Build Enumerations of Constants With Python's Enum :
by:
blow post content copied from Real Python
click here to view original post
Python’s enum
module offers a way to create enumerations, a data type allowing you to group related constants. You can define an enumeration using the Enum
class, either by subclassing it or using its functional API. This tutorial will guide you through the process of creating and using Python enums, comparing them to simple constants, and exploring specialized types like IntEnum
, IntFlag
, and Flag
.
Enumerations provide benefits over simple constants by offering a structured, readable, and maintainable way to manage sets of constant values. They ensure type safety, prevent value reassignment, and facilitate iteration and member access. By learning to utilize Python’s enum types, you enhance your ability to write organized and efficient code.
By the end of this tutorial, you’ll understand that:
- An enum in Python groups related constants in a single data type using the
Enum
class. - You create enumerations by subclassing
Enum
or using the module’s functional API. - Using
Enum
over simple constants provides structure, prevents reassignment, and enhances code readability. Enum
,IntEnum
,IntFlag
, andFlag
differ in their support for integer operations and bitwise flags.- Enums can work with data types like integers or strings, boosting their flexibility.
- You access enumeration members using dot notation, call notation, or subscript notation.
- You can iterate over enum members using loops or the
.__members__
attribute.
To follow along with this tutorial, you should be familiar with object-oriented programming and inheritance in Python.
Get Your Code: Click here to download the free sample code that you’ll use to build enumerations in Python.
Getting to Know Enumerations in Python
Several programming languages, including Java and C++, have a native enumeration or enum data type as part of their syntax. This data type allows you to create sets of named constants, which are considered members of the containing enum. You can access the members through the enumeration itself.
Enumerations come in handy when you need to define an immutable and discrete set of similar or related constant values that may or may not have semantic meaning in your code.
Days of the week, months and seasons of the year, Earth’s cardinal directions, a program’s status codes, HTTP status codes, colors in a traffic light, and pricing plans of a web service are all great examples of enumerations in programming. In general, you can use an enum whenever you have a variable that can take one of a limited set of possible values.
Python doesn’t have an enum data type as part of its syntax. Fortunately, Python 3.4 added the enum
module to the standard library. This module provides the Enum
class for supporting general-purpose enumerations in Python.
Enumerations were introduced by PEP 435, which defines them as follows:
An enumeration is a set of symbolic names bound to unique, constant values. Within an enumeration, the values can be compared by identity, and the enumeration itself can be iterated over. (Source)
Before this addition to the standard library, you could create something similar to an enumeration by defining a sequence of similar or related constants. To this end, Python developers often used the following idiom:
>>> RED, GREEN, YELLOW = range(3)
>>> RED
0
>>> GREEN
1
Even though this idiom works, it doesn’t scale well when you’re trying to group a large number of related constants. Another inconvenience is that the first constant will have a value of 0
, which is falsy in Python. This can be an issue in certain situations, especially those involving Boolean tests.
Note: If you’re using a Python version before 3.4, then you can create enumerations by installing the enum34 library, which is a backport of the standard-library enum
. The aenum third-party library could be an option for you as well.
In most cases, enumerations can help you avoid the drawbacks of the above idiom. They’ll also help you produce more organized, readable, and robust code. Enumerations have several benefits, some of which relate to ease of coding:
- Allowing for conveniently grouping related constants in a sort of namespace
- Allowing for additional behavior with custom methods that operate on either enum members or the enum itself
- Providing quick and flexible access to enum members
- Enabling direct iteration over members, including their names and values
- Facilitating code completion within IDEs and editors
- Enabling type and error checking with static checkers
- Providing a hub of searchable names
- Mitigating spelling mistakes when using the members of an enumeration
They also make your code robust by providing the following benefits:
- Ensuring constant values that can’t be changed during the code’s execution
- Guaranteeing type safety by differentiating the same value shared across several enums
- Improving readability and maintainability by using descriptive names instead of mysterious values or magic numbers
- Facilitating debugging by taking advantage of readable names instead of values with no explicit meaning
- Providing a single source of truth and consistency throughout the code
Now that you know the basics of enumerations in programming and in Python, you can start creating your own enum types by using Python’s Enum
class.
Creating Enumerations With Python’s Enum
Python’s enum
module provides the Enum
class, which allows you to create enumeration types. To create your own enumerations, you can either subclass Enum
or use its functional API. Both options will let you define a set of related constants as enum members.
In the following sections, you’ll learn how to create enumerations in your code using the Enum
class. You’ll also learn how to set automatically generated values for your enums and how to create enumerations containing alias and unique values. To kick things off, you’ll start by learning how to create an enumeration by subclassing Enum
.
Read the full article at https://realpython.com/python-enum/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
December 15, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
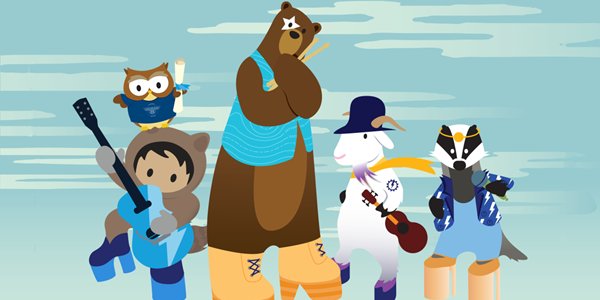
Post a Comment