Python for Beginners: List of Dictionaries to CSV in Python :
by:
blow post content copied from Planet Python
click here to view original post
We use dictionaries in python to store key-value pairs. Similarly, we use a CSV file to store records that contain values for specific fields. In this article, we will discuss how we can convert a list of dictionaries to a CSV file in python.
List of Dictionaries to CSV in Python using csv.writer()
The csv module provides us with different methods to perform various operations on a CSV file. To convert a list of dictionaries to csv in python, we can use the csv.writer()
method along with the csv.writerow()
method. For this, we will use the following steps.
- First, we will open a csv file in write mode using the
open()
function. Theopen()
function takes the file name as the first input argument and the literal “w” as the second input argument to show that the file will be opened in the write mode. It returns a file object that contains the empty csv file created by theopen()
function. - After opening the file, we will create a
csv.writer
object using thecsv.writer()
method. Thecsv.writer()
method takes the file object as an input argument and returns a writer object. Once the writer object is created, we can add data from the list of dictionaries to the csv file using thecsv.writerow()
method. - The
csv.writerow()
method, when invoked on a writer object, takes a list of values and adds it to the csv file referred by the writer object. - First, we will add the header for the CSV file by adding the keys of a dictionary to the csv file.
- After adding the header, we will use a for loop with the
writerow()
method to add each dictionary to the csv file. Here, we will pass the values in the dictionary to the CSV file.
After execution of the for loop, the data from the python dictionary will be added to the CSV file. To save the data, you should close the file using the close()
method. Otherwise, no changes will be saved to the csv file.
The source code to convert a list of dictionaries to a csv file using the csv.writer()
method is as follows.
import csv
listOfDict = [{'Name': 'Aditya', 'Roll': 1, 'Language': 'Python'}, {'Name': 'Sam', 'Roll': 2, 'Language': 'Java'},
{'Name': 'Chris', 'Roll': 3, 'Language': 'C++'}, {'Name': 'Joel', 'Roll': 4, 'Language': 'TypeScript'}]
print("THe list of dictionaries is:")
print(listOfDict)
myFile = open('demo_file.csv', 'w')
writer = csv.writer(myFile)
writer.writerow(['Name', 'Roll', 'Language'])
for dictionary in listOfDict:
writer.writerow(dictionary.values())
myFile.close()
myFile = open('demo_file.csv', 'r')
print("The content of the csv file is:")
print(myFile.read())
myFile.close()
Output:
THe list of dictionaries is:
[{'Name': 'Aditya', 'Roll': 1, 'Language': 'Python'}, {'Name': 'Sam', 'Roll': 2, 'Language': 'Java'}, {'Name': 'Chris', 'Roll': 3, 'Language': 'C++'}, {'Name': 'Joel', 'Roll': 4, 'Language': 'TypeScript'}]
The content of the csv file is:
Name,Roll,Language
Aditya,1,Python
Sam,2,Java
Chris,3,C++
Joel,4,TypeScript
List of Dictionaries to CSV in Python using csv.DictWriter()
Instead of using the iterative method to add each dictionary to the csv file, we can convert the entire list of dictionaries to a CSV file at once. For this, we will use the DictWriter()
method and the csv.writerows()
method.
This approach differs from the previous method in the following steps.
- Instead of creating a csv.writer object, we will create a
csv.DictWriter
object using theDictwriter()
method. TheDictWriter()
method takes the file object containing the csv file as its first argument and the name of the columns of the csv file as the second input argument. After execution, it returns aDictWriter
object. - After creating the DictWriter object, we will add the header to the csv file. For this, we will use the
writeheader()
method. Thewriteheader()
method, when executed on aDictWriter
object, adds the columns provided to theDictWriter()
method as the header in the csv file. - After adding the header, we can add the entire list of dictionaries to the csv file using the
writerows()
method. Thewriterows()
method, when invoked on aDictWriter
object, takes a list of dictionaries as its input argument and adds the values in the dictionaries to the csv file.
After adding the entire list of dictionaries to the csv file, you must close the file using the close()
method. Otherwise, no changes will be saved.
The source code for converting a list of dictionaries to csv file in python is given below.
import csv
listOfDict = [{'Name': 'Aditya', 'Roll': 1, 'Language': 'Python'}, {'Name': 'Sam', 'Roll': 2, 'Language': 'Java'},
{'Name': 'Chris', 'Roll': 3, 'Language': 'C++'}, {'Name': 'Joel', 'Roll': 4, 'Language': 'TypeScript'}]
print("THe list of dictionaries is:")
print(listOfDict)
myFile = open('demo_file.csv', 'w')
writer = csv.DictWriter(myFile, fieldnames=['Name', 'Roll', 'Language'])
writer.writeheader()
writer.writerows(listOfDict)
myFile.close()
myFile = open('demo_file.csv', 'r')
print("The content of the csv file is:")
print(myFile.read())
myFile.close()
Output:
THe list of dictionaries is:
[{'Name': 'Aditya', 'Roll': 1, 'Language': 'Python'}, {'Name': 'Sam', 'Roll': 2, 'Language': 'Java'}, {'Name': 'Chris', 'Roll': 3, 'Language': 'C++'}, {'Name': 'Joel', 'Roll': 4, 'Language': 'TypeScript'}]
The content of the csv file is:
Name,Roll,Language
Aditya,1,Python
Sam,2,Java
Chris,3,C++
Joel,4,TypeScript
Conclusion
In this article, we have discussed two approaches to convert a list of dictionaries to csv file in python. In these approaches, each dictionary will be added to the csv file irrespective of whether it has the same number of items as compared to the columns in the csv file or it has the same keys as compared to the column names in the csv file. Thus it is advised to make sure that each dictionary should have the same number of items. Also, You should make sure that the order of keys present in the dictionaries should be same. Otherwise, the data appended to the csv file will become inconsistent and will lead to errors.
To know more about dictionaries in python, you can read this article on dictionary comprehension in python. You might also like this article on list comprehension in python.
The post List of Dictionaries to CSV in Python appeared first on PythonForBeginners.com.
July 18, 2022 at 06:30PM
Click here for more details...
=============================
The original post is available in Planet Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
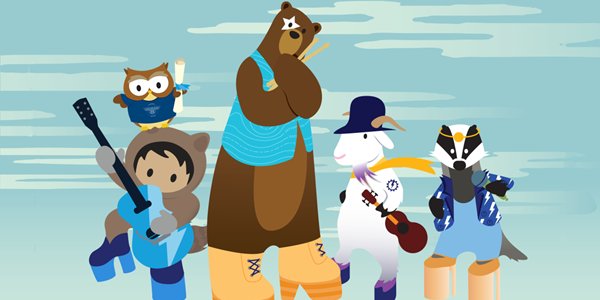
Post a Comment