Lists vs Tuples in Python :
by:
blow post content copied from Real Python
click here to view original post
In Python, lists and tuples are versatile and useful data types that allow you to store data in a sequence. You’ll find them in virtually every nontrivial Python program. Learning about them is a core skill for you as a Python developer.
In this tutorial, you’ll:
- Get to know lists and tuples
- Explore the core characteristics of lists and tuples
- Learn how to define and manipulate lists and tuples
- Decide when to use lists or tuples in your code
To get the most out of this tutorial, you should know the basics of Python programming, including how to define variables.
Get Your Code: Click here to download the free sample code that shows you how to work with lists and tuples in Python.
Take the Quiz: Test your knowledge with our interactive “Lists vs Tuples in Python” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Lists vs Tuples in PythonChallenge yourself with this quiz to evaluate and deepen your understanding of Python lists and tuples. You'll explore key concepts, such as how to create, access, and manipulate these data types, while also learning best practices for using them efficiently in your code.
Getting Started With Python Lists and Tuples
In Python, a list is a collection of arbitrary objects, somewhat akin to an array in many other programming languages but more flexible. To define a list, you typically enclose a comma-separated sequence of objects in square brackets ([]
), as shown below:
>>> colors = ["red", "green", "blue", "yellow"]
>>> colors
['red', 'green', 'blue', 'yellow']
In this code snippet, you define a list of colors using string objects separated by commas and enclose them in square brackets.
Similarly, tuples are also collections of arbitrary objects. To define a tuple, you’ll enclose a comma-separated sequence of objects in parentheses (()
), as shown below:
>>> person = ("Jane Doe", 25, "Python Developer", "Canada")
>>> person
('Jane Doe', 25, 'Python Developer', 'Canada')
In this example, you define a tuple with data for a given person, including their name, age, job, and base country.
Up to this point, it may seem that lists and tuples are mostly the same. However, there’s an important difference:
Feature | List | Tuple |
---|---|---|
Is an ordered sequence | ✅ | ✅ |
Can contain arbitrary objects | ✅ | ✅ |
Can be indexed and sliced | ✅ | ✅ |
Can be nested | ✅ | ✅ |
Is mutable | ✅ | ❌ |
Both lists and tuples are sequence data types, which means they can contain objects arranged in order. You can access those objects using an integer index that represents their position in the sequence.
Even though both data types can contain arbitrary and heterogeneous objects, you’ll commonly use lists to store homogeneous objects and tuples to store heterogeneous objects.
Note: In this tutorial, you’ll see the terms homogeneous and heterogeneous used to express the following ideas:
- Homogeneous: Objects of the same data type or the same semantic meaning, like a series of animals, fruits, colors, and so on.
- Heterogeneous: Objects of different data types or different semantic meanings, like the attributes of a car: model, color, make, year, fuel type, and so on.
You can perform indexing and slicing operations on both lists and tuples. You can also have nested lists and nested tuples or a combination of them, like a list of tuples.
The most notable difference between lists and tuples is that lists are mutable, while tuples are immutable. This feature distinguishes them and drives their specific use cases.
Essentially, a list doesn’t have a fixed length since it’s immutable. Therefore, it’s natural to use homogeneous elements to have some structure in the list. A tuple, on the other hand, has a fixed length so the position of elements can have meaning, supporting heterogeneous data.
Creating Lists in Python
In many situations, you’ll define a list
object using a literal. A list literal is a comma-separated sequence of objects enclosed in square brackets:
>>> countries = ["United States", "Canada", "Poland", "Germany", "Austria"]
>>> countries
['United States', 'Canada', 'Poland', 'Germany', 'Austria']
In this example, you create a list of countries represented by string objects. Because lists are ordered sequences, the values retain the insertion order.
Read the full article at https://realpython.com/python-lists-tuples/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
September 04, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
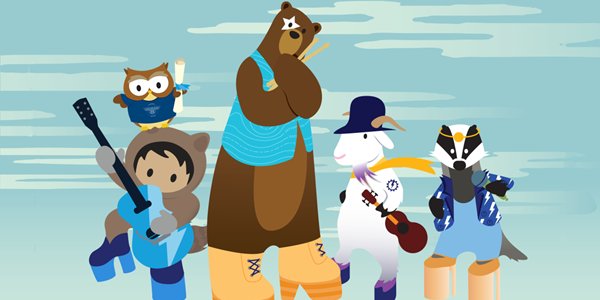
Post a Comment