Python for Beginners: Pandas Assign New Columns to a DataFrame :
by:
blow post content copied from Planet Python
click here to view original post
Pandas dataframes are the data structures that we use to handle tabular data in python. This article discusses different ways to assign new columns to pandas dataframe using the assign()
method.
The Pandas assign() Method
The assign()
method is used to assign new columns to a pandas dataframe. It has the following syntax.
df.assign(col_1=series_1, col_2=series2,...)
In the above function, the column names and the values for the columns are passed as keyword arguments. Here, column names are the keywords, and list or series objects containing the data for the column are the corresponding values.
When we invoke the assign() method on a dataframe df, it takes column names and series objects as its input argument. After execution, the assign() method adds the specified column to the dataframe df and returns the modified dataframe.
Pandas Assign a Column to a DataFrame
To assign a new column to the pandas dataframe using the assign()
method, we will pass the column name as its input argument and the values in the column as the value assigned to the column name parameter.
After execution, the assign()
method returns the modified dataframe. You can observe this in the following example.
import pandas as pd
myDicts=[{"Roll":1,"Maths":100, "Physics":80, "Chemistry": 90},
{"Roll":2,"Maths":80, "Physics":100, "Chemistry": 90},
{"Roll":3,"Maths":90, "Physics":80, "Chemistry": 70},
{"Roll":4,"Maths":100, "Physics":100, "Chemistry": 90},
{"Roll":5,"Maths":90, "Physics":90, "Chemistry": 80},
{"Roll":6,"Maths":80, "Physics":70, "Chemistry": 70}]
df=pd.DataFrame(myDicts)
print("The original dataframe is:")
print(df)
df=df.assign(Name= ["Aditya","Joel", "Sam", "Chris", "Riya", "Anne"])
print("The mofified dataframe is:")
print(df)
Output:
The original dataframe is:
Roll Maths Physics Chemistry
0 1 100 80 90
1 2 80 100 90
2 3 90 80 70
3 4 100 100 90
4 5 90 90 80
5 6 80 70 70
The mofified dataframe is:
Roll Maths Physics Chemistry Name
0 1 100 80 90 Aditya
1 2 80 100 90 Joel
2 3 90 80 70 Sam
3 4 100 100 90 Chris
4 5 90 90 80 Riya
5 6 80 70 70 Anne
In the above example, we created a column "Name"
in the input dataframe using a list and the assign()
method.
Instead of a list, we can also assign a pandas series to the column name parameter to create a new column in the dataframe as shown below.
import pandas as pd
myDicts=[{"Roll":1,"Maths":100, "Physics":80, "Chemistry": 90},
{"Roll":2,"Maths":80, "Physics":100, "Chemistry": 90},
{"Roll":3,"Maths":90, "Physics":80, "Chemistry": 70},
{"Roll":4,"Maths":100, "Physics":100, "Chemistry": 90},
{"Roll":5,"Maths":90, "Physics":90, "Chemistry": 80},
{"Roll":6,"Maths":80, "Physics":70, "Chemistry": 70}]
df=pd.DataFrame(myDicts)
print("The original dataframe is:")
print(df)
df=df.assign(Name= pd.Series(["Aditya","Joel", "Sam", "Chris", "Riya", "Anne"]))
print("The mofified dataframe is:")
print(df)
Output:
The original dataframe is:
Roll Maths Physics Chemistry
0 1 100 80 90
1 2 80 100 90
2 3 90 80 70
3 4 100 100 90
4 5 90 90 80
5 6 80 70 70
The mofified dataframe is:
Roll Maths Physics Chemistry Name
0 1 100 80 90 Aditya
1 2 80 100 90 Joel
2 3 90 80 70 Sam
3 4 100 100 90 Chris
4 5 90 90 80 Riya
5 6 80 70 70 Anne
In this example, we passed a series to the assign()
method as an input argument instead of a list. However, you can observe that the output dataframe in this example is the same as the previous example.
Assign a Column Based on Another Column
We can also create a column based on another column in a pandas dataframe. For this, we will first create a series based on another column. Then, we can use the assign()
method and pass the column name with the series as its input to assign the column to the pandas dataframe.
You can observe this in the following example.
import pandas as pd
myDicts=[{"Roll":1,"Maths":100, "Physics":80, "Chemistry": 90},
{"Roll":2,"Maths":80, "Physics":100, "Chemistry": 90},
{"Roll":3,"Maths":90, "Physics":80, "Chemistry": 70},
{"Roll":4,"Maths":100, "Physics":100, "Chemistry": 90},
{"Roll":5,"Maths":90, "Physics":90, "Chemistry": 80},
{"Roll":6,"Maths":80, "Physics":70, "Chemistry": 70}]
df=pd.DataFrame(myDicts)
print("The original dataframe is:")
print(df)
df=df.assign(GPI= df["Maths"]/10)
print("The mofified dataframe is:")
print(df)
Output:
The original dataframe is:
Roll Maths Physics Chemistry
0 1 100 80 90
1 2 80 100 90
2 3 90 80 70
3 4 100 100 90
4 5 90 90 80
5 6 80 70 70
The mofified dataframe is:
Roll Maths Physics Chemistry GPI
0 1 100 80 90 10.0
1 2 80 100 90 8.0
2 3 90 80 70 9.0
3 4 100 100 90 10.0
4 5 90 90 80 9.0
5 6 80 70 70 8.0
In this example, we have created the GPI
column using the "Maths"
column. For this, we created a series by dividing the Maths
column by 10. Then, we assigned the new series to the GPI
keyword as an input argument in the assign()
method. You can also use the pandas apply method to create a new series in this case.
Assign Multiple Columns to a DataFrame
To assign multiple columns to the pandas dataframe, you can use the assign()
method as shown below.
import pandas as pd
myDicts=[{"Roll":1,"Maths":100, "Physics":80, "Chemistry": 90},
{"Roll":2,"Maths":80, "Physics":100, "Chemistry": 90},
{"Roll":3,"Maths":90, "Physics":80, "Chemistry": 70},
{"Roll":4,"Maths":100, "Physics":100, "Chemistry": 90},
{"Roll":5,"Maths":90, "Physics":90, "Chemistry": 80},
{"Roll":6,"Maths":80, "Physics":70, "Chemistry": 70}]
df=pd.DataFrame(myDicts)
print("The original dataframe is:")
print(df)
df=df.assign(Name=["Aditya","Joel", "Sam", "Chris", "Riya", "Anne"],GPI= df["Maths"]/10)
print("The mofified dataframe is:")
print(df)
Output:
he original dataframe is:
Roll Maths Physics Chemistry
0 1 100 80 90
1 2 80 100 90
2 3 90 80 70
3 4 100 100 90
4 5 90 90 80
5 6 80 70 70
The mofified dataframe is:
Roll Maths Physics Chemistry Name GPI
0 1 100 80 90 Aditya 10.0
1 2 80 100 90 Joel 8.0
2 3 90 80 70 Sam 9.0
3 4 100 100 90 Chris 10.0
4 5 90 90 80 Riya 9.0
5 6 80 70 70 Anne 8.0
In this example, we assigned two columns to the pandas dataframe using the assign()
method. For this, we passed both the column names and their values as keyword arguments to the assign()
method.
Conclusion
In this article, we have discussed different ways to assign columns to a pandas dataframe. To learn more about python programming, you can read this article on how to use the insert()
method to insert a column into a dataframe. You might also like this article on how to convert epoch to datetime in python.
I hope you enjoyed reading this article. Stay tuned for more informative articles.
Happy Learning!
The post Pandas Assign New Columns to a DataFrame appeared first on PythonForBeginners.com.
March 08, 2023 at 07:33PM
Click here for more details...
=============================
The original post is available in Planet Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
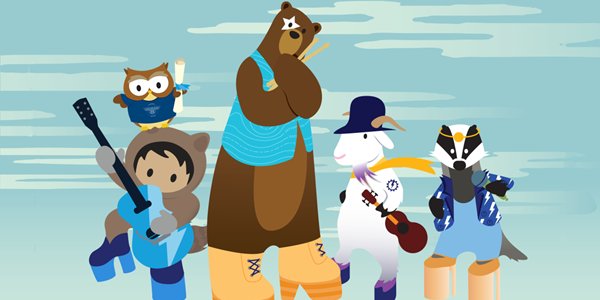
Post a Comment