Python Sequences: A Comprehensive Guide :
by:
blow post content copied from Real Python
click here to view original post
A phrase you’ll often hear is that everything in Python is an object, and every object has a type. This points to the importance of data types in Python. However, often what an object can do is more important than what it is. So, it’s useful to discuss categories of data types and one of the main categories is Python’s sequence.
In this tutorial, you’ll learn about:
- Basic characteristics of a sequence
- Operations that are common to most sequences
- Special methods associated with sequences
- Abstract base classes
Sequence
andMutableSequence
- User-defined mutable and immutable sequences and how to create them
This tutorial assumes that you’re familiar with Python’s built-in data types and with the basics of object-oriented programming.
Get Your Code: Click here to download the free sample code that you’ll use to learn about Python sequences in this comprehensive guide.
Take the Quiz: Test your knowledge with our interactive “Python Sequences: A Comprehensive Guide” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Python Sequences: A Comprehensive GuideIn this quiz, you'll test your understanding of sequences in Python. You'll revisit the basic characteristics of a sequence, operations common to most sequences, special methods associated with sequences, and how to create user-defined mutable and immutable sequences.
Building Blocks of Python Sequences
It’s likely you used a Python sequence the last time you wrote Python code, even if you don’t know it. The term sequence doesn’t refer to a specific data type but to a category of data types that share common characteristics.
Characteristics of Python Sequences
A sequence is a data structure that contains items arranged in order, and you can access each item using an integer index that represents its position in the sequence. You can always find the length of a sequence. Here are some examples of sequences from Python’s basic built-in data types:
>>> # List
>>> countries = ["USA", "Canada", "UK", "Norway", "Malta", "India"]
>>> for country in countries:
... print(country)
...
USA
Canada
UK
Norway
Malta
India
>>> len(countries)
6
>>> countries[0]
'USA'
>>> # Tuple
>>> countries = "USA", "Canada", "UK", "Norway", "Malta", "India"
>>> for country in countries:
... print(country)
...
USA
Canada
UK
Norway
Malta
India
>>> len(countries)
6
>>> countries[0]
'USA'
>>> # Strings
>>> country = "India"
>>> for letter in country:
... print(letter)
...
I
n
d
i
a
>>> len(country)
5
>>> country[0]
'I'
Lists, tuples, and strings are among Python’s most basic data types. Even though they’re different types with distinct characteristics, they have some common traits. You can summarize the characteristics that define a Python sequence as follows:
- A sequence is an iterable, which means you can iterate through it.
- A sequence has a length, which means you can pass it to
len()
to get its number of elements. - An element of a sequence can be accessed based on its position in the sequence using an integer index. You can use the square bracket notation to index a sequence.
There are other built-in data types in Python that also have all of these characteristics. One of these is the range
object:
>>> numbers = range(5, 11)
>>> type(numbers)
<class 'range'>
>>> len(numbers)
6
>>> numbers[0]
5
>>> numbers[-1]
10
>>> for number in numbers:
... print(number)
...
5
6
7
8
9
10
You can iterate through a range
object, which makes it iterable. You can also find its length using len()
and fetch items through indexing. Therefore, a range
object is also a sequence.
You can also verify that bytes
and bytearray
objects, two of Python’s built-in data structures, are also sequences. Both are sequences of integers. A bytes
sequence is immutable, while a bytearray
is mutable.
Special Methods Associated With Python Sequences
In Python, the key characteristics of a data type are determined using special methods, which are defined in the class definitions. The special methods associated with the properties of sequences are the following:
.__iter__()
: This special method makes an object iterable using Python’s preferred iteration protocol. However, it’s possible for a class without an.__iter__()
special method to create iterable objects if the class has a.__getitem__()
special method that supports iteration. Most sequences have an.__iter__()
special method, but it’s possible to have a sequence without this method..__len__()
: This special method defines the length of an object, which is normally the number of elements contained within it. Thelen()
built-in function calls an object’s.__len__()
special method. Every sequence has this special method..__getitem__()
: This special method enables you to access an item from a sequence. The square brackets notation can be used to fetch an item. The expressioncountries[0]
is equivalent tocountries.__getitem__(0)
. For sequences,.__getitem__()
should accept integer arguments starting from zero. Every sequence has this special method. This method can also ensure an object is iterable if the.__iter__()
special method is missing.
Therefore, all sequences have a .__len__()
and a .__getitem__()
special method and most also have .__iter__()
.
However, it’s not sufficient for an object to have these special methods to be a sequence. For example, many mappings also have these three methods but mappings aren’t sequences.
A dictionary is an example of a mapping. You can find the length of a dictionary and iterate through its keys using a for
loop or other iteration techniques. You can also fetch an item from a dictionary using the square brackets notation.
This characteristic is defined by .__getitem__()
. However, .__getitem__()
needs arguments that are dictionary keys and returns their matching values. You can’t index a dictionary using integers that refer to an item’s position in the dictionary. Therefore, dictionaries are not sequences.
Slicing in Python Sequences
Read the full article at https://realpython.com/python-sequences/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
May 01, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
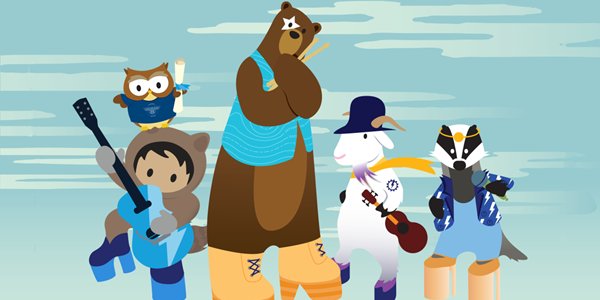
Post a Comment