(Fix) ValueError: Argument Z must be 2-dimensional : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation
import matplotlib.pyplot as plt import numpy as np X = np.array([1, 2, 3]) Y = np.array([1, 2, 3]) Z = np.array([1, 2, 3]) fig = plt.figure() ax = fig.gca(projection='3d') surface = ax.plot_surface(X, Y, Z, linewidth=0, antialiased=False) plt.show()
The output when running this code is as follows
Warning (from warnings module): File "C:\Users\xcent\Desktop\code.py", line 10 ax = fig.gca(projection='3d') MatplotlibDeprecationWarning: Calling gca() with keyword arguments was deprecated in Matplotlib 3.4. Starting two minor releases later, gca() will take no keyword arguments. The gca() function should only be used to get the current axes, or if no axes exist, create new axes with default keyword arguments. To create a new axes with non-default arguments, use plt.axes() or plt.subplot(). Traceback (most recent call last): File "C:\Users\xcent\Desktop\code.py", line 11, in <module> surface = ax.plot_surface(X, Y, Z, linewidth=0, antialiased=False) File "C:\Users\xcent\AppData\Local\Programs\Python\Python39\lib\site-packages\matplotlib\_api\deprecation.py", line 431, in wrapper return func(*inner_args, **inner_kwargs) File "C:\Users\xcent\AppData\Local\Programs\Python\Python39\lib\site-packages\mpl_toolkits\mplot3d\axes3d.py", line 1658, in plot_surface raise ValueError("Argument Z must be 2-dimensional.") 👉 ValueError: Argument Z must be 2-dimensional.
Solution
The error message ValueError: Argument Z must be 2-dimensional.
indicates that the plot_surface
method requires a 2-dimensional array for the Z
parameter. In the code above, I’m passing a 1-dimensional array Z = np.array([1, 2, 3])
.
As for the deprecation warning, this is because the method gca()
will no longer accept keyword arguments in future versions of Matplotlib, so I should use a different method to create the axes.
Here’s a modified version of your code that should work correctly. This code creates a simple 3D surface where the Z values are simply the product of the X and Y values:
import matplotlib.pyplot as plt import numpy as np X = np.array([[1, 2, 3]]) Y = np.array([[1], [2], [3]]) Z = X * Y fig = plt.figure() ax = plt.axes(projection='3d') surface = ax.plot_surface(X, Y, Z, linewidth=0, antialiased=False) plt.show()
In the fixed code, X
, Y
, and Z
are all 2-dimensional arrays now. I made X
and Y
2D by adding an extra set of brackets around their definitions. This creates arrays with shapes (1,3)
and (3,1)
, respectively.
Shape Example: A 2D array with shape
(1,3)
is like a single row with 3 columns, containing 3 elements. An array with shape (3,1)
is like 3 rows with a single column each, a tall, thin structure with 3 elements.
When multiplied to create Z
, they are broadcasted into two dimensions to create a (3,3)
array.
Also, instead of using gca()
to create the 3D axes, I have used the plt.axes()
function with the projection='3d'
keyword argument, as suggested by the deprecation warning.
July 22, 2023 at 11:58PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
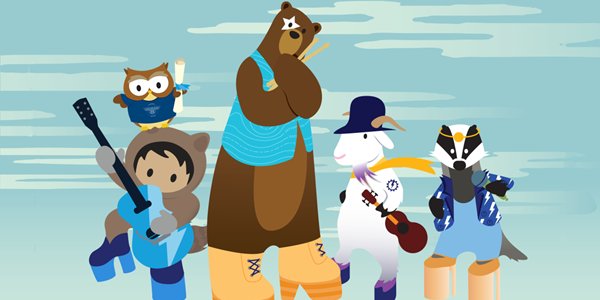
Post a Comment