Python's Built-in Exceptions: A Walkthrough With Examples
by:
blow post content copied from Real Python
click here to view original post
Python has a complete set of built-in exceptions that provide a quick and efficient way to handle errors and exceptional situations that may happen in your code. Knowing the most commonly used built-in exceptions is key for you as a Python developer. This knowledge will help you debug code because each exception has a specific meaning that can shed light on your debugging process.
You’ll also be able to handle and raise most of the built-in exceptions in your Python code, which is a great way to deal with errors and exceptional situations without having to create your own custom exceptions.
In this tutorial, you’ll:
- Learn what errors and exceptions are in Python
- Understand how Python organizes the built-in exceptions in a class hierarchy
- Explore the most commonly used built-in exceptions
- Learn how to handle and raise built-in exceptions in your code
To smoothly walk through this tutorial, you should be familiar with some core concepts in Python. These concepts include Python classes, class hierarchies, exceptions, try
… except
blocks, and the raise
statement.
Get Your Code: Click here to download the free sample code that you’ll use to learn about Python’s built-in exceptions.
Errors and Exceptions in Python
Errors and exceptions are important concepts in programming, and you’ll probably spend a considerable amount of time dealing with them in your programming career. Errors are concrete conditions, such as syntax and logical errors, that make your code work incorrectly or even crash.
Often, you can fix errors by updating or modifying the code, installing a new version of a dependency, checking the code’s logic, and so on.
For example, say you need to make sure that a given string has a certain number of characters. In this case, you can use the built-in len()
function:
>>> len("Pythonista") = 10
File "<input>", line 1
...
SyntaxError: cannot assign to function call here.
Maybe you meant '==' instead of '='?
In this example, you use the wrong operator. Instead of using the equality comparison operator, you use the assignment operator. This code raises a SyntaxError
, which represents a syntax error as its name describes.
Note: In the above code, you’ll note how nicely the error message suggests a possible solution for correcting the code. Starting in version 3.10, the Python core developers have put a lot of effort into improving the error messages to make them more friendly and useful for debugging.
To fix the error, you need to localize the affected code and correct the syntax. This action will remove the error:
>>> len("Pythonista") == 10
True
Now the code works correctly, and the SyntaxError
is gone. So, your code won’t break, and your program will continue its normal execution.
There’s something to learn from the above example. You can fix errors, but you can’t handle them. In other words, if you have a syntax error like the one in the example, then you won’t be able to handle that error and make the code run. You need to correct the syntax.
On the other hand, exceptions are events that interrupt the execution of a program. As their name suggests, exceptions occur in exceptional situations that should or shouldn’t happen. So, to prevent your program from crashing after an exception, you must handle the exception with the appropriate exception-handling mechanism.
To better understand exceptions, say that you have a Python expression like a + b
. This expression will work if a
and b
are both strings or numbers:
>>> a = 4
>>> b = 3
>>> a + b
7
In this example, the code works correctly because a
and b
are both numbers. However, the expression raises an exception if a
and b
are of types that can’t be added together:
>>> a = "4"
>>> b = 3
>>> a + b
Traceback (most recent call last):
File "<input>", line 1, in <module>
a + b
~~^~~
TypeError: can only concatenate str (not "int") to str
Because a
is a string and b
is a number, your code fails with a TypeError
exception. Since there is no way to add text and numbers, your code has faced an exceptional situation.
Python uses classes to represent exceptions and errors. These classes are generically known as exceptions, regardless of what a concrete class represents, an exception or an error. Exception classes give us information about an exceptional situation and also errors detected during the program’s execution.
The first example in this section shows a syntax error in action. The SyntaxError
class represents an error but it’s implemented as a Python exception. This could be confusing, but Python uses exception classes for both errors and exceptions.
Read the full article at https://realpython.com/python-built-in-exceptions/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
May 15, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
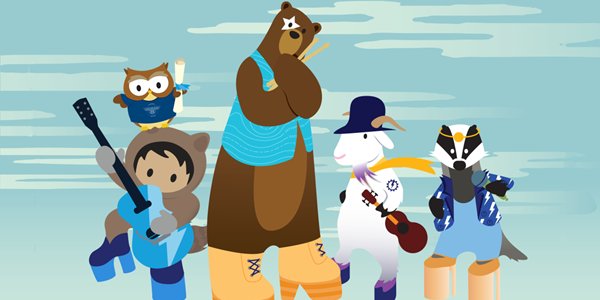
Post a Comment