Python Return Lambda From Function : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
How to Return a Lambda Function From a Function?
In Python, you can return a lambda function from another function by declaring the lambda function inside the return
statement of the outer function. The returned lambda function can then be assigned to a variable and used just like any other function, and it will have access to the arguments and variables of the outer function.
Here’s a simple example:
def make_multiplier(n): return lambda x: x * n # Usage: times_two = make_multiplier(2) print(times_two(4)) # Outputs: 8 times_three = make_multiplier(3) print(times_three(4)) # Outputs: 12
The make_multiplier
function takes an argument n
, and returns a lambda function. This returned function takes an argument x
and multiplies it by n
. When we call make_multiplier(2)
, we get back a function that multiplies its argument by 2. When we call make_multiplier(3)
, we get back a function that multiplies its argument by 3.
Note: While lambdas can be used to create small, anonymous functions, they have some limitations compared to full
def
defined functions. For example, they can only contain expressions and can’t include statements, they don’t have a name and don’t have their own local scope for variable assignments.
Understanding Lambda Functions

Lambda functions in Python are anonymous functions, meaning they don’t have a name. They are used for simple, short operations that can be defined in one line of code. Lambda functions are versatile and frequently used in functional programming as they can help reduce the number of lines of code, making your code more efficient and easier to read.
Python Syntax and Usage
The syntax for a lambda function is quite simple and elegant:
lambda arguments: expression
The lambda
keyword is used to define an anonymous function. The arguments are optional and can be one or more variables separated by commas. The expression is a single line of code that uses the input arguments and returns a value.
But before we move on, I’m excited to present you my new Python book Python One-Liners (Amazon Link).
If you like one-liners, you’ll LOVE the book. It’ll teach you everything there is to know about a single line of Python code. But it’s also an introduction to computer science, data science, machine learning, and algorithms. The universe in a single line of Python!
The book was released in 2020 with the world-class programming book publisher NoStarch Press (San Francisco).
Link: https://nostarch.com/pythononeliners
Here’s an example of a simple lambda function that takes two arguments x
and y
, and returns their sum:
add = lambda x, y: x + y print(add(3, 4)) # Output: 7
In this case, the lambda function is assigned to a variable called add
, which can then be used like any other function.
Lambda functions are frequently used with higher-order functions like map
, filter
, and reduce
. Here’s an example using the map
function to square a list of numbers:
numbers = [1, 2, 3, 4, 5] squared = list(map(lambda x: x ** 2, numbers)) print(squared) # Output: [1, 4, 9, 16, 25]
In this example, the map
function takes two arguments: a lambda function that defines the operation to perform on each element, and the input list of numbers
. The lambda function squares each input element, and the result is stored in a new list called squared
.
Lambda functions can be versatile and provide a clean, concise way to write simple functions when used properly. Keep their usage limited to short and simple expressions, but feel confident in their effectiveness when they are necessary for your Python code.
Writing Efficient Lambda Functions
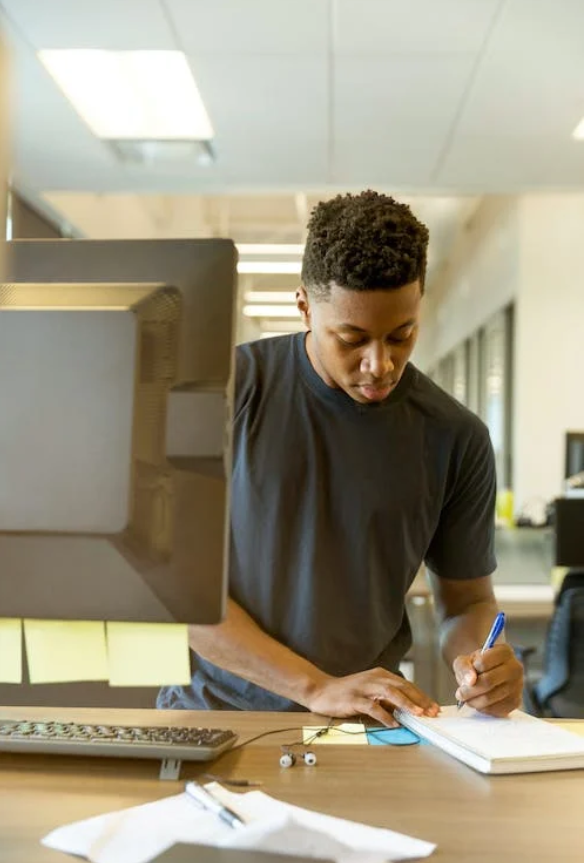
Lambda functions, or anonymous functions, are a concise way to create small functions in Python. They can be quite useful for simple operations, but understanding how to write efficient lambda functions is important for minimizing runtime and improving the overall performance of your code.
One key aspect of writing efficient lambda functions is to avoid complex expressions. Keep your lambda functions concise by focusing on simple operations that can be easily understood.
For example:
# Good lambda function example square = lambda x: x * x # Bad lambda function example complicated_lambda = lambda x, y, z: (x + y) / z if z != 0 else None
Lambda functions are designed to be simple, so make use of higher-order functions like map
, filter
, and reduce
.
These functions allow you to pass a lambda function along with a sequence to perform an operation on each element:
# Using lambda with map squared_numbers = map(lambda x: x * x, [1, 2, 3, 4, 5]) # Using lambda with filter even_numbers = filter(lambda x: x % 2 == 0, [1, 2, 3, 4, 5]) # Using lambda with reduce from functools import reduce sum_of_numbers = reduce(lambda x, y: x + y, [1, 2, 3, 4, 5])
Unlike normal Python functions, lambda functions only allow for a single expression, and they cannot contain any statements. This can make some lambda functions harder to understand and less efficient. If you find that a lambda function is becoming complex or difficult to read, it’s better to switch to a regular Python function.
Lambda Functions vs Regular Functions
Lambda functions and regular functions in Python serve similar purposes, but they differ in various aspects. Before diving into the differences, let’s get a brief overview of each.
Lambda functions are anonymous, one-liner functions defined using the lambda
keyword. They’re utilized mostly for simple operations. Here’s an example:
multiply = lambda x, y: x * y
Regular functions, on the other hand, are defined using the def
keyword and are useful for more complex operations. An example of a regular function:
def multiply(x, y): return x * y
When to Use Each
Lambda Functions
- Short, simple operations: Lambda functions are best suited for single-line operations that can be expressed concisely and don’t demand high readability.
- Higher-order functions: They’re often used as arguments for higher-order functions like
map()
andfilter()
, where the function can be easily defined inline. For example:
squares = map(lambda x: x**2, [1, 2, 3, 4, 5])
- Temporary use: When you require a function for a short period and don’t need to reuse it multiple times, lambda functions can be the ideal choice.
Regular Functions
- Readability: Regular functions using the
def
keyword offer improved code readability, especially if the logic is complex and involves multiple lines. - Function definition: Regular functions have a clear structure, making them suitable for larger codebases where clarity and maintainability are essential.
- Error handling: Regular functions support error handling with
try
–except
blocks, while lambda functions don’t. This is important for handling and preventing errors in more complex operations. - Advanced features: Regular functions are better suited for implementing advanced features like decorators, generators and more, providing greater flexibility than lambda functions.
In summary, use lambda functions for concise, simple operations and temporary use cases. For anything requiring better readability, error handling, or advanced features, opt for regular Python functions defined with the def
keyword.
Frequently Asked Questions
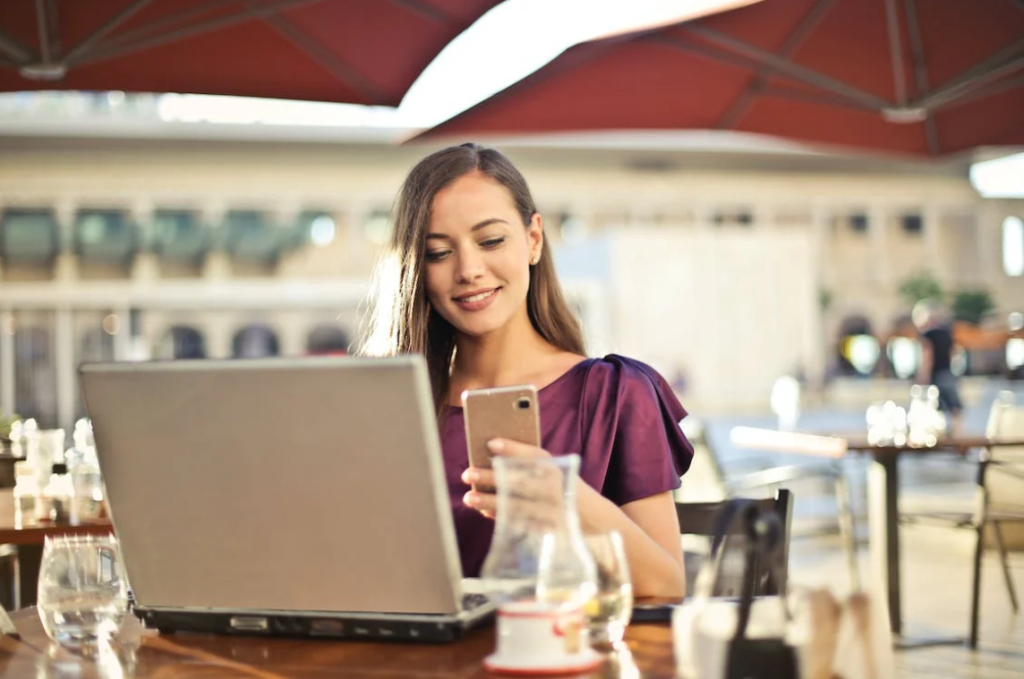
How do you create a lambda function in Python?
Creating a lambda function in Python is quite simple. You can use the lambda
keyword, followed by a set of parameters, a colon, and an expression. For example:
add = lambda x, y: x + y result = add(1, 2) print(result) # Output: 3
What are some use cases for lambda functions in Python?
Lambda functions are often used when you need a small function for a short period of time, and defining a whole function using def
is unnecessary. They are commonly used with functions like map()
, filter()
, and sorted()
where you want to pass a simple transformation or comparison function.
How do you use lambda functions with map and filter in Python?
You can use lambda functions with map()
and filter()
by passing the lambda function as an argument. For example, using map()
:
numbers = [1, 2, 3, 4] squared = list(map(lambda x: x ** 2, numbers)) print(squared) # Output: [1, 4, 9, 16]
And using filter()
:
even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) print(even_numbers) # Output: [2, 4]
What are the differences between regular functions and lambda functions in Python?
Regular functions are defined using the def
keyword, while lambda functions use the lambda
keyword. Regular functions can have multiple lines of code and multiple expressions, while lambda functions are limited to a single expression. Regular functions can have a name, making them reusable, while lambda functions are anonymous and often used for one-time computations.
How do you use if-else statements in lambda functions in Python?
Lambda functions support conditional expressions using an if-else statement. Here’s an example:
check_even = lambda x: "Even" if x % 2 == 0 else "Odd" result = check_even(4) print(result) # Output: "Even"
Can you use a lambda function without any arguments in Python?
Yes, it is possible to define a lambda function without any arguments. Such a lambda function looks like this:
get_hello = lambda: "Hello, World!" message = get_hello() print(message) # Output: "Hello, World!"
In this case, the lambda function returns the string “Hello, World!” when it is called.
Python One-Liners Book: Master the Single Line First!
Python programmers will improve their computer science skills with these useful one-liners.
Python One-Liners will teach you how to read and write “one-liners”: concise statements of useful functionality packed into a single line of code. You’ll learn how to systematically unpack and understand any line of Python code, and write eloquent, powerfully compressed Python like an expert.
The book’s five chapters cover (1) tips and tricks, (2) regular expressions, (3) machine learning, (4) core data science topics, and (5) useful algorithms.
Detailed explanations of one-liners introduce key computer science concepts and boost your coding and analytical skills. You’ll learn about advanced Python features such as list comprehension, slicing, lambda functions, regular expressions, map and reduce functions, and slice assignments.
You’ll also learn how to:
- Leverage data structures to solve real-world problems, like using Boolean indexing to find cities with above-average pollution
- Use NumPy basics such as array, shape, axis, type, broadcasting, advanced indexing, slicing, sorting, searching, aggregating, and statistics
- Calculate basic statistics of multidimensional data arrays and the K-Means algorithms for unsupervised learning
- Create more advanced regular expressions using grouping and named groups, negative lookaheads, escaped characters, whitespaces, character sets (and negative characters sets), and greedy/nongreedy operators
- Understand a wide range of computer science topics, including anagrams, palindromes, supersets, permutations, factorials, prime numbers, Fibonacci numbers, obfuscation, searching, and algorithmic sorting
By the end of the book, you’ll know how to write Python at its most refined, and create concise, beautiful pieces of “Python art” in merely a single line.
Get your Python One-Liners on Amazon!!
July 31, 2023 at 02:30AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
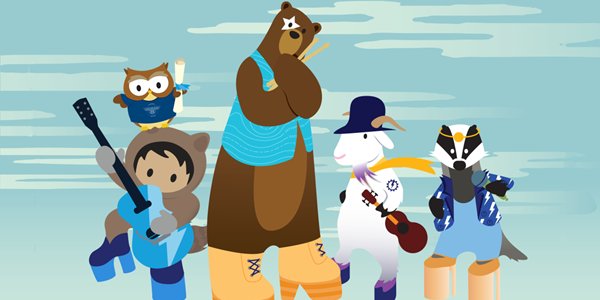
Post a Comment