How to Access the First, Second, or N-th Child Div Element in BeautifulSoup? : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
To access the first, second, or N-th child div element in BeautifulSoup, use the .contents
or .find_all()
methods on a parent div element. The .contents
method returns a list of children, including tags and strings, while .find_all()
returns a list of matching tags only. Simply select the desired index to obtain the child div element you need.
In Beautiful Soup, you can navigate to the first, second, or third div
within a parent div
using the .contents
or .find_all()
methods.
Here’s an example:
from bs4 import BeautifulSoup html = """ <div id="parent-div"> <div class="child-div">First child div</div> <div class="child-div">Second child div</div> <div class="child-div">Third child div</div> </div> """ soup = BeautifulSoup(html, 'html.parser') # Find the parent div parent_div = soup.find('div', {'id': 'parent-div'}) # Method 1: Using .contents first_child_div = parent_div.contents[1] second_child_div = parent_div.contents[3] third_child_div = parent_div.contents[5] print("Using .contents:") print("First child div:", first_child_div.text) print("Second child div:", second_child_div.text) print("Third child div:", third_child_div.text) # Method 2: Using .find_all() all_child_divs = parent_div.find_all('div', {'class': 'child-div'}) print("\nUsing .find_all():") print("First child div:", all_child_divs[0].text) print("Second child div:", all_child_divs[1].text) print("Third child div:", all_child_divs[2].text)
The output of this script is:
Using .contents:
First child div: First child div
Second child div: Second child div
Third child div: Third child div
Using .find_all():
First child div: First child div
Second child div: Second child div
Third child div: Third child div
Note:
The .contents
solution returns a list of the parent element’s children, including tags and strings. Note that the indexing numbers are shifted using this solution, i.e., the first element is indexed using .contents[1]
, the second with .content[3]
, and the n
-th with .contents[2*n-1]
.
The .find_all()
solution returns a list of matching tags only.
You can use either method to navigate to the first, second, or third div
within a parent div
.
Keep Learning
If you want to learn BeautifulSoup from scratch, I’d recommend you check out our academy course:
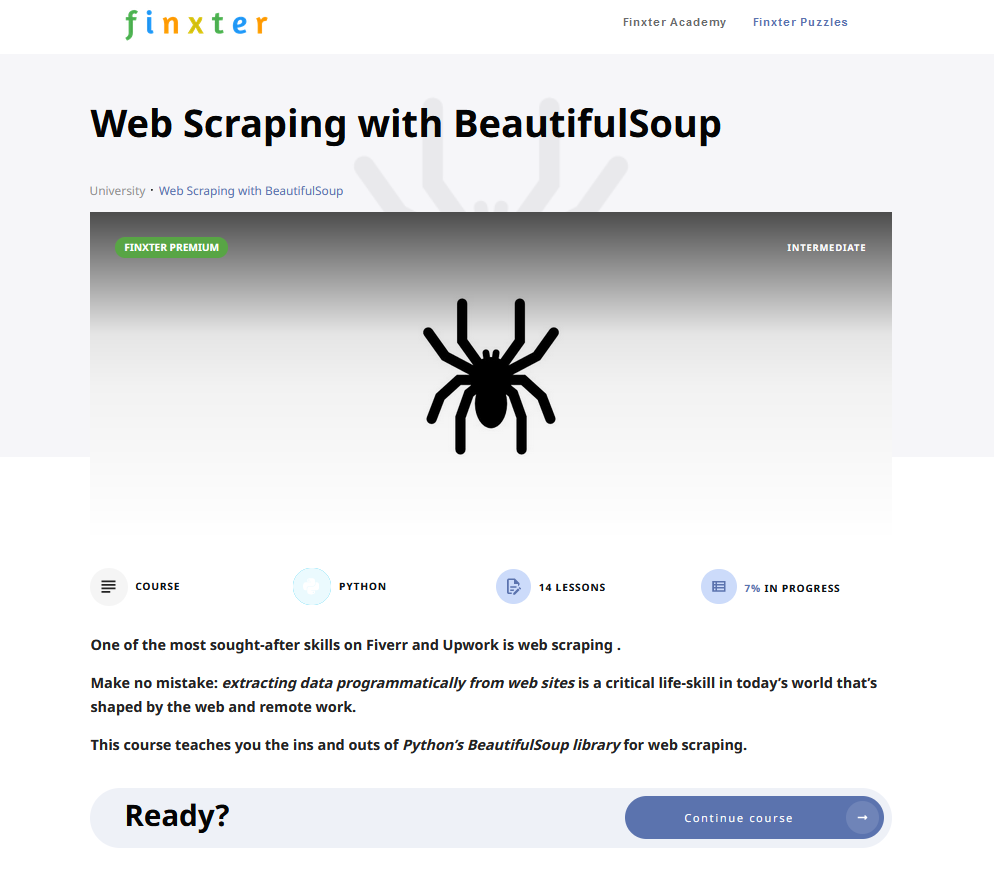
March 21, 2023 at 04:33PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
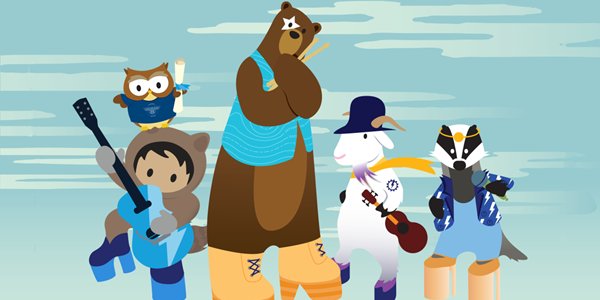
Post a Comment