Python How to Create a Category in WordPress if it Doesnt Already Exist? : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
To create a new category in WordPress using Python, you can use the WordPress REST API. Here’s a basic function that checks if a category already exists and creates it if it doesn’t:
import requests import json # Your WordPress site URL site_url = 'http://your-site-url' # Your WordPress username username = 'your-username' # The application password you generated password = 'your-application-password' def create_category(category_name): # Check if the category already exists url = f'{site_url}/wp-json/wp/v2/categories?search={category_name}' response = requests.get(url, auth=(username, password)) if response.status_code == 200: categories = json.loads(response.text) for category in categories: if category['name'].lower() == category_name.lower(): print(f"Category '{category_name}' already exists") return category['id'] # The category doesn't exist, so create it url = f'{site_url}/wp-json/wp/v2/categories' data = { 'name': category_name } response = requests.post(url, auth=(username, password), json=data) if response.status_code == 201: category = json.loads(response.text) print(f"Category '{category_name}' created successfully") return category['id'] else: print(f"Failed to create category '{category_name}': {response.text}") return None # Test the function category_id = create_category('My New Category')
In this code, replace 'http://your-site-url'
, 'your-username'
, and 'your-application-password'
with your WordPress site URL, your WordPress username, and the application password you generated.
This function first sends a GET request to search for categories with the specified name. If it finds a match, it prints a message and returns the ID of the existing category.
If it doesn’t find a match, it sends a POST request to create a new category with the specified name, then prints a message and returns the ID of the new category.
You can then use the returned category ID when creating a post to assign the post to the category.
Here’s an example:
data = { 'title': 'My New Post', 'content': 'This is the content of my new post.', 'categories': [category_id], # Assign the post to the category 'status': 'publish' } response = requests.post(f'{site_url}/wp-json/wp/v2/posts', auth=(username, password), json=data)
Note: You should only use Basic Auth over HTTPS in a production environment. For a more secure method, you should look into using a more secure authentication method such as OAuth.
When I tried this, I got the following error:
{"code":"rest_cannot_create","message":"Sorry, you are not allowed to create terms in this taxonomy.","data":{"status":403}}
In this case, you need to set your role to “Editor
” or “Administrator
” because only those two roles have the right to set categories in WordPress:
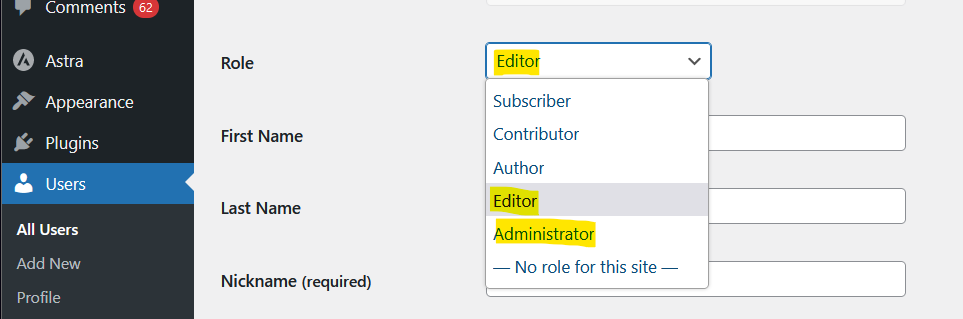
If you’re interested in becoming a six-figure programmer online, check out the following tutorial on the Finxter blog:
Recommended: How to Become a Six-Figure Coder Online
June 17, 2023 at 06:52PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
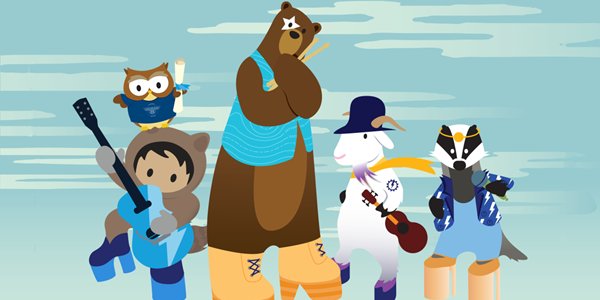
Post a Comment