Python Set Comprehensions: How and When to Use Them
by:
blow post content copied from Real Python
click here to view original post
Python set comprehensions provide a concise way to create and manipulate sets in your code. They generate sets with a clean syntax, making your code more readable and Pythonic. With set comprehensions, you can create, transform, and filter sets, which are great skills to add to your Python programming toolkit.
In this tutorial, you’ll learn the syntax and use cases of set comprehensions, ensuring you can decide when and how to use them in your code. Understanding set comprehensions will help you write cleaner, more efficient Python code.
By the end of this tutorial, you’ll understand that:
- Python has set comprehensions, which allow you to create sets with a concise syntax.
- Python has four types of comprehensions: list, set, dictionary, and generator expressions.
- A set comprehension can be written as
{expression for item in iterable [if condition]}
. - Sets can’t contain duplicates, as they ensure that all their elements are unique.
To get the most out of this tutorial, you should be familiar with basic Python concepts such as for
loops, iterables, list comprehensions, and dictionary comprehensions.
Get Your Code: Click here to download the free sample code that you’ll use to learn about Python set comprehensions.
Take the Quiz: Test your knowledge with our interactive “Python Set Comprehensions: How and When to Use Them” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Python Set Comprehensions: How and When to Use ThemIn this quiz, you'll test your understanding of Python set comprehensions. Set comprehensions are a concise and quick way to create, transform, and filter sets in Python. They can significantly enhance your code's conciseness and readability compared to using regular for loops to process your sets.
Creating and Transforming Sets in Python
In Python programming, you may need to create, populate, and transform sets. To do this, you can use set literals, the set()
constructor, and for
loops. In the following sections, you’ll take a quick look at how to use these tools. You’ll also learn about set comprehensions, which are a powerful way to manipulate sets in Python.
Creating Sets With Literals and set()
To create new sets, you can use literals. A set literal is a series of elements enclosed in curly braces. The syntax of a set literal is shown below:
{element_1, element_2,..., element_N}
The elements must be hashable objects. The objects in the literal might be duplicated, but only one instance will be stored in the resulting set. Sets don’t allow duplicate elements. Here’s a quick example of a set:
>>> colors = {"blue", "red", "green", "orange", "green"}
>>> colors
{'red', 'green', 'orange', 'blue'}
>>> colors.add("purple")
>>> colors
{'red', 'green', 'orange', 'purple', 'blue'}
In this example, you create a set containing color names. The elements in your resulting set are unique string objects. You can add new elements using the .add()
method. Remember that sets are unordered collections, so the order of elements in the resulting set won’t match the insertion order in most cases.
Note: To learn more about sets, check out the Sets in Python tutorial.
You can also create a new set using the set()
constructor and an iterable of objects:
>>> numbers = [2, 2, 1, 4, 2, 3]
>>> set(numbers)
{1, 2, 3, 4}
In this example, you create a new set using set()
with a list of numeric values. Note how the resulting set doesn’t contain duplicate elements. In practice, the set()
constructor is a great tool for eliminating duplicate values in iterables.
To create an empty set, you use the set()
constructor without arguments:
>>> set()
set()
You can’t create an empty set with a literal because a pair of curly braces {}
represents an empty dictionary, not a set. To create an empty set, you must use the set()
constructor.
Using for
Loops to Populate Sets
Sometimes, you need to start with an empty set and populate it with elements dynamically. To do this, you can use a for
loop. For example, say that you want to create a set of unique words from a text. Here’s how to do this with a loop:
>>> unique_words = set()
>>> text = """
... Beautiful is better than ugly
... Explicit is better than implicit
... Simple is better than complex
... Complex is better than complicated
... """.lower()
>>> for word in text.split():
... unique_words.add(word)
...
>>> unique_words
{
'beautiful',
'ugly',
'better',
'implicit',
'complicated',
'than',
'explicit',
'is',
'complex',
'simple'
}
Read the full article at https://realpython.com/python-set-comprehension/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
December 11, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
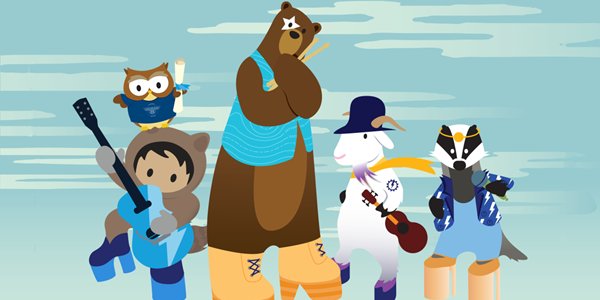
Post a Comment