Python Mappings: A Comprehensive Guide
by:
blow post content copied from Real Python
click here to view original post
One of the main data structures you learn about early in your Python learning journey is the dictionary. Dictionaries are the most common and well-known of Python’s mappings. However, there are other mappings in Python’s standard library and third-party modules. Mappings share common characteristics, and understanding these shared traits will help you use them more effectively.
In this tutorial, you’ll learn about:
- Basic characteristics of a mapping
- Operations that are common to most mappings
- Abstract base classes
Mapping
andMutableMapping
- User-defined mutable and immutable mappings and how to create them
This tutorial assumes that you’re familiar with Python’s built-in data types, especially dictionaries, and with the basics of object-oriented programming.
Get Your Code: Click here to download the free sample code that you’ll use to learn about mappings in Python.
Take the Quiz: Test your knowledge with our interactive “Python Mappings” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Python MappingsIn this quiz, you'll test your understanding of the basic characteristics and operations of Python mappings. By working through this quiz, you'll revisit the key concepts and techniques of creating a custom mapping.
Understanding the Main Characteristics of Python Mappings
A mapping is a collection that allows you to look up a key and retrieve its value. The keys in mappings can be objects of a broad range of types. However, in most mappings, there are object types that can’t be used as keys, as you’ll learn later in this tutorial.
The previous paragraph described mappings as collections. A collection is an iterable container that has a defined size. However, mappings also have additional features. You’ll explore each of these mapping characteristics with examples from Python’s main mapping types.
The feature that’s most characteristic of mappings is the ability to retrieve a value using a key. You can use a dictionary to demonstrate this operation:
>>> points = {
... "Denise": 3,
... "Igor": 2,
... "Sarah": 3,
... "Trevor": 1,
... }
>>> points["Sarah"]
3
>>> points["Matt"]
Traceback (most recent call last):
...
KeyError: 'Matt'
The dictionary points
contains four items, each with a key and a value. You can use the key within the square brackets to fetch the value associated with that key. However, if the key doesn’t exist in the dictionary, the code raises a KeyError
.
You can use one of the mappings in the standard-library collections
module to assign a default value for keys that aren’t present in the collection. The defaultdict
type includes a callable that’s called each time you try to access a key that doesn’t exist. If you want the default value to be zero, you can use a lambda
function that returns 0 as the first argument in defaultdict
:
>>> from collections import defaultdict
>>> points_default = defaultdict(
... lambda: 0,
... points,
... )
>>> points_default
defaultdict(<function <lambda> at 0x104a95da0>, {'Denise': 3,
'Igor': 2, 'Sarah': 3, 'Trevor': 1})
>>> points_default["Sarah"]
3
>>> points_default["Matt"]
0
>>> points_default
defaultdict(<function <lambda> at 0x103e6c700>, {'Denise': 3,
'Igor': 2, 'Sarah': 3, 'Trevor': 1, 'Matt': 0})
The defaultdict
constructor has two arguments in this example. The first argument is the callable that’s used when a default value is needed. The second argument is the dictionary you created earlier. You can use any valid argument when you call dict()
as the second argument in defaultdict()
or omit this argument to create an empty defaultdict
.
When you access a key that’s missing from the dictionary, the key is added, and the default value is assigned to it. You can also create the same points_default
object using the callable int
as the first argument since calling int()
with no arguments returns 0.
All mappings are also collections, which means they’re iterable containers with a defined length. You can explore these characteristics with another mapping in Python’s standard library, collections.Counter
:
>>> from collections import Counter
>>> letters = Counter("learning python")
>>> letters
Counter({'n': 3, 'l': 1, 'e': 1, 'a': 1, 'r': 1, 'i': 1, 'g': 1,
' ': 1, 'p': 1, 'y': 1, 't': 1, 'h': 1, 'o': 1})
The letters in the string "learning python"
are converted into keys in Counter
, and the number of occurrences of each letter is used as the value corresponding to each key.
You can confirm that this mapping is iterable, has a defined length, and is a container:
>>> for letter in letters:
... print(letter)
...
l
e
a
r
n
i
g
p
y
t
h
o
>>> len(letters)
13
>>> "n" in letters
True
>>> "x" in letters
False
You can use the Counter
object letters
in a for
loop, which confirms it’s iterable. All mappings are iterable. However, the iteration loops through the keys and not the values. You’ll see how to iterate through the values or through both keys and values later in this tutorial.
The built-in len()
function returns the number of items in the mapping. This is equal to the number of unique characters in the original string, including the space character. The object is sized since len()
returns a value.
You can use the in
keyword to confirm which elements are in the mapping. This check alone isn’t sufficient to confirm that the mapping is a container. However, you can also access the object’s .__contains__()
special method directly:
>>> letters.__contains__("n")
True
Read the full article at https://realpython.com/python-mappings/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
June 12, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
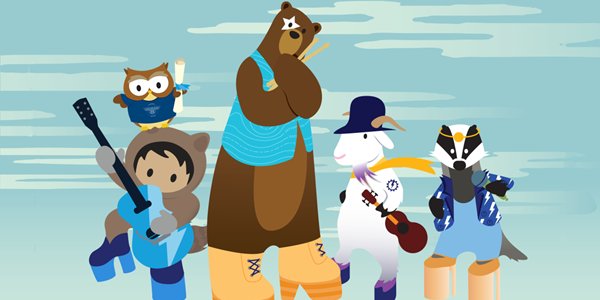
Post a Comment