Python's unittest: Writing Unit Tests for Your Code
by:
blow post content copied from Real Python
click here to view original post
The Python standard library ships with a testing framework named unittest
, which you can use to write automated tests for your code. The unittest
package has an object-oriented approach where test cases derive from a base class, which has several useful methods.
The framework supports many features that will help you write consistent unit tests for your code. These features include test cases, fixtures, test suites, and test discovery capabilities.
In this tutorial, you’ll learn how to:
- Write
unittest
tests with theTestCase
class - Explore the assert methods that
TestCase
provides - Use
unittest
from the command line - Group test cases using the
TestSuite
class - Create fixtures to handle setup and teardown logic
To get the most out of this tutorial, you should be familiar with some important Python concepts, such as object-oriented programming, inheritance, and assertions. Having a good understanding of code testing is a plus.
Free Bonus: Click here to download the free sample code that shows you how to use Python’s unittest to write tests for your code.
Take the Quiz: Test your knowledge with our interactive “Python's unittest: Writing Unit Tests for Your Code” quiz. You’ll receive a score upon completion to help you track your learning progress:
Interactive Quiz
Python's unittest: Writing Unit Tests for Your CodeIn this quiz, you'll test your understanding of Python testing with the unittest framework from the standard library. With this knowledge, you'll be able to create basic tests, execute them, and find bugs before your users do.
Testing Your Python Code
Code testing or software testing is a fundamental part of a modern software development cycle. Through code testing, you can verify that a given software project works as expected and fulfills its requirements. Testing enforces code quality and robustness.
You’ll do code testing during the development stage of an application or project. You’ll write tests that isolate sections of your code and verify its correctness. A well-written battery or suite of tests can also serve as documentation for the project at hand.
You’ll find several different concepts and techniques around testing. Most of them surpass the scope of this tutorial. However, unit test is an important and relevant concept. A unit test is a test that operates on an individual unit of software. A unit test aims to validate that the tested unit works as designed.
A unit is often a small part of a program that takes a few inputs and produces an output. Functions, methods, and other callables are good examples of units that you’d need to test.
In Python, there are several tools to help you write, organize, run, and automate your unit test. In the Python standard library, you’ll find two of these tools:
doctest
unittest
Python’s doctest
module is a lightweight testing framework that provides quick and straightforward test automation. It can read the test cases from your project’s documentation and your code’s docstrings. This framework is shipped with the Python interpreter as part of the batteries-included philosophy.
Note: To dive deeper into doctest
, check out the Python’s doctest: Document and Test Your Code at Once tutorial.
The unittest
package is also a testing framework. However, it provides a more complete solution than doctest
. In the following sections, you’ll learn and work with unittest
to create suitable unit tests for your Python code.
Getting to Know Python’s unittest
The unittest
package provides a unit test framework inspired by JUnit, which is a unit test framework for the Java language. The unittest
framework is directly available in the standard library, so you don’t have to install anything to use this tool.
The framework uses an object-oriented approach and supports some essential concepts that facilitate test creation, organization, preparation, and automation:
- Test case: An individual unit of testing. It examines the output for a given input set.
- Test suite: A collection of test cases, test suites, or both. They’re grouped and executed as a whole.
- Test fixture: A group of actions required to set up an environment for testing. It also includes the teardown processes after the tests run.
- Test runner: A component that handles the execution of tests and communicates the results to the user.
In the following sections, you’ll dive into using the unittest
package to create test cases, suites of tests, fixtures, and, of course, run your tests.
Organizing Your Tests With the TestCase
Class
The unittest
package defines the TestCase
class, which is primarily designed for writing unit tests. To start writing your test cases, you just need to import the class and subclass it. Then, you’ll add methods whose names should begin with test
. These methods will test a given unit of code using different inputs and check for the expected results.
Here’s a quick test case that tests the built-in abs()
function:
Read the full article at https://realpython.com/python-unittest/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
April 29, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
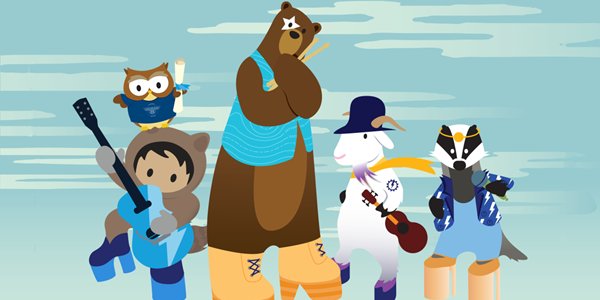
Post a Comment