Python while Loops: Repeating Tasks Conditionally :
by:
blow post content copied from Real Python
click here to view original post
Python’s while
loop enables you to execute a block of code repeatedly as long as a given condition remains true. Unlike for
loops, which iterate a known number of times, while
loops are ideal for situations where the number of iterations isn’t known upfront.
Loops are a pretty useful construct in Python, so learning how to write and use them is a great skill for you as a Python developer.
By the end of this tutorial, you’ll understand that:
while
is a Python keyword used to initiate a loop that repeats a block of code as long as a condition is true.- A
while
loop works by evaluating a condition at the start of each iteration. If the condition is true, then the loop executes. Otherwise, it terminates. while
loops are useful when the number of iterations is unknown, such as waiting for a condition to change or continuously processing user input.while True
in Python creates an infinite loop that continues until abreak
statement or external interruption occurs.- Python lacks a built-in do-while loop, but you can emulate it using a
while True
loop with abreak
statement for conditional termination.
With this knowledge, you’re prepared to write effective while
loops in your Python programs, handling a wide range of iteration needs.
Get Your Code: Click here to download the free sample code that shows you how to work with while loops in Python.
Take the Quiz: Test your knowledge with our interactive “Python while Loops: Repeating Tasks Conditionally” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Python while Loops: Repeating Tasks ConditionallyIn this quiz, you'll test your understanding of Python's while loop. This loop allows you to execute a block of code repeatedly as long as a given condition remains true. Understanding how to use while loops effectively is a crucial skill for any Python developer.
Getting Started With Python while
Loops
In programming, loops are control flow statements that allow you to repeat a given set of operations a number of times. In practice, you’ll find two main types of loops:
for
loops are mostly used to iterate a known number of times, which is common when you’re processing data collections with a specific number of data items.while
loops are commonly used to iterate an unknown number of times, which is useful when the number of iterations depends on a given condition.
Python has both of these loops and in this tutorial, you’ll learn about while
loops. In Python, you’ll generally use while
loops when you need to repeat a series of tasks an unknown number of times.
Python while
loops are compound statements with a header and a code block that runs until a given condition becomes false. The basic syntax of a while
loop is shown below:
while condition:
<body>
In this syntax, condition
is an expression that the loop evaluates for its truth value. If the condition is true, then the loop body runs. Otherwise, the loop terminates. Note that the loop body can consist of one or more statements that must be indented properly.
Here’s a more detailed breakdown of this syntax:
while
is the keyword that initiates the loop header.condition
is an expression evaluated for truthiness that defines the exit condition.<body>
consists of one or more statements to execute in each iteration.
Here’s a quick example of how you can use a while
loop to iterate over a decreasing sequence of numbers:
>>> number = 5
>>> while number > 0:
... print(number)
... number -= 1
...
5
4
3
2
1
In this example, number > 0
is the loop condition. If this condition returns a false value, the loop terminates. The body consists of a call to print()
that displays the value on the screen. Next, you decrease the value of number
. This change will produce a different result when the loop evaluates the condition in the next iteration.
The loop runs while the condition remains true. When the condition turns false, the loop terminates, and the program execution proceeds to the first statement after the loop body. In this example, the loop terminates when number
reaches a value less than or equal to 0
.
If the loop condition doesn’t become false, then you have a potentially infinite loop. Consider the following loop, and keep your fingers near the Ctrl+C key combination to terminate its execution:
>>> number = 5
>>> while number != 0:
... print(number)
... number -= 1
...
5
4
3
2
1
>>> number = 5
>>> while number != 0:
... print(number)
... number -= 2
...
5
3
1
-1
-3
-5
-7
-9
-11
Traceback (most recent call last):
...
KeyboardInterrupt
In this example, the loop condition is number != 0
. This condition works when you decrease number
by 1
. However, if you decrease it by 2, the condition may never become false, resulting in a potentially infinite loop. In such cases, you can usually terminate the loop by pressing Ctrl+C, which raises a KeyboardInterrupt
exception on most operating systems.
Note that the while
loop checks its condition first before anything else happens. If it’s false to start with, then the loop body will never run:
>>> number = 0
>>> while number > 0:
... print(number)
... number -= 1
...
Read the full article at https://realpython.com/python-while-loop/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
March 03, 2025 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
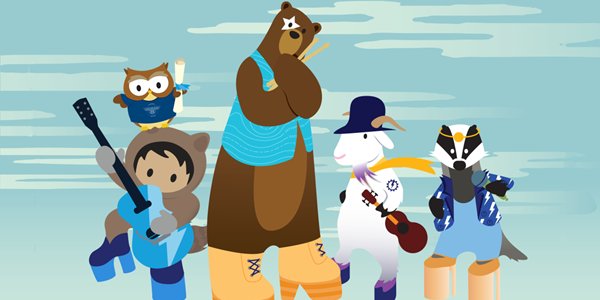
Post a Comment