5 Best Ways to Convert Python HTML String to Image : Emily Rosemary Collins
5 Best Ways to Convert Python HTML String to Image
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Converting HTML content to images programmatically is a common requirement for generating thumbnails, previews, or for graphical representation. You might have a Python string containing HTML code and you need to render it as an image – perhaps a PNG or JPEG file. For instance, you receive “
February 18, 2024 at 07:11PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post

<div>Hello, World!</div>
” and you need to make an image that shows these words styled as they would appear in a web browser.
Method 1: Using Webkit Rendering with QtWebKit
The PyQt5 QtWebKit module provides a way to render HTML content into images using the Webkit engine. This functionality can be used to convert any HTML string into a raster image format such as PNG or JPEG.Here’s an example:
from PyQt5.QtWidgets import QApplication from PyQt5.QtWebEngineWidgets import QWebEngineView from PyQt5.QtCore import QUrl from PyQt5.QtGui import QImage, QPainter import sys, io app = QApplication(sys.argv) html_content = '<html><body><h1>Hello, World!</h1></body></html>' view = QWebEngineView() def capture_func(): size = view.page().contentsSize().toSize() image = QImage(size, QImage.Format_ARGB32) painter = QPainter(image) view.page().view().render(painter) painter.end() image.save('output.png') app.exit() view.setHtml(html_content) view.resize(800, 600) view.show() view.page().loadFinished.connect(capture_func) sys.exit(app.exec_())
This code snippet will generate an image file named “output.png” in the current directory.
The code creates an instance of QWebEngineView, sets the provided HTML content, and, once the page loading is finished, captures the webpage’s render into an image. By using QPainter, the webpage content is painted onto a QImage object which is then saved to a file.Method 2: Using Selenium WebDriver
Selenium WebDriver is a tool for automating web browser interaction, which can also be used to capture screenshots of webpages. By rendering the HTML in a browser context, Selenium can capture a screenshot and save it as an image.Here’s an example:
from selenium import webdriver options = webdriver.ChromeOptions() options.add_argument('headless') driver = webdriver.Chrome(options=options) html_content = "<html><body><p>Python rocks!</p></body></html>" driver.get("data:text/html;charset=utf-8," + html_content) driver.save_screenshot('screenshot.png') driver.quit()
This code snippet will create a file ‘screenshot.png’ with the rendered HTML.
In this snippet, Selenium opens a headless Chrome browser, loads the HTML string directly as the content of a new webpage, and then saves the entire browser window as a screenshot. This method is powerful but can be heavy on resources since it requires a full browser environment.Method 3: Using imgkit
ImgKit is a Python wrapper for the wkhtmltoimage command-line tool, which converts HTML to images using the Webkit rendering engine. It can be used to convert HTML strings or files to various image formats with ease.Here’s an example:
import imgkit html_content = "<h1>Capture this as an image</h1>" imgkit.from_string(html_content, 'output.jpg')
This code creates an image file ‘output.jpg’ that contains the HTML-rendered text.
The example uses imgkit’s `from_string()` function to render the provided HTML string to an image, specifying the output filename. This method is a simple and straightforward way to convert HTML to images, but it relies on the external tool wkhtmltoimage.Method 4: Using HTML/CSS to Image API
For those who prefer a service-based approach, the HTML/CSS to Image API is a web-based solution that can convert HTML and CSS to images. It requires an internet connection and usage might involve fee-based subscription for high volume processing.Here’s an example:
import requests html_content = "<div>Hello from the web service</div>" api_url = 'https://hcti.io/v1/image' api_key = 'your_api_key' api_id = 'your_api_id' data = {'html': html_content} response = requests.post(api_url, data=data, auth=(api_id, api_key)) if response.status_code == 200: with open('service_output.png', 'wb') as f: f.write(response.content)
The output is an image saved as ‘service_output.png’ containing the HTML content.
This snippet sends a POST request to the HTML/CSS to Image API with the HTML content, and then writes the binary response (the image) to a file. It’s a hassle-free method, with the complexity of rendering and image generation handled by the external service.Bonus One-Liner Method 5: Using MSPaint via pyautogui
A creative and somewhat unconventional approach involves using the pyautogui module to script interactions with MSPaint or another graphics editing program to “draw” the HTML content as text on a canvas, then save it as an image.Here’s an example:
import pyautogui # Ensure that MSPaint is open and the screen is configured properly html_content = "Python is fun!" pyautogui.typewrite(html_content) pyautogui.hotkey('ctrl', 's')
The output is the work saved in MSPaint.
In this playful snippet, after manually preparing the MSPaint window, pyautogui scripts the typing of the HTML content as regular text and saves the file using a keyboard shortcut. It’s not a production-reliable method, but it’s a fun demonstration of what’s possible with automation.Summary/Discussion
- Method 1: QtWebKit. High-quality rendering with Webkit. Requires a PyQt5 environment and may be complex to set up.
- Method 2: Selenium WebDriver. Accurate browser-based rendering. Resource-intensive and requires a webdriver setup.
- Method 3: imgkit. Easy to use and decent quality. Dependent on the wkhtmltoimage external tool.
- Method 4: HTML/CSS to Image API. Convenient API service. Requires internet access and potential cost for volume use.
- Bonus Method 5: MSPaint via pyautogui. Creative and fun. Not practical for reliable or scalable use.
February 18, 2024 at 07:11PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
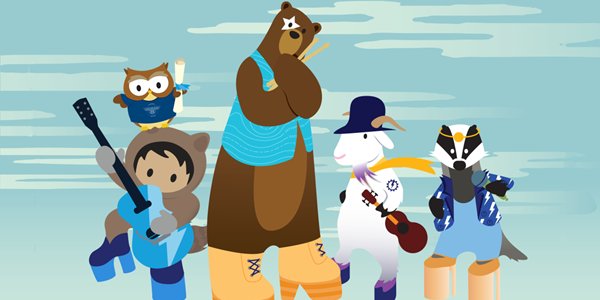
Post a Comment