Python Ternary Multiple Times : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
The Python ternary operator, also known as the conditional operator, is a concise way to write simple if-else statements. Introduced in Python 2.5, this operator allows you to return one of two values depending on the evaluation of a given condition.
You might find the ternary operator particularly useful when you want to write clean and readable code without compromising on functionality. The syntax for the ternary operator in Python is
value_if_true if condition else value_if_false
For example, you can use it to assign a value to a variable depending on whether a certain condition is met.
Nested Ternary Example
When working with multiple conditions, it’s possible to use the Python ternary operator multiple times by nesting them. This can help you handle more complex scenarios while still maintaining the concise and readable format provided by the ternary operator.
Nested ternary expressions in Python are a compact way to combine multiple conditional operations within a single line of code. They follow the format of [on_true] if [condition] else [on_false]
and can be nested to handle more complex conditions. However, while they can make the code more concise, they can also reduce readability, especially when overused or used in complex scenarios.
Here’s a minimal example without nested ternary:
x = 5 result = "Positive" if x > 0 else "Non-positive"
In this example, result
will be "Positive"
if x
is greater than 0, otherwise "Non-positive"
.
Now, with nested ternary:
x = 5 result = "Positive" if x > 0 else "Zero" if x == 0 else "Negative"
Here, result
will be "Positive"
if x
is greater than 0, "Zero"
if x
is 0, and "Negative"
if x
is less than 0. The nested ternary allows us to add an additional condition, but as you can see, it becomes more complex to read.
Here’s the example without nested ternary, using simple if/else:
x = 5 if x > 0: result = "Positive" else: result = "Non-positive"
In this example, result
is set to "Positive"
if x
is greater than 0, and "Non-positive"
otherwise.
And now, with a more complex condition, similar to the nested ternary example, using if/elif/else:
x = 5 if x > 0: result = "Positive" elif x == 0: result = "Zero" else: result = "Negative"
Here, result
will be "Positive"
if x
is greater than 0, "Zero"
if x
is 0, and "Negative"
if x
is less than 0.
Working with Python Ternary Operators

Basics of Ternary Operators
The syntax for a ternary operator is
<variable> = <true_value> if <condition> else <false_value>
For example, consider a simple grading system that assigns values to the variable score
and determines whether a student passes or fails. Using a traditional if-else statement, it would look like this:
if score >= 60: result = "Pass" else: result = "Fail"
With a ternary operator, the same logic can be implemented more concisely:
result = "Pass" if score >= 60 else "Fail"
Here, the condition is score >= 60
, and the variables result
will be assigned the value "Pass"
if the condition is true, and "Fail"
otherwise.
Using Python Ternary with Multiple Conditions
Python ternary operators can also handle multiple conditions in one line of code by nesting them. Instead of using an elif
statement or creating multiple lines, you can combine conditions by nesting ternary operators.
For instance, let’s say you want to assign a letter grade (A, B, C, D, or F) based on a student’s score. Using nested ternary operators, you can achieve this in just one line:
grade = "A" if score >= 90 else "B" if score >= 80 else "C" if score >= 70 else "D" if score >= 60 else "F"
In this example, the code will evaluate each condition in order, starting with score >= 90
and assign the corresponding grade value to the variable grade
based on the given condition.
Advanced Python Ternary Operators Usage
In this section, we will explore some advanced usage of Python ternary operators and their various applications.
Python Ternary Operators with Tuples
Using tuples in a ternary expression can provide a simpler and more efficient way to write a conditional expression. The syntax for using tuples in a ternary operator is as follows:
(result_if_false, result_if_true)[condition]
For example, if you want to determine whether a number is even or odd, you can use tuples in the following way:
number = 4 result = ("odd", "even")[number % 2 == 0] print(result) # Output: "even"
This code snippet checks if the remainder when dividing the number by 2 is 0 (i.e., if the number is even) and returns the corresponding string "even"
or "odd"
based on the condition.
Python Ternary Operators with Comprehensions and Lambdas
List comprehensions and lambda functions are other ways to use ternary operators in Python. They can be useful when you need to create a list or dictionary with conditional expressions.
For example, let’s create a list with the square of even numbers and the cube of odd numbers from 1 to 10 using list comprehension combined with a ternary operator:
result = [x**3 if x % 2 else x**2 for x in range(1, 11)] print(result) # Output: [1, 4, 27, 16, 125, 36, 343, 64, 729, 100]
This example iterates over numbers from 1 to 10 and applies the relevant conditional expressions for even and odd numbers.
Similarly, you can use a lambda function to create more concise and efficient expressions that utilize ternary operators. Below is an example of using lambda to determine if a given number is prime:
is_prime = lambda num: all(num % i != 0 for i in range(2, int(num**0.5) + 1)) if num > 1 else False result = is_prime(17) print(result) # Output: True
Frequently Asked Questions
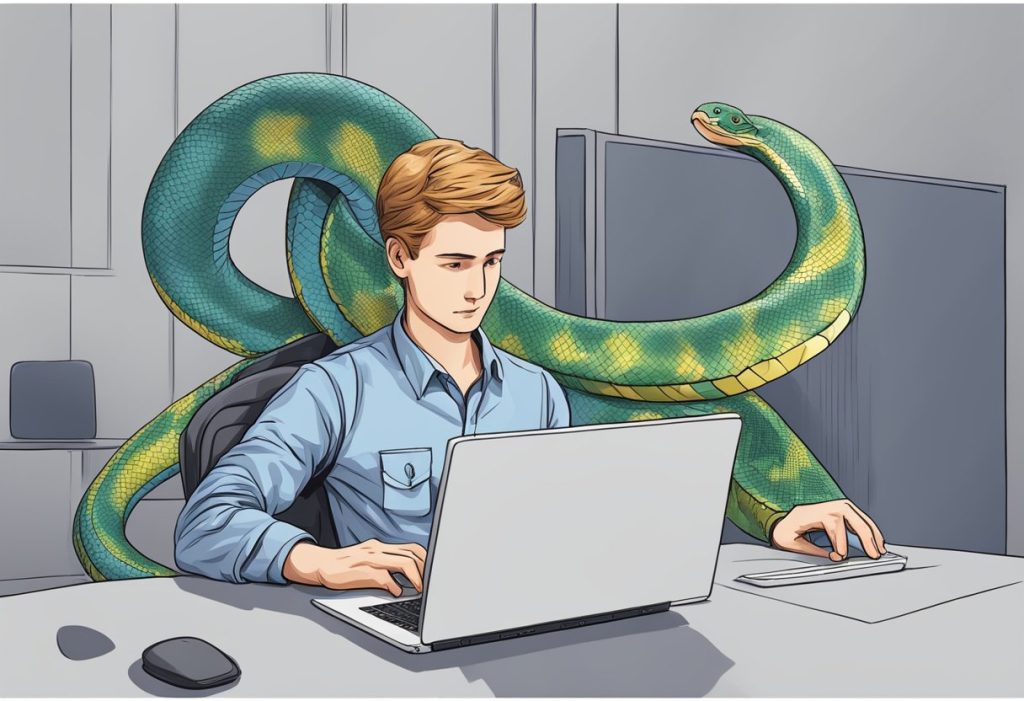
How do you use nested ternary operators in Python?
To use nested ternary operators in Python, you can place another ternary operator inside the else clause of the first one. For example:
result = x if condition1 else (y if condition2 else z)
This evaluates condition1
, and if true, assigns x
to result
. If condition1
is false, it then evaluates condition2
, and if true, assigns y
to result
. If both conditions are false, z
is assigned to result
.
Can you perform multiple actions with a ternary operator in Python?
Ternary operators in Python are designed for simplifying single-action, if-else statements. However, you can use tuples to perform multiple actions, but keep in mind that this may reduce the readability of your code:
result = (action1, action2)[True if condition else False]
What is the syntax for a ternary operator with three conditions in Python?
The syntax for a ternary operator with three conditions in Python requires nested ternary operators. Here’s an example:
result = x if condition1 else (y if condition2 else z)
This code first evaluates condition1
, then condition2
if necessary, and assigns the corresponding value to result
.
Is it possible to nest ternary operators in Python?
Yes, it is possible to nest ternary operators in Python. Just like in the first example, you can place another ternary operator inside the else clause to create nested operators:
result = x if condition1 else (y if condition2 else z)
How readable are Python ternary operators?
Python ternary operators are generally more readable than traditional if-else statements, especially when used properly in simple and short statements. However, excessive nesting and complex conditions can reduce readability. It’s important to strike a balance between readability and brevity when using ternary operators.
What alternatives exist for ternary operators in Python?
If ternary operators become too complex or reduce the readability of your code, you can use traditional if-else statements as an alternative. For example, instead of writing:
x = a if condition else b
You can write:
if condition: x = a else: x = b
This approach provides more flexibility for complex conditions, and can be easier to understand for longer blocks of code.
December 10, 2023 at 07:27PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
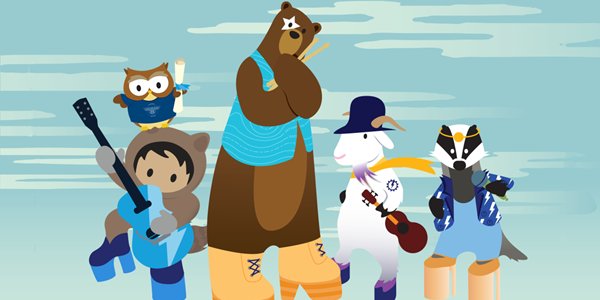
Post a Comment