How to Watermark a Graph with Matplotlib : Mike
by: Mike
blow post content copied from Mouse Vs Python
click here to view original post
Matplotlib is one of the most popular data visualization packages for the Python programming language. It allows you to create many different charts and graphs. This tutorial focuses on adding a “watermark” to your graph. If you need to learn the basics, you might want to check out Matplotlib—An Intro to Creating Graphs with Python.
Let’s get started!
Installing Matplotlib
If you don’t have Matplotlib on your computer, you must install it. Fortunately, you can use pip, the Python package manager utility that comes with Python.
Open up your terminal or command prompt and run the following command:
python -m pip install matplotlib
Pip will now install Matplotlib and any dependencies that Matplotlib needs to work properly. Assuming that Matplotlib installs successfully, you are good to go!
Watermarking Your Graph
Adding a watermark to a graph is a fun way to learn how to use Matplotlib. For this example, you will create a simple bar chart and then add some text. The text will be added at an angle across the graph as a watermark.
Open up your favorite Python IDE or text editor and create a new Python file. Then add the following code:
import matplotlib.pyplot as plt def bar_chart(numbers, labels, pos): fig = plt.figure(figsize=(5, 8)) plt.bar(pos, numbers, color="red") # add a watermark fig.text(1, 0.15, "Mouse vs Python", fontsize=45, color="blue", ha="right", va="bottom", alpha=0.4, rotation=25) plt.xticks(ticks=pos, labels=labels) plt.show() if __name__ == "__main__": numbers = [2, 1, 4, 6] labels = ["Electric", "Solar", "Diesel", "Unleaded"] pos = list(range(4)) bar_chart(numbers, labels, pos)
Your bar_chart() function takes in some numbers, labels and a list of positions for where the bars should be placed. You then create a figure to put your plot into. Then you create the bar chart using the list of bar positions and the numbers. You also tell the chart that you want the bars to be colored “red”.
The next step is to add a watermark. To do that, you call fig.text()
which lets you add text on top of your plot. Here is a quick listing of the arguments that you need to pass in:
- x, y (the first two arguments are the x/y coordinates for the text)
- fontsize – The size of the font
- color – The color of the text
- ha – Horizontal alignment
- va – Vertical alignment
- alpha – How transparent the text should be
- rotation – How many degrees to rotate the text
The last bit of code in bar_chart() adds the ticks and labels to the bottom of the plot.
When you run this code, you will see something like this:
Isn’t that neat? You now have a simple plot, and you know how to add semi-transparent text to it, too!
Wrapping Up
Proper attribution is important in academics and business. Knowing how to add a watermark to your data visualization can help you do that. You now have that knowledge when using Matplotlib.
The Matplotlib package can do many other types of plots and provides much more customization than what it covered here. Check out its documentation to learn more!
The post How to Watermark a Graph with Matplotlib appeared first on Mouse Vs Python.
April 30, 2024 at 08:31PM
Click here for more details...
=============================
The original post is available in Mouse Vs Python by Mike
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
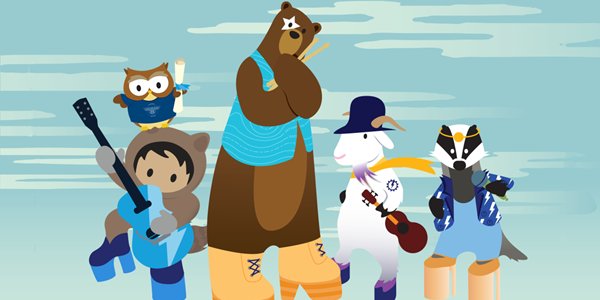
Post a Comment