(count_squares(3))
Output: 14
The function count_squares
uses the mathematical formula to directly calculate the total number of squares for a n x n
grid. The result of the formula represents the sum of squares of the integers from 1 to N, capturing all possible square sizes. This method is ideal for performance since it doesn’t involve iteration.
Method 3: Recursive Solution
This method applies a recursive strategy to break down the problem into smaller sub-problems. The total squares in an N x N
grid include all squares from the smaller (N-1) x (N-1)
grid, plus the new squares that have sides of length N.
Here’s an example:
def count_squares(n): if n == 1: return 1 return n * n + count_squares(n - 1) print(count_squares(3))
Output: 14
The recursive count_squares
function calls itself with a decremented size until it reaches the base case of a single square. For each recursive call, it adds the number of squares with the current side length. While elegant, recursion might not be suitable for very large grids due to stack size limitations.
Method 4: Dynamic Programming
Dynamic programming can optimize the recursive approach by storing interim results in a cache to avoid redundant calculations. This method is efficient for computing the count in polynomial time and can handle larger grids effectively.
Here’s an example:
def count_squares(n, memo={}): if n in memo: return memo[n] if n == 1: return 1 memo[n] = n * n + count_squares(n - 1, memo) return memo[n] print(count_squares(3))
Output: 14
The count_squares
function is enhanced by a memoization dictionary memo
that caches previously computed results. The dynamic programming approach improves performance over the simple recursive solution, especially noticeable when dealing with larger values of N.
Bonus One-Liner Method 5: List Comprehension
This bonus method uses Python’s list comprehension to create a compact one-liner that still employs the mathematical sum of squares formula. It achieves the same result in a more Pythonic way.
Here’s an example:
count_squares = lambda n: sum(i*i for i in range(1, n+1)) print(count_squares(3))
Output: 14
The one-liner function count_squares
leverages a generator expression within the built-in sum()
function to accumulate squares of all sizes. This approach offers concise and clear code but is essentially a variation of the mathematical approach and shares its strengths and weaknesses.
Summary/Discussion
- Method 1: Iterative Counting. Offers clarity of the process. Not suitable for large grids due to cubic time complexity.
- Method 2: Mathematical Formula. Highly efficient with constant time complexity. May require additional understanding of mathematical principles.
- Method 3: Recursive Solution. Simple conceptual implementation. Not practical for large grids due to potential stack overflow.
- Method 4: Dynamic Programming. Handles larger grids efficiently. Requires additional space for caching results.
- Method 5: List Comprehension. Pythonic and compact. It’s a simplified expression of the mathematical sum of squares approach, combining readability with efficiency.
March 10, 2024 at 04:36AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
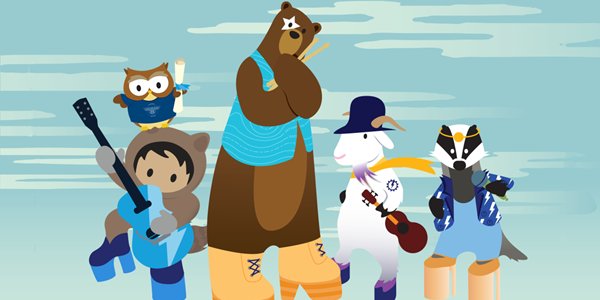
Post a Comment