Object-Oriented Programming (OOP) in Python :
by:
blow post content copied from Real Python
click here to view original post
Object-oriented programming (OOP) in Python lets you structure your code by grouping related properties and behaviors into individual objects. You create classes as blueprints and instantiate them to form objects. With OOP, you can model real-world entities and their interactions, and create complex systems with reusable components.
OOP in Python revolves around four main concepts: encapsulation, inheritance, abstraction, and polymorphism. Encapsulation bundles data with methods, inheritance lets you create subclasses, abstraction hides complex details, and polymorphism allows for different implementations.
By the end of this tutorial, you’ll understand that:
- Object-oriented programming in Python involves structuring code into classes to model real-world entities.
- The four key concepts of OOP in Python are encapsulation, inheritance, abstraction, and polymorphism.
- OOP in Python is considered straightforward to learn due to its clear syntax and readability.
- The main focus of OOP in Python involves creating classes as blueprints for objects. These objects contain data and the methods needed to manipulate that data.
You’ll explore how to define classes, instantiate classes to create objects, and leverage inheritance to build robust systems in Python.
Note: This tutorial is adapted from the chapter “Object-Oriented Programming (OOP)” in Python Basics: A Practical Introduction to Python 3.
The book uses Python’s built-in IDLE editor to create and edit Python files and interact with the Python shell, so you’ll see occasional references to IDLE throughout this tutorial. If you don’t use IDLE, you can run the example code from the editor and environment of your choice.
Get Your Code: Click here to download the free sample code that shows you how to do object-oriented programming with classes in Python 3.
Take the Quiz: Test your knowledge with our interactive “Object-Oriented Programming (OOP) in Python” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Object-Oriented Programming (OOP) in PythonObject-oriented programming (OOP) is a method of structuring a program by bundling related properties and behaviors into individual objects.
What Is Object-Oriented Programming in Python?
Object-oriented programming is a programming paradigm that provides a means of structuring programs so that properties and behaviors are bundled into individual objects.
For example, an object could represent a person with properties like a name, age, and address and behaviors such as walking, talking, breathing, and running. Or it could represent an email with properties like a recipient list, subject, and body and behaviors like adding attachments and sending.
Put another way, object-oriented programming is an approach for modeling concrete, real-world things, like cars, as well as relations between things, like companies and employees or students and teachers. OOP models real-world entities as software objects that have some data associated with them and can perform certain operations.
OOP also exists in other programming languages and is often described to center around the four pillars, or four tenants of OOP:
-
Encapsulation allows you to bundle data (attributes) and behaviors (methods) within a class to create a cohesive unit. By defining methods to control access to attributes and its modification, encapsulation helps maintain data integrity and promotes modular, secure code.
-
Inheritance enables the creation of hierarchical relationships between classes, allowing a subclass to inherit attributes and methods from a parent class. This promotes code reuse and reduces duplication.
-
Abstraction focuses on hiding implementation details and exposing only the essential functionality of an object. By enforcing a consistent interface, abstraction simplifies interactions with objects, allowing developers to focus on what an object does rather than how it achieves its functionality.
-
Polymorphism allows you to treat objects of different types as instances of the same base type, as long as they implement a common interface or behavior. Python’s duck typing make it especially suited for polymorphism, as it allows you to access attributes and methods on objects without needing to worry about their actual class.
In this tutorial you’ll take a practical approach to understanding OOP in Python. But keeping these four concepts of object-oriented programming in mind may help you to remember the information that you gather.
The key takeaway is that objects are at the center of object-oriented programming in Python. In other programming paradigms, objects only represent the data. In OOP, they additionally inform the overall structure of the program.
How Do You Define a Class in Python?
In Python, you define a class by using the class
keyword followed by a name and a colon. Then you use .__init__()
to declare which attributes each instance of the class should have:
class Employee:
def __init__(self, name, age):
self.name = name
self.age = age
But what does all of that mean? And why do you even need classes in the first place? Take a step back and consider using built-in, primitive data structures as an alternative.
Primitive data structures—like numbers, strings, and lists—are designed to represent straightforward pieces of information, such as the cost of an apple, the name of a poem, or your favorite colors, respectively. What if you want to represent something more complex?
For example, you might want to track employees in an organization. You need to store some basic information about each employee, such as their name, age, position, and the year they started working.
One way to do this is to represent each employee as a list:
kirk = ["James Kirk", 34, "Captain", 2265]
spock = ["Spock", 35, "Science Officer", 2254]
mccoy = ["Leonard McCoy", "Chief Medical Officer", 2266]
There are a number of issues with this approach.
Read the full article at https://realpython.com/python3-object-oriented-programming/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
December 15, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
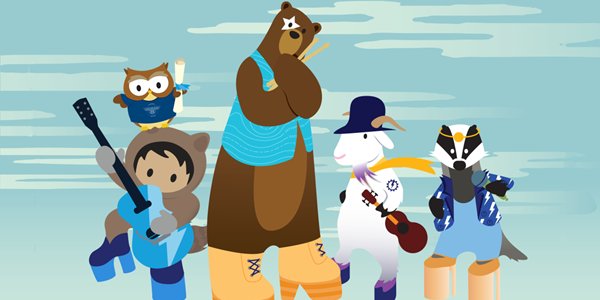
Post a Comment