How to Use sorted() and .sort() in Python
by:
blow post content copied from Real Python
click here to view original post
Sorting in Python is a fundamental task that you can accomplish using sorted()
and .sort()
. The sorted()
function returns a new sorted list from the elements of any iterable, without modifying the original iterable. On the other hand, the .sort()
method modifies a list in place and doesn’t return a value. Both methods support customization through optional keyword arguments like key
and reverse
.
By the end of this tutorial, you’ll understand that:
- You can sort any iterable with the
sorted()
function. - The
sorted()
function returns a new sorted list. - The
.sort()
method sorts the list in place. - You sort items in descending order by setting the
reverse
argument toTrue
. - The
key
argument accepts a function to customize the sort order.
In this tutorial, you’ll learn how to sort various types of data in different data structures, customize the order, and work with two different ways of sorting in Python. You’ll need a basic understanding of lists and tuples as well as sets. These are the data structures you’ll be using to perform some basic operations.
Get Your Cheat Sheet: Click here to download a free cheat sheet that summarizes how to use sorted() and .sort() in Python.
Take the Quiz: Test your knowledge with our interactive “How to Use sorted() and .sort() in Python” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
How to Use sorted() and .sort() in PythonIn this quiz, you'll test your understanding of sorting in Python using sorted() and .sort(). You'll revisit how to sort various types of data in different data structures, customize the order, and work with two different ways of sorting in Python.
Ordering Values With sorted()
In Python, you can sort iterables with the sorted()
built-in function. To get started, you’ll work with iterables that contain only one data type.
Sorting Numbers
You can use sorted()
to sort a list in Python. In this example, a list of integers is defined, and then sorted()
is called with the numbers
variable as the argument:
>>> numbers = [6, 9, 3, 1]
>>> sorted(numbers)
[1, 3, 6, 9]
>>> numbers
[6, 9, 3, 1]
The output from this code is a new, sorted list. When the original variable is printed, the initial values are unchanged.
This example shows four important characteristics of sorted()
:
- You don’t have to define the
sorted()
function. It’s a built-in function that’s available in any standard installation of Python. - You’re ordering the values in
numbers
from smallest to largest when you callsorted(numbers)
. When you pass no additional arguments or parameters,sorted()
orders the values innumbers
in ascending order. - You don’t change the original
numbers
variable becausesorted()
provides sorted output and doesn’t update the original value in place. - You get an ordered list as a return value when you call
sorted()
.
These points mean that sorted()
can be used on a list, and the output can immediately be assigned to a variable:
>>> numbers = [6, 9, 3, 1]
>>> numbers_sorted = sorted(numbers)
>>> numbers_sorted
[1, 3, 6, 9]
>>> numbers
[6, 9, 3, 1]
In this example, a new variable called numbers_sorted
now stores the output of the sorted()
function.
You can confirm all of these observations by calling help()
on sorted()
:
>>> help(sorted)
Help on built-in function sorted in module builtins:
sorted(iterable, /, *, key=None, reverse=False)
Return a new list containing all items from the iterable in ascending order.
A custom key function can be supplied to customize the sort order, and the
reverse flag can be set to request the result in descending order.
You’ll cover the optional arguments key
and reverse
later in the tutorial.
The first parameter of sorted()
is an iterable. That means that you can use sorted()
on tuples and sets very similarly:
>>> numbers_tuple = (6, 9, 3, 1)
>>> sorted(numbers_tuple)
[1, 3, 6, 9]
>>> numbers_set = {5, 10, 1, 0}
>>> sorted(numbers_set)
[0, 1, 5, 10]
Notice how even though the input was a set and a tuple, the output is a list because sorted()
returns a new list by definition. The returned object can be cast to a new type if it needs to match the input type. Be careful if attempting to cast the resulting list back to a set, as a set by definition is unordered:
>>> numbers_tuple = (6, 9, 3, 1)
>>> numbers_set = {5, 10, 1, 0}
>>> numbers_tuple_sorted = sorted(numbers_tuple)
>>> numbers_set_sorted = sorted(numbers_set)
>>> numbers_tuple_sorted
[1, 3, 6, 9]
>>> numbers_set_sorted
[0, 1, 5, 10]
>>> tuple(numbers_tuple_sorted)
(1, 3, 6, 9)
>>> set(numbers_set_sorted)
{0, 1, 10, 5}
When you cast the numbers_set_sorted
value to a set
, it’s unordered, as expected. If you’re curious about how sets work in Python, then you can check out the tutorial Sets in Python.
Read the full article at https://realpython.com/python-sort/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
February 24, 2025 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
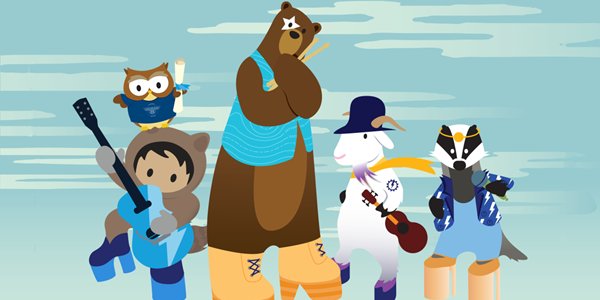
Post a Comment