Using Pandas Concat ( pd.concat ) : Hector Martinez
by: Hector Martinez
blow post content copied from PyImageSearch
click here to view original post
Overview of the Pandas Concat Function
In this tutorial, you will learn how to masterfully use pandas concat
to merge and combine large datasets with ease, boosting your data manipulation skills in Python. Whether you are new to data science or looking to refine your toolkit, understanding the pd.concat
method is crucial for efficient data handling in any project.
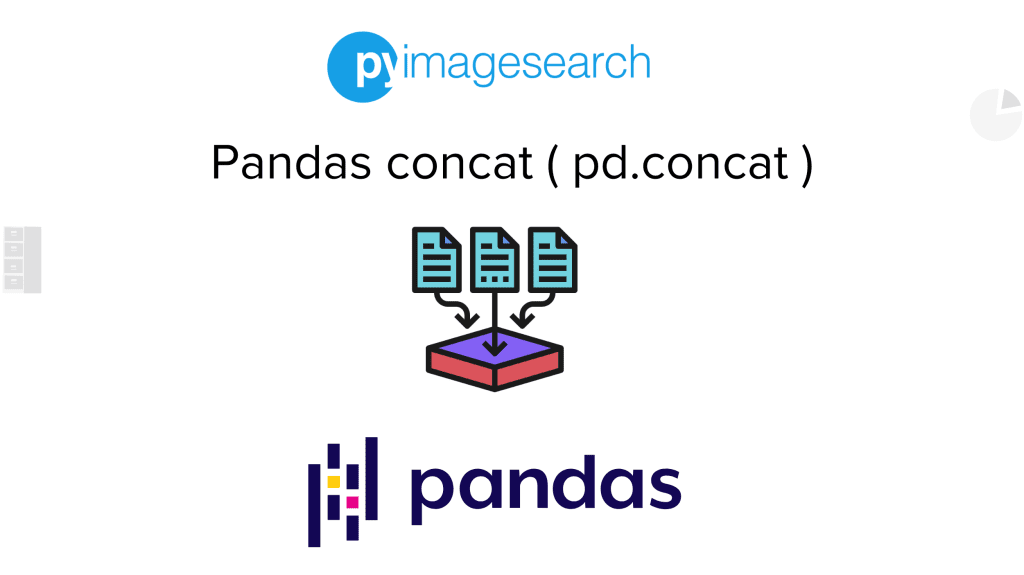
The pd.concat
function is a powerful tool within the pandas library, designed to concatenate pandas objects along a particular axis while performing optional set logic (union or intersection) of the indexes (if any) on the other axes. By the end of this guide, you’ll be able to seamlessly integrate datasets from various sources, handle different types of data alignment issues, and optimize your data analysis workflow with pandas concat
.
Next we’ll set up your development environment to ensure you have all the necessary tools installed. Following that, we’ll dive into simple examples to help you get comfortable with the basic functionalities of pandas concat
. Then, we will explore more complex scenarios to demonstrate its advanced features and versatility. Each example will be explained in detail, helping you understand not only how to implement these functions but also why they are useful in different contexts.
Stay tuned as we embark on this journey to unlock the full potential of data manipulation with pd.concat
in Python.
Things to Be Aware of When Using Pandas Concat
When using pd.concat
to combine DataFrames or Series in pandas, there are several considerations to keep in mind to ensure your data manipulation is effective and error-free:
- Handling Indexes: By default,
pd.concat
preserves the original indexes of the DataFrames or Series being concatenated. This can lead to duplicate index values, which might cause issues in subsequent data operations. You can use theignore_index=True
parameter to reset the index in the resulting DataFrame. - Column Alignment:
pd.concat
aligns data based on column labels in the different DataFrames. If some columns are not present in all DataFrames, the resulting DataFrame will have NaN values in these positions unless handled otherwise. Thejoin='outer'
parameter (which is the default) results in an outer join, andjoin='inner'
results in an inner join, which can be used to control this behavior. - Data Types: Mixing dtypes in
pandas concat
can lead to the upcasting of the entire column to a more general or compatible type. This might impact memory usage and performance. Ensuring consistent data types across DataFrames can help maintain performance. - Performance Considerations: While
pd.concat
is very efficient, concatenating a large number of objects or very large DataFrames can be memory-intensive and slow. In such cases, alternatives like Dask or incremental concatenation, where you concatenate in chunks, might be more efficient.
Being aware of these nuances will help you use pd.concat
more effectively and avoid common pitfalls that might lead to unexpected results or performance issues.
Configuring Your Development Environment
To follow this guide, you need to have the Pandas library installed on your system.
Luckily, Pandas is pip-installable:
$pip install pandas
If you need help configuring your development environment for Pandas, we highly recommend that you read our pip install Pandas guide — it will have you up and running in minutes.
Need Help Configuring Your Development Environment?
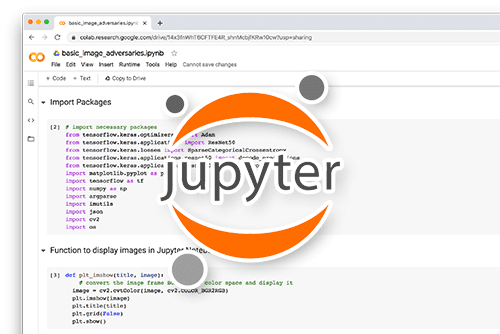
All that said, are you:
- Short on time?
- Learning on your employer’s administratively locked system?
- Wanting to skip the hassle of fighting with the command line, package managers, and virtual environments?
- Ready to run the code immediately on your Windows, macOS, or Linux system?
Then join PyImageSearch University today!
Gain access to Jupyter Notebooks for this tutorial and other PyImageSearch guides pre-configured to run on Google Colab’s ecosystem right in your web browser! No installation required.
And best of all, these Jupyter Notebooks will run on Windows, macOS, and Linux!
Project Structure
We first need to review our project directory structure.
Start by accessing this tutorial’s “Downloads” section to retrieve the source code and example images.
From there, take a look at the directory structure:
$ tree . --dirsfirst . └── pandas_melt_examples.py 0 directories, 1 files
Simple Example of Using pd.concat
To get started with pd.concat
, let’s create a simple example that demonstrates how to concatenate two small DataFrames. This will help you understand the basic functionality of concatenating datasets vertically (row-wise) and horizontally (column-wise).
# Import Pandas library import pandas as pd # Create two simple DataFrames df1 = pd.DataFrame({ 'A': ['A0', 'A1', 'A2', 'A3'], 'B': ['B0', 'B1', 'B2', 'B3'], 'C': ['C0', 'C1', 'C2', 'C3'] }) df2 = pd.DataFrame({ 'A': ['A4', 'A5', 'A6', 'A7'], 'B': ['B4', 'B5', 'B6', 'B7'], 'C': ['C4', 'C5', 'C6', 'C7'] }) # Concatenate the DataFrames vertically result_vertical = pd.concat([df1, df2], ignore_index=True) # Concatenate the DataFrames horizontally result_horizontal = pd.concat([df1, df2], axis=1) print("Vertical Concatenation:\n", result_vertical) print("Horizontal Concatenation:\n", result_horizontal)
We Start on Lines 1 and 2: First, the pandas library is imported with the alias ‘pd’. This library provides data manipulation and analysis capabilities in Python.
Lines 5-9: A DataFrame ‘df1’ is created using the pandas DataFrame function. This DataFrame consists of three columns labeled ‘A’, ‘B’, and ‘C’. Each column is populated with a list of values.
Lines 11-15: Another DataFrame ‘df2’ is created in the same way as ‘df1’. It also has three columns ‘A’, ‘B’, and ‘C’ with different values.
Line 18: The ‘pd.concat’ function is used to concatenate ‘df1’ and ‘df2’ vertically. The ‘ignore_index’ parameter is set to True, which means the original row indices from ‘df1’ and ‘df2’ are ignored and a new index is generated for the resulting DataFrame. The result is stored in the ‘result_vertical’ variable.
Line 21: The ‘pd.concat’ function is used again, but this time the ‘axis’ parameter is set to 1. This results in a horizontal concatenation of ‘df1’ and ‘df2’. The result is stored in the ‘result_horizontal’ variable.
Lines 23 and 24: The ‘print’ function is used to display the results of the vertical and horizontal concatenations.
When you run this code, you’ll see the following output:
Vertical Concatenation:
A B C 0 A0 B0 C0 1 A1 B1 C1 2 A2 B2 C2 3 A3 B3 C3 4 A4 B4 C4 5 A5 B5 C5 6 A6 B6 C6 7 A7 B7 C7
Horizontal Concatenation:
A B C A B C 0 A0 B0 C0 A4 B4 C4 1 A1 B1 C1 A5 B5 C5 2 A2 B2 C2 A6 B6 C6 3 A3 B3 C3 A7 B7 C7
This example clearly shows how pandas concat
can be used to combine DataFrames along different axes, providing flexibility in how you merge data. Next, we’ll create a more complex example that demonstrates the function’s utility with different variables.
Complex Example of Using Pandas Concat
In this more advanced example, we will explore how pd.concat
can handle different types of data alignment and manage missing values when concatenating DataFrames that don’t perfectly align. This demonstrates the flexibility and power of pd.concat
in more realistic data scenarios where discrepancies in data structure often occur.
# Create two DataFrames with different columns and missing values df3 = pd.DataFrame({ 'A': ['A8', 'A9', 'A10', 'A11'], 'B': ['B8', 'B9', 'B10', 'B11'], 'C': ['C8', 'C9', 'C10', 'C11'] }) df4 = pd.DataFrame({ 'A': ['A12', 'A13', 'A14', 'A15'], 'C': ['C12', 'C13', 'C14', 'C15'], 'D': ['D12', 'D13', 'D14', 'D15'] # Note the new column 'D' }) # Concatenate the DataFrames with different columns result_with_diff_columns = pd.concat([df3, df4], sort=False) print("Concatenation with Different Columns and Missing Values:\n", result_with_diff_columns)
This explanation will detail the code involving the creation and concatenation of two pandas DataFrames with different columns and missing values.
In Lines 1-6, a DataFrame named `df3` is created using the `pd.DataFrame()` function. This DataFrame consists of three columns (‘A’, ‘B’, ‘C’) each containing four string values (‘A8’ to ‘A11’ for column ‘A’, ‘B8’ to ‘B11’ for column ‘B’, and ‘C8’ to ‘C11’ for column ‘C’).
In Lines 8-12, a second DataFrame named `df4` is created. This DataFrame also consists of three columns, but they are ‘A’, ‘C’, and ‘D’. The column ‘B’ from `df3` is missing and a new column ‘D’ is added. The values for these columns range from ‘A12’ to ‘A15’ for column ‘A’, ‘C12’ to ‘C15’ for column ‘C’, and ‘D12’ to ‘D15’ for column ‘D’.
On Line 15, the `pd.concat()` function is used to concatenate `df3` and `df4`. The `sort=False` parameter is used to keep the original order of columns in the new DataFrame. Because `df3` and `df4` don’t have the exact same set of columns, the resulting DataFrame will have missing values (denoted as `NaN` in pandas).
Finally, Line 17 prints the concatenated DataFrame. The resulting DataFrame has four columns (‘A’, ‘B’, ‘C’, ‘D’) and eight rows. The rows originating from `df4` will have `NaN` in the ‘B’ column (because `df4` didn’t have a ‘B’ column), and the rows originating from `df3` will have `NaN` in the ‘D’ column (because `df3` didn’t have a ‘D’ column).
When you run this code, you’ll observe the following output:
Concatenation with Different Columns and Missing Values:
A B C D 0 A8 B8 C8 NaN 1 A9 B9 C9 NaN 2 A10 B10 C10 NaN 3 A11 B11 C11 NaN 4 A12 NaN C12 D12 5 A13 NaN C13 D13 6 A14 NaN C14 D14 7 A15 NaN C15 D15
This output illustrates how pandas concat
deals with columns that do not match across DataFrames. It fills in missing values with NaN
where data from a non-existent column in one of the DataFrames is expected, allowing for a flexible integration of datasets with varying structures.
Exploring Alternatives to pd.concat
While pd.concat
is highly effective for many data manipulation tasks, there are alternatives that may provide better performance or additional features, especially in the context of large datasets or parallel computing. One such alternative is the Dask library, which is particularly suited for big data applications and can work in parallel on large datasets that do not fit into memory.
Dask: Scalable Analytics in Python
Dask is a flexible parallel computing library for analytics that integrates seamlessly with existing Python libraries like Pandas. Unlike pandas, which operates in-memory, Dask can work with data that exceeds the memory capacity of your system, processing large datasets in chunks across multiple cores or even different machines.
Simple Example Using Dask
Here’s how you can use Dask to achieve similar functionality to pd.concat
but with the capability to handle larger datasets more efficiently:
import dask.dataframe as dd # Create two Dask DataFrames (simulating large datasets) ddf1 = dd.from_pandas(df1, npartitions=2) ddf2 = dd.from_pandas(df2, npartitions=2) # Concatenate the Dask DataFrames vertically result_dask = dd.concat([ddf1, ddf2]) # Compute result to bring it into memory (this executes the actual computation) computed_result = result_dask.compute() print(computed_result)
Why Dask is a Better Approach for Large Datasets
- Scalability: Dask can scale up to clusters of machines and handle computations on datasets that are much larger than the available memory, whereas pandas is limited by the size of the machine’s RAM.
- Lazy Evaluation: Dask operations are lazy, meaning they build a task graph and execute it only when you explicitly compute the results. This allows Dask to optimize the operations and manage resources more efficiently.
- Parallel Computing: Dask can automatically divide data and computation over multiple cores or different machines, providing significant speed-ups especially for large-scale data.
This makes Dask an excellent alternative to pd.concat
when working with very large data sets or in distributed computing environments where parallel processing can significantly speed up data manipulations.
What's next? We recommend PyImageSearch University.
84 total classes • 114+ hours of on-demand code walkthrough videos • Last updated: February 2024
★★★★★ 4.84 (128 Ratings) • 16,000+ Students Enrolled
I strongly believe that if you had the right teacher you could master computer vision and deep learning.
Do you think learning computer vision and deep learning has to be time-consuming, overwhelming, and complicated? Or has to involve complex mathematics and equations? Or requires a degree in computer science?
That’s not the case.
All you need to master computer vision and deep learning is for someone to explain things to you in simple, intuitive terms. And that’s exactly what I do. My mission is to change education and how complex Artificial Intelligence topics are taught.
If you're serious about learning computer vision, your next stop should be PyImageSearch University, the most comprehensive computer vision, deep learning, and OpenCV course online today. Here you’ll learn how to successfully and confidently apply computer vision to your work, research, and projects. Join me in computer vision mastery.
Inside PyImageSearch University you'll find:
- ✓ 84 courses on essential computer vision, deep learning, and OpenCV topics
- ✓ 84 Certificates of Completion
- ✓ 114+ hours of on-demand video
- ✓ Brand new courses released regularly, ensuring you can keep up with state-of-the-art techniques
- ✓ Pre-configured Jupyter Notebooks in Google Colab
- ✓ Run all code examples in your web browser — works on Windows, macOS, and Linux (no dev environment configuration required!)
- ✓ Access to centralized code repos for all 536+ tutorials on PyImageSearch
- ✓ Easy one-click downloads for code, datasets, pre-trained models, etc.
- ✓ Access on mobile, laptop, desktop, etc.
Summary of pd.concat Tutorial
In this comprehensive tutorial, you have learned how to use the pandas concat
function to merge and combine data efficiently in Python. We started with a basic introduction to pd.concat
, exploring its fundamental capabilities to concatenate pandas objects along a particular axis. This included simple examples of vertical and horizontal concatenations, which demonstrated how to combine DataFrame objects row-wise and column-wise.
We then advanced to more complex scenarios, addressing data alignment and managing missing values when DataFrames with different structures are concatenated. These examples showcased the robustness of pandas concat
in handling datasets that do not perfectly align, illustrating its practical utility in real-world data manipulation tasks.
Moreover, we explored Dask as a powerful alternative to pandas for handling large datasets. Dask extends the capabilities of pandas by enabling parallel computation on larger-than-memory data, making it suitable for big data applications that require scalability and efficiency.
I hope you found this tutorial informative and engaging, providing you with valuable skills that you can apply to your data analysis projects.
To download the source code to this post (and be notified when future tutorials are published here on PyImageSearch), simply enter your email address in the form below!
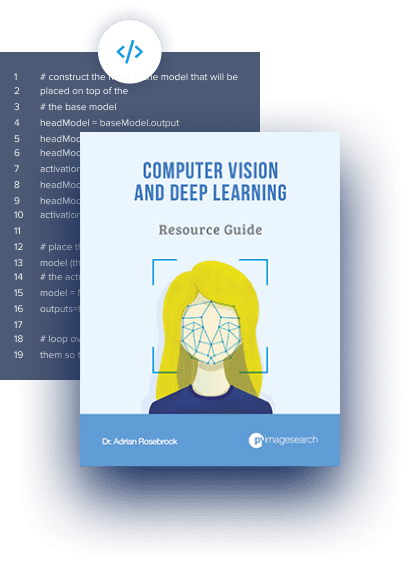
Download the Source Code and FREE 17-page Resource Guide
Enter your email address below to get a .zip of the code and a FREE 17-page Resource Guide on Computer Vision, OpenCV, and Deep Learning. Inside you'll find my hand-picked tutorials, books, courses, and libraries to help you master CV and DL!
The post Using Pandas Concat ( pd.concat ) appeared first on PyImageSearch.
May 08, 2024 at 03:07AM
Click here for more details...
=============================
The original post is available in PyImageSearch by Hector Martinez
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
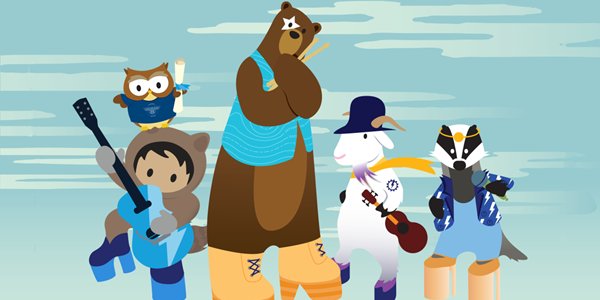
Post a Comment