Python Delete File (Ultimate Guide) : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Python offers several powerful options to handle file operations, including deleting files. Whether you need to check if a file exists before deleting, use patterns to delete multiple files, or automatically delete files under certain conditions, Python has a tool for you.
Let’s delve into various ways you can delete files using Python, ranging from straightforward to more complex scenarios.
Python Delete File if Exists
In Python, it’s good practice to check if a file exists before attempting to delete it to avoid errors. You can achieve this using the os
module.
import os file_path = 'example.txt' if os.path.exists(file_path): os.remove(file_path) print(f"File {file_path} has been deleted.") else: print(f"File {file_path} does not exist.")
In this code, we first import the os
module. We then specify the file path and check if the file exists. If it does, we use os.remove()
to delete it and confirm the deletion. If not, we output a message stating the file does not exist.
Python Delete File Pathlib
Pathlib
is a modern file handling library in Python. It provides an object-oriented approach to manage file systems and works very intuitively.
from pathlib import Path file_path = Path('example.txt') if file_path.exists(): file_path.unlink() print(f"File {file_path} has been deleted.") else: print(f"File {file_path} does not exist.")
Here, we use Path
from the pathlib
module to create a Path
object. We check if the file exists with .exists()
, and then use .unlink()
to delete it, providing an elegant, readable approach to file deletion.
Python Delete File Contents
If your goal is to clear the contents of a file without deleting the file itself, you can simply open the file in write mode.
file_path = 'example.txt' with open(file_path, 'w'): print(f"Contents of {file_path} have been cleared.")
Opening the file in ‘write’ mode ('w'
) without writing anything effectively erases the file’s contents, giving you a blank file.
Python Delete File OS
Using the os
module is one of the most common ways to delete a file in Python. It’s straightforward and explicit.
import os file_path = 'example.txt' try: os.remove(file_path) print(f"File {file_path} successfully deleted.") except FileNotFoundError: print(f"The file {file_path} does not exist.")
This approach attempts to delete the file and handles the potential FileNotFoundError
to avoid crashes if the file doesn’t exist.
Python Delete File with Wildcard
To delete multiple files that match a pattern, we can combine glob
from the glob
module with os.remove
.
import os import glob for file_path in glob.glob('*.txt'): os.remove(file_path) print(f"Deleted {file_path}") print("All .txt files deleted.")
This code snippet finds all .txt
files in the current directory and deletes each, providing an efficient way to handle multiple files.
Python Delete File After Reading
If you need to delete a file right after processing its contents, this can be managed smoothly using Python.
file_path = 'example.txt' with open(file_path, 'r') as file: data = file.read() print(f"Read data: {data}") os.remove(file_path) print(f"File {file_path} deleted after reading.")
We read the file, process the contents, and immediately delete the file afterwards. This is particularly useful for temporary files.
Python Delete File From Directory if Exists
Sometimes, we need to target a file inside a specific directory. Checking if the file exists within the directory before deletion can prevent errors.
import os directory = 'my_directory' file_name = 'example.txt' file_path = os.path.join(directory, file_name) if os.path.exists(file_path): os.remove(file_path) print(f"File {file_path} from directory {directory} has been deleted.") else: print(f"File {file_path} does not exist in {directory}.")
This code constructs the file path, checks its existence, and deletes it if present, all while handling directories.
Python Delete File Extension
Deleting files by extension in a directory is straightforward with Python. Here’s how you might delete all .log
files in a directory:
import os directory = '/path_to_directory' for file_name in os.listdir(directory): if file_name.endswith('.log'): os.remove(os.path.join(directory, file_name)) print(f"Deleted {file_name}")
This loops through all files in the given directory, checks if they end with .log
, and deletes them.
Python Delete File on Exit
To ensure files are deleted when a Python script is done executing, use atexit
.
import atexit import os file_path = 'temporary_file.txt' open(file_path, 'a').close() # Create the file def cleanup(): os.remove(file_path) print(f"File {file_path} has been deleted on exit.") atexit.register(cleanup)
This code sets up a cleanup function that deletes a specified file and registers this function to be called when the script exits.
Python Delete File After Time
For deleting files after a certain period, use time
and os
.
import os import time file_path = 'old_file.txt' time_to_wait = 10 # seconds time.sleep(time_to_wait) os.remove(file_path) print(f"File {file_path} deleted after {time_to_wait} seconds.")
After waiting for a specified time, the file is deleted, which can be useful for timed file cleanup tasks.
Summary
- Delete if Exists: Use
os.path.exists()
andos.remove()
. - Pathlib: Employ
Path.exists()
andPath.unlink()
. - Delete Contents: Open in ‘write’ mode.
- OS Module: Directly use
os.remove()
. - Wildcard Deletion: Utilize
glob.glob()
andos.remove()
. - After Reading: Delete following file read operations.
- From Directory if Exists: Construct path with
os.path.join()
. - Delete File Extension: Loop through directory and delete based on extension.
- On Exit: Use
atexit.register()
. - After Time: Combine
time.sleep()
withos.remove()
.
Each method provides a robust way to handle different file deletion scenarios efficiently and securely in Python. Choose the one that best suits your needs to maintain sleek and effective code.
April 14, 2024 at 03:23PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
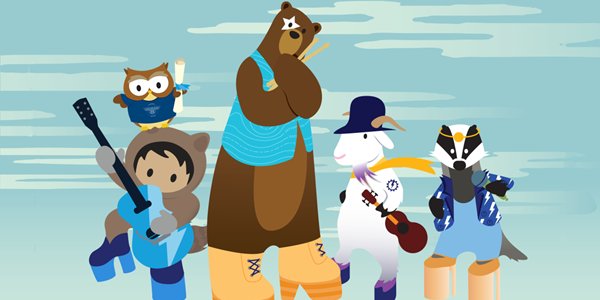
Post a Comment