Functional Programming in Python: When and How to Use It
by:
blow post content copied from Real Python
click here to view original post
Functional programming is a programming paradigm in which the primary method of computation is the evaluation of functions. But how does Python support functional programming?
In this tutorial, you’ll learn:
- What the functional programming paradigm entails
- What it means to say that functions are first-class citizens in Python
- How to define anonymous functions with the
lambda
keyword - How to implement functional code using
map()
,filter()
, andreduce()
Functional programming typically plays a minor role in Python code, but it’s still good to be familiar with it. You’ll probably encounter it from time to time when reading code written by others. And you may even find situations where it’s advantageous to use Python’s functional programming capabilities in your own code.
Get Your Code: Click here to download the free sample code that shows you when and how to use functional programming in Python.
What Is Functional Programming?
A pure function is a function whose output value follows solely from its input values without any observable side effects. In functional programming, a program consists primarily of the evaluation of pure functions. Computation proceeds by nested or composed function calls without changes to state or mutable data.
The functional paradigm is popular because it offers several advantages over other programming paradigms. Functional code is:
- High level: You describe the result you want rather than explicitly specifying the steps required to get there. Single statements tend to be concise but pack a lot of punch.
- Transparent: The behavior of a pure function can be described by its inputs and outputs, without intermediary values. This eliminates the possibility of side effects and facilitates debugging.
- Parallelizable: Routines that don’t cause side effects can more easily run in parallel with one another.
Many programming languages support some degree of functional programming. In some languages, virtually all code follows the functional paradigm. Haskell is one such example. Python, by contrast, does support functional programming but contains features of other programming models as well.
While it’s true that an in-depth description of functional programming is somewhat complex, the goal here isn’t to present a rigorous definition but to show you what you can do by way of functional programming in Python.
How Well Does Python Support Functional Programming?
To support functional programming, it’s beneficial if a function in a given programming language can do these two things:
- Take another function as an argument
- Return another function to its caller
Python plays nicely in both respects. Everything in Python is an object, and all objects in Python have more or less equal stature. Functions are no exception.
In Python, functions are first-class citizens. This means that functions have the same characteristics as values like strings and numbers. Anything you would expect to be able to do with a string or number, you can also do with a function.
For example, you can assign a function to a variable. You can then use that variable the same way you would use the function itself:
1>>> def func():
2... print("I am function func()!")
3...
4
5>>> func()
6I am function func()!
7
8>>> another_name = func
9>>> another_name()
10I am function func()!
The assignment another_name = func
on line 8 creates a new reference to func()
named another_name
. You can then call the function by either of the two names, func
or another_name
, as shown on lines 5 and 9.
You can display a function to the console with print()
, include it as an element in a composite data object like a list, or even use it as a dictionary key:
>>> def func():
... print("I am function func()!")
...
>>> print("cat", func, 42)
cat <function func at 0x7f81b4d29bf8> 42
>>> objects = ["cat", func, 42]
>>> objects[1]
<function func at 0x7f81b4d29bf8>
>>> objects[1]()
I am function func()!
>>> d = {"cat": 1, func: 2, 42: 3}
>>> d[func]
2
In this example, func()
appears in all the same contexts as the values "cat"
and 42
, and the interpreter handles it just fine.
Note: What you can or can’t do with an object in Python depends to some extent on context. Some operations work for certain object types but not for others.
For example, you can add two integer objects or concatenate two string objects with the plus operator (+
), but the plus operator isn’t defined for function objects.
For present purposes, what matters is that functions in Python satisfy the two criteria beneficial for functional programming listed above. You can pass a function to another function as an argument:
1>>> def inner():
2... print("I am function inner()!")
3...
4
5>>> def outer(function):
6... function()
7...
8
9>>> outer(inner)
10I am function inner()!
Read the full article at https://realpython.com/python-functional-programming/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
August 05, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
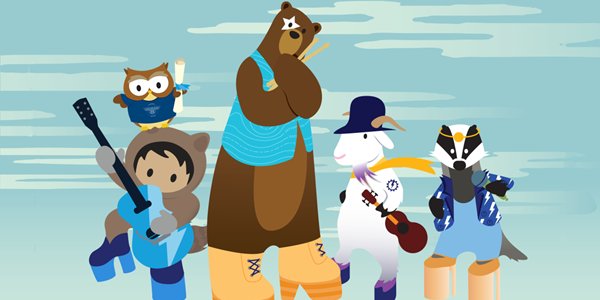
Post a Comment