5 Best Ways to Convert Python Datetime to Integer Seconds : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: In Python, developers often need to convert datetime objects to an integer representing seconds for easier calculations or storage. The input in question is a datetime object, say
datetime.datetime(2021, 1, 1, 0, 0)
, and the desired output is the corresponding number of seconds since a standard epoch time, typically January 1, 1970, 00:00 UTC, which would be 1609459200
.
Method 1: Using datetime.timestamp()
This method utilizes the timestamp()
method from Python’s datetime module, which returns the time expressed as the number of seconds since the epoch. The timestamp()
method is straightforward and does not require any additional libraries.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = int(dt.timestamp()) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The code converts the datetime object into a time tuple and then uses the mktime()
function to get the number of seconds since the epoch, which is also converted to an integer for consistency.
Method 3: Using calendar.timegm()
with datetime.utctimetuple()
An alternative for UTC datetimes is to use the calendar.timegm()
method in combination with datetime.utctimetuple()
, making this method more suitable for UTC conversions and avoiding any local time discrepancies.
Here’s an example:
import datetime import calendar dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = calendar.timegm(dt.utctimetuple()) print(seconds)
Output:
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
This snippet creates a datetime object representing January 1, 2021. The timestamp()
method then converts this datetime to seconds since the Unix epoch, which is converted to an integer.
Method 2: Using time.mktime()
with datetime.timetuple()
Another approach is to combine time.mktime()
with datetime.timetuple()
. The timetuple()
method converts the datetime into a time tuple which is compatible with the mktime()
function from the time module to get the epoch seconds.
Here’s an example:
import datetime import time dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = int(time.mktime(dt.timetuple())) print(seconds)
Output:
1609459200
The code converts the datetime object into a time tuple and then uses the mktime()
function to get the number of seconds since the epoch, which is also converted to an integer for consistency.
Method 3: Using calendar.timegm()
with datetime.utctimetuple()
An alternative for UTC datetimes is to use the calendar.timegm()
method in combination with datetime.utctimetuple()
, making this method more suitable for UTC conversions and avoiding any local time discrepancies.
Here’s an example:
import datetime import calendar dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = calendar.timegm(dt.utctimetuple()) print(seconds)
Output:
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
This snippet creates a datetime object representing January 1, 2021. The timestamp()
method then converts this datetime to seconds since the Unix epoch, which is converted to an integer.
Method 2: Using time.mktime()
with datetime.timetuple()
Another approach is to combine time.mktime()
with datetime.timetuple()
. The timetuple()
method converts the datetime into a time tuple which is compatible with the mktime()
function from the time module to get the epoch seconds.
Here’s an example:
import datetime import time dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = int(time.mktime(dt.timetuple())) print(seconds)
Output:
1609459200
The code converts the datetime object into a time tuple and then uses the mktime()
function to get the number of seconds since the epoch, which is also converted to an integer for consistency.
Method 3: Using calendar.timegm()
with datetime.utctimetuple()
An alternative for UTC datetimes is to use the calendar.timegm()
method in combination with datetime.utctimetuple()
, making this method more suitable for UTC conversions and avoiding any local time discrepancies.
Here’s an example:
import datetime import calendar dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = calendar.timegm(dt.utctimetuple()) print(seconds)
Output:
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
This snippet creates a datetime object representing January 1, 2021. The timestamp()
method then converts this datetime to seconds since the Unix epoch, which is converted to an integer.
Method 2: Using time.mktime()
with datetime.timetuple()
Another approach is to combine time.mktime()
with datetime.timetuple()
. The timetuple()
method converts the datetime into a time tuple which is compatible with the mktime()
function from the time module to get the epoch seconds.
Here’s an example:
import datetime import time dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = int(time.mktime(dt.timetuple())) print(seconds)
Output:
1609459200
The code converts the datetime object into a time tuple and then uses the mktime()
function to get the number of seconds since the epoch, which is also converted to an integer for consistency.
Method 3: Using calendar.timegm()
with datetime.utctimetuple()
An alternative for UTC datetimes is to use the calendar.timegm()
method in combination with datetime.utctimetuple()
, making this method more suitable for UTC conversions and avoiding any local time discrepancies.
Here’s an example:
import datetime import calendar dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = calendar.timegm(dt.utctimetuple()) print(seconds)
Output:
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The code converts the datetime object into a time tuple and then uses the mktime()
function to get the number of seconds since the epoch, which is also converted to an integer for consistency.
Method 3: Using calendar.timegm()
with datetime.utctimetuple()
An alternative for UTC datetimes is to use the calendar.timegm()
method in combination with datetime.utctimetuple()
, making this method more suitable for UTC conversions and avoiding any local time discrepancies.
Here’s an example:
import datetime import calendar dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = calendar.timegm(dt.utctimetuple()) print(seconds)
Output:
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
This snippet creates a datetime object representing January 1, 2021. The timestamp()
method then converts this datetime to seconds since the Unix epoch, which is converted to an integer.
Method 2: Using time.mktime()
with datetime.timetuple()
Another approach is to combine time.mktime()
with datetime.timetuple()
. The timetuple()
method converts the datetime into a time tuple which is compatible with the mktime()
function from the time module to get the epoch seconds.
Here’s an example:
import datetime import time dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = int(time.mktime(dt.timetuple())) print(seconds)
Output:
1609459200
The code converts the datetime object into a time tuple and then uses the mktime()
function to get the number of seconds since the epoch, which is also converted to an integer for consistency.
Method 3: Using calendar.timegm()
with datetime.utctimetuple()
An alternative for UTC datetimes is to use the calendar.timegm()
method in combination with datetime.utctimetuple()
, making this method more suitable for UTC conversions and avoiding any local time discrepancies.
Here’s an example:
import datetime import calendar dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = calendar.timegm(dt.utctimetuple()) print(seconds)
Output:
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The code converts the datetime object into a time tuple and then uses the mktime()
function to get the number of seconds since the epoch, which is also converted to an integer for consistency.
Method 3: Using calendar.timegm()
with datetime.utctimetuple()
An alternative for UTC datetimes is to use the calendar.timegm()
method in combination with datetime.utctimetuple()
, making this method more suitable for UTC conversions and avoiding any local time discrepancies.
Here’s an example:
import datetime import calendar dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = calendar.timegm(dt.utctimetuple()) print(seconds)
Output:
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
This snippet creates a datetime object representing January 1, 2021. The timestamp()
method then converts this datetime to seconds since the Unix epoch, which is converted to an integer.
Method 2: Using time.mktime()
with datetime.timetuple()
Another approach is to combine time.mktime()
with datetime.timetuple()
. The timetuple()
method converts the datetime into a time tuple which is compatible with the mktime()
function from the time module to get the epoch seconds.
Here’s an example:
import datetime import time dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = int(time.mktime(dt.timetuple())) print(seconds)
Output:
1609459200
The code converts the datetime object into a time tuple and then uses the mktime()
function to get the number of seconds since the epoch, which is also converted to an integer for consistency.
Method 3: Using calendar.timegm()
with datetime.utctimetuple()
An alternative for UTC datetimes is to use the calendar.timegm()
method in combination with datetime.utctimetuple()
, making this method more suitable for UTC conversions and avoiding any local time discrepancies.
Here’s an example:
import datetime import calendar dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = calendar.timegm(dt.utctimetuple()) print(seconds)
Output:
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The code converts the datetime object into a time tuple and then uses the mktime()
function to get the number of seconds since the epoch, which is also converted to an integer for consistency.
Method 3: Using calendar.timegm()
with datetime.utctimetuple()
An alternative for UTC datetimes is to use the calendar.timegm()
method in combination with datetime.utctimetuple()
, making this method more suitable for UTC conversions and avoiding any local time discrepancies.
Here’s an example:
import datetime import calendar dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = calendar.timegm(dt.utctimetuple()) print(seconds)
Output:
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
This snippet creates a datetime object representing January 1, 2021. The timestamp()
method then converts this datetime to seconds since the Unix epoch, which is converted to an integer.
Method 2: Using time.mktime()
with datetime.timetuple()
Another approach is to combine time.mktime()
with datetime.timetuple()
. The timetuple()
method converts the datetime into a time tuple which is compatible with the mktime()
function from the time module to get the epoch seconds.
Here’s an example:
import datetime import time dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = int(time.mktime(dt.timetuple())) print(seconds)
Output:
1609459200
The code converts the datetime object into a time tuple and then uses the mktime()
function to get the number of seconds since the epoch, which is also converted to an integer for consistency.
Method 3: Using calendar.timegm()
with datetime.utctimetuple()
An alternative for UTC datetimes is to use the calendar.timegm()
method in combination with datetime.utctimetuple()
, making this method more suitable for UTC conversions and avoiding any local time discrepancies.
Here’s an example:
import datetime import calendar dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = calendar.timegm(dt.utctimetuple()) print(seconds)
Output:
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
The code converts the datetime object into a time tuple and then uses the mktime()
function to get the number of seconds since the epoch, which is also converted to an integer for consistency.
Method 3: Using calendar.timegm()
with datetime.utctimetuple()
An alternative for UTC datetimes is to use the calendar.timegm()
method in combination with datetime.utctimetuple()
, making this method more suitable for UTC conversions and avoiding any local time discrepancies.
Here’s an example:
import datetime import calendar dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = calendar.timegm(dt.utctimetuple()) print(seconds)
Output:
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
1609459200
This snippet creates a datetime object representing January 1, 2021. The timestamp()
method then converts this datetime to seconds since the Unix epoch, which is converted to an integer.
Method 2: Using time.mktime()
with datetime.timetuple()
Another approach is to combine time.mktime()
with datetime.timetuple()
. The timetuple()
method converts the datetime into a time tuple which is compatible with the mktime()
function from the time module to get the epoch seconds.
Here’s an example:
import datetime import time dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = int(time.mktime(dt.timetuple())) print(seconds)
Output:
1609459200
The code converts the datetime object into a time tuple and then uses the mktime()
function to get the number of seconds since the epoch, which is also converted to an integer for consistency.
Method 3: Using calendar.timegm()
with datetime.utctimetuple()
An alternative for UTC datetimes is to use the calendar.timegm()
method in combination with datetime.utctimetuple()
, making this method more suitable for UTC conversions and avoiding any local time discrepancies.
Here’s an example:
import datetime import calendar dt = datetime.datetime(2021, 1, 1, 0, 0) seconds = calendar.timegm(dt.utctimetuple()) print(seconds)
Output:
1609459200
The example illustrates converting a UTC datetime into a time tuple and then getting the epoch seconds with timegm()
, which is automatically an integer.
Method 4: Manual Calculation Using datetime
Attributes
For a more manual approach, one can calculate the seconds by breaking down the datetime object into individual components and calculating the total seconds accordingly. This method is useful if you need a calculation without relying on specific conversion functions.
Here’s an example:
import datetime dt = datetime.datetime(2021, 1, 1, 0, 0) dt_epoch = datetime.datetime(1970, 1, 1, 0, 0) delta = dt - dt_epoch seconds = delta.days * 24 * 3600 + delta.seconds print(seconds)
Output:
1609459200
The code snippet manually calculates the seconds by finding the time delta between the datetime object and the epoch, then multiplying the days by the number of seconds in a day and adding the leftover seconds.
Bonus One-Liner Method 5: Using a Lambda Function
A concise one-liner solution for quick conversions could be a lambda function that takes a datetime object and returns the equivalent integer seconds.
Here’s an example:
import datetime dt_to_seconds = lambda dt: int(dt.timestamp()) seconds = dt_to_seconds(datetime.datetime(2021, 1, 1, 0, 0)) print(seconds)
Output:
1609459200
The lambda function dt_to_seconds
uses the timestamp()
method for conversion, making it a compact and reusable piece of code for converting multiple datetime objects.
Summary/Discussion
- Method 1:
datetime.timestamp()
. Strengths: Simple and concise. Weaknesses: Not timezone-aware. - Method 2:
time.mktime()
withdatetime.timetuple()
. Strengths: Useful for local time conversions. Weaknesses: Timezone-dependent, can cause confusion with daylight savings. - Method 3:
calendar.timegm()
withdatetime.utctimetuple()
. Strengths: UTC conversion, good for global time standards. Weaknesses: Not suitable for local timezones. - Method 4: Manual calculation. Strengths: Full control over the calculation process. Weaknesses: More verbose and error-prone.
- Method 5: Lambda function. Strengths: Compact, easy to reuse. Weaknesses: Requires understanding of lambda and
timestamp()
.
February 18, 2024 at 09:50PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
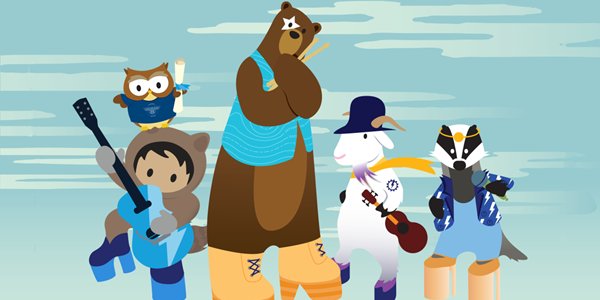
Post a Comment