I Used This Python Script to Rename All Files in Subfolders (Recursive) : Chris
by: Chris
blow post content copied from Finxter
click here to view original post
I’m currently working on a website project for the Finxter ecosystem, where I’m given a folder with subfolders (and subfolders within) that contain image files.
Example folder structure:
folder
– subfolder1
-- – image1.jpg
-- – image2.jpg
-- – image3.jpg
– subfolder2
----image1.jpg
----image2.jpg
Across the subfolders, the files may have the same name, which causes an issue when uploading all files to my webserver. To fix all issues, I want to rename all files, so they have unique names.
Project Goal: My simple idea was to add a prefix with the folder structure in front of each file name. For example, a file named
'myfile1.jpg'
in folder 'myfolder1'
will be renamed to the new file 'myfolder1_myfile1.jpg'
. All the files with new names should be copied into a subfolder as specified in a destination
variable.
Desired output folder structure:
destination
-- – subfolder1_image1.jpg
-- – subfolder1_image2.jpg
-- – subfolder1_image3.jpg
-- – subfolder2_image1.jpg
-- – subfolder2_image2.jpg

Here’s the code to accomplish this — I’ll explain it in a minute!
# import os and sys modules import os, sys import shutil # get the root directory from command line argument root_dir = '.' destination = './my_destination' # walk through the directory tree for dirname, subdirs, files in os.walk(root_dir): # for each directory, print the directory's path print(dirname) # for each file in the directory, copy and rename for file in files: new_name = dirname[2:].replace('\\', '_') + "_" + file shutil.copy(os.path.join(dirname, file), os.path.join(destination, new_name))
You need to give absolute or relative paths from the perspective of this Python file. It’ll already run fine if the Python file is stored in the same folder as the subfolders. But you need to have a subfolder called my_destination
.
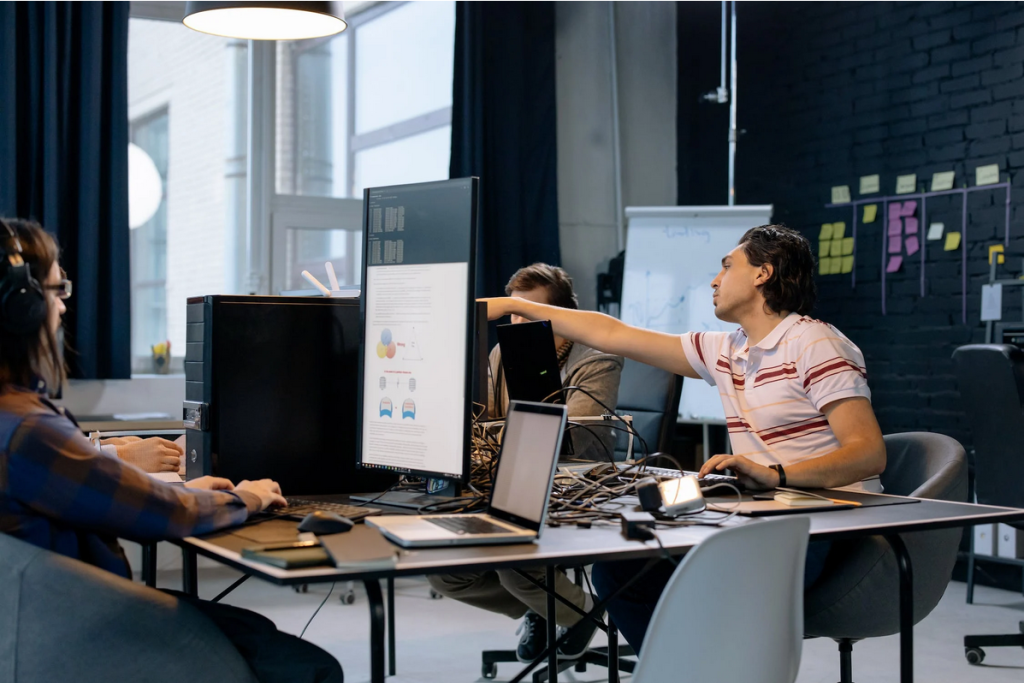
How does this code work?
This code recursively copies all files in a directory tree to a specified destination folder.
You take the root and destination directories and iterate through each directory and subdirectory within the root directory. For each directory, you print out the directory’s path.
For each file in the directory, you copy it to the specified destination folder, renaming it to include the path of the original directory in the name.
I used string slicing and the string.replace()
method to modify the destination filenames according to my goals, see the following line:
new_name = dirname[2:].replace('\\', '_') + "_" + file
Note that the double backslash \\
is actually an (escaped) single backslash character that you usually don’t want in your new filename. We replace all of this with single underscores such that a file myDir\myFile
becomes myDir_myFile
.
January 09, 2023 at 11:05PM
Click here for more details...
=============================
The original post is available in Finxter by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
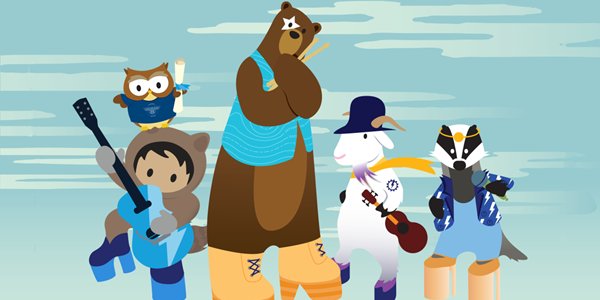
Post a Comment